编写一个shell脚本,显示当天日期,查找 xiyangyang用户是否在系统中。如果在系统中,发 一个问候“hello”给他。
时间: 2024-10-22 17:25:34 浏览: 24
为了编写一个简单的shell脚本来完成这个任务,我们可以使用`who`命令检查当前是否有名为"xiyangyang"的用户,并使用`echo`发送一条消息。这里是一个基本的bash脚本示例:
```bash
#!/bin/bash
# 显示当前日期
current_date=$(date)
echo "今日日期是: $current_date"
# 检查用户是否存在
if who | grep -q 'xiyangyang'; then
# 如果用户存在,发送问候
echo "Hello, xiyangyang!"
else
echo "Sorry, xiyangyang 用户目前不在系统中."
fi
```
要运行此脚本,需要将内容保存到一个文件(例如`greet_xiyangyang.sh`),然后给予它执行权限:
```sh
chmod +x greet_xiyangyang.sh
./greet_xiyangyang.sh
```
请注意,这个脚本假设`who`命令能提供所需信息,但在某些系统上,`who`可能会有其他限制或者返回结果格式不同。此外,如果"xiyangyang"用户登录后立即运行此脚本,可能会找不到他,因为`who`命令通常显示的是实时在线用户。
相关问题
1.下列代码的运行结果是? HashMap map = new HashMap(); map.put(“name”,null); map.put(“name”,”xiyangyang”); System.out.println(map.get(“name”));
运行结果是 "xiyangyang"。
HashMap 允许 key 为 null,所以第一次 put 操作将 null 作为 key 存入 map 中。第二次 put 操作将 "name" 作为 key 存入 map 中,对应的 value 为 "xiyangyang"。由于 key 不能重复,第二次 put 操作会覆盖第一次的操作。最后,get 方法根据 key "name" 获取对应的 value,即 "xiyangyang"。
用Python画一个喜羊羊的代码
### 回答1:
我们可以使用 Python 的第三方库 `turtle` 来画一个喜羊羊。首先,你需要安装 `turtle` 库:
```
pip install turtle
```
然后,你可以使用以下代码来画一个喜羊羊:
```
import turtle
t = turtle.Turtle()
# 画头
t.circle(50)
# 画身体
t.penup()
t.goto(0, -50)
t.pendown()
t.circle(100, 180)
# 画腿
t.penup()
t.goto(-50, -200)
t.pendown()
t.circle(50, 180)
t.penup()
t.goto(50, -200)
t.pendown()
t.circle(50, 180)
# 画眼睛
t.penup()
t.goto(-20, 50)
t.pendown()
t.circle(10)
t.penup()
t.goto(20, 50)
t.pendown()
t.circle(10)
# 画嘴巴
t.penup()
t.goto(-20, 20)
t.pendown()
t.goto(20, 20)
t.penup()
t.goto(0, 20)
t.pendown()
t.goto(0, 10)
# 隐藏画笔
t.penup()
# 让窗口等待关闭
turtle.exitonclick()
```
运行这段代码,你就可以看到一个画有喜羊羊的窗口弹出来了!
### 回答2:
要用Python画一个喜羊羊,我们可以使用turtle模块来实现图形绘制。下面是一个简单的代码示例:
```python
import turtle
def draw_xiyangyang():
# 设置画笔的颜色和尺寸
turtle.pensize(3)
turtle.pencolor("black")
# 画出喜羊羊的头部
turtle.circle(100)
turtle.fillcolor("white")
turtle.begin_fill()
turtle.circle(100)
turtle.end_fill()
# 画出喜羊羊的眼睛
turtle.penup()
turtle.goto(-40, 80)
turtle.pendown()
turtle.fillcolor("black")
turtle.begin_fill()
turtle.circle(20)
turtle.end_fill()
turtle.penup()
turtle.goto(40, 80)
turtle.pendown()
turtle.begin_fill()
turtle.circle(20)
turtle.end_fill()
# 画出喜羊羊的嘴巴
turtle.penup()
turtle.goto(-40, 50)
turtle.pendown()
turtle.pencolor("black")
turtle.seth(-60)
turtle.circle(40, 120)
turtle.penup()
# 画出喜羊羊的身体
turtle.goto(-70, -50)
turtle.pendown()
turtle.fillcolor("white")
turtle.begin_fill()
turtle.circle(70)
turtle.end_fill()
# 画出喜羊羊的脚
turtle.penup()
turtle.goto(0, -70)
turtle.pendown()
turtle.fillcolor("white")
turtle.begin_fill()
turtle.circle(15)
turtle.end_fill()
turtle.penup()
turtle.goto(-35, -70)
turtle.pendown()
turtle.fillcolor("white")
turtle.begin_fill()
turtle.circle(15)
turtle.end_fill()
# 画出喜羊羊的尾巴
turtle.penup()
turtle.goto(20, -120)
turtle.pendown()
turtle.pensize(6)
turtle.pencolor("black")
turtle.seth(-20)
turtle.circle(70, 120)
# 隐藏画笔和展示绘制结果
turtle.hideturtle()
turtle.done()
# 调用函数进行绘制
draw_xiyangyang()
```
运行上述代码后,可以看到一个用Python绘制的喜羊羊形象。注意,这只是一个简单的示例,你可以根据自己的喜好和需求进行自定义修改。
### 回答3:
使用Python绘制喜羊羊的代码可以使用Python的绘图库matplotlib来实现。
首先,需要导入matplotlib库和绘图所需的其他模块:
```python
import matplotlib.pyplot as plt
import numpy as np
```
接下来,创建一个画布和一个子图:
```python
fig, ax = plt.subplots()
```
然后,使用matplotlib的绘图函数来绘制喜羊羊的身体和脸部:
```python
# 绘制身体
body = plt.Rectangle((0, 0), 10, 12, fc='white')
ax.add_patch(body)
# 绘制脸部
face = plt.Circle((5, 10), 4, fc='white')
ax.add_patch(face)
```
再通过绘制多边形来绘制喜羊羊的耳朵和腿:
```python
# 绘制左耳朵
left_ear = plt.Polygon(np.array([[1, 15], [3, 18], [0, 20]]), fc='white')
ax.add_patch(left_ear)
# 绘制右耳朵
right_ear = plt.Polygon(np.array([[9, 15], [7, 18], [10, 20]]), fc='white')
ax.add_patch(right_ear)
# 绘制左腿
left_leg = plt.Rectangle((1, 1), 2, 8, fc='white')
ax.add_patch(left_leg)
# 绘制右腿
right_leg = plt.Rectangle((7, 1), 2, 8, fc='white')
ax.add_patch(right_leg)
```
最后,使用matplotlib的绘图函数显示绘制的喜羊羊图像并保存:
```python
# 设置坐标轴范围
ax.set_xlim(0, 10)
ax.set_ylim(0, 20)
# 隐藏坐标轴
ax.axis('off')
# 保存图片
plt.savefig('XiYangYang.png', dpi=300)
# 显示图片
plt.show()
```
以上就是使用Python绘制喜羊羊的代码,运行代码将生成一张名为"XiYangYang.png"的喜羊羊图像。
阅读全文
相关推荐



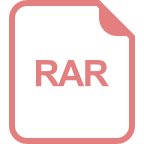




