在Spring Boot框架下架构web评论系统,实体类有帖子、用户和评论,给出具有展示帖子和下面的评论、发表、查询的html代码和javascript代码
时间: 2024-02-04 15:02:25 浏览: 106
好的,以下是一个简单的示例,展示如何使用HTML和JavaScript来展示帖子和评论,以及发表和查询评论。
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>评论系统</title>
</head>
<body>
<h1>帖子列表</h1>
<ul id="postList"></ul>
<h2>发表评论</h2>
<form id="commentForm">
<label>姓名:</label>
<input type="text" name="name" required><br>
<label>内容:</label>
<textarea name="content" required></textarea><br>
<button type="submit">发表评论</button>
</form>
<h2>查询评论</h2>
<form id="searchForm">
<label>关键字:</label>
<input type="text" name="keyword" required><br>
<button type="submit">查询评论</button>
</form>
<h2>评论列表</h2>
<ul id="commentList"></ul>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script src="comment.js"></script>
</body>
</html>
```
JavaScript代码(保存为comment.js文件):
```javascript
// 获取所有帖子
$.get("/api/posts", function(posts) {
var postList = $("#postList");
$.each(posts, function(index, post) {
var listItem = $("<li><a href='/post/" + post.id + "'>" + post.title + "</a></li>");
postList.append(listItem);
});
});
// 发表评论
$("#commentForm").submit(function(event) {
event.preventDefault();
var name = $("input[name='name']").val();
var content = $("textarea[name='content']").val();
var postId = $("#postList li.active").data("post-id");
var comment = {
"name": name,
"content": content,
"postId": postId
};
$.ajax({
type: "POST",
url: "/api/comments",
data: JSON.stringify(comment),
contentType: "application/json",
success: function() {
alert("评论已发表!");
$("#commentForm")[0].reset();
refreshCommentList(postId);
},
error: function() {
alert("评论发表失败!");
}
});
});
// 查询评论
$("#searchForm").submit(function(event) {
event.preventDefault();
var keyword = $("input[name='keyword']").val();
$.get("/api/comments?keyword=" + encodeURIComponent(keyword), function(comments) {
var commentList = $("#commentList");
commentList.empty();
$.each(comments, function(index, comment) {
var listItem = $("<li>" + comment.name + ":" + comment.content + "</li>");
commentList.append(listItem);
});
});
});
// 刷新评论列表
function refreshCommentList(postId) {
$.get("/api/comments?postId=" + postId, function(comments) {
var commentList = $("#commentList");
commentList.empty();
$.each(comments, function(index, comment) {
var listItem = $("<li>" + comment.name + ":" + comment.content + "</li>");
commentList.append(listItem);
});
});
}
// 点击帖子时切换活动状态
$("#postList").on("click", "li", function() {
$("#postList li.active").removeClass("active");
$(this).addClass("active");
var postId = $(this).data("post-id");
refreshCommentList(postId);
});
```
注意,上述代码只是一个简单的示例,实际开发中还需要考虑更多的细节和安全性问题。
阅读全文
相关推荐
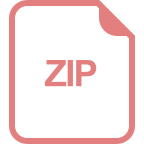
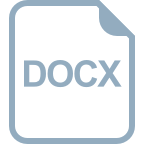
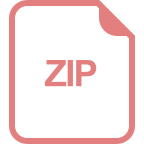
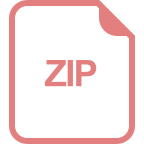
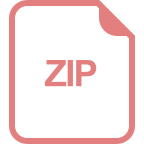
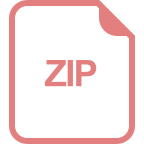
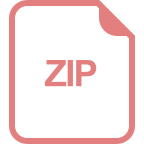
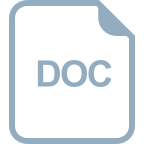
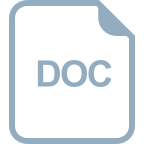
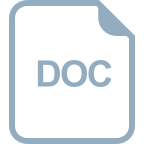
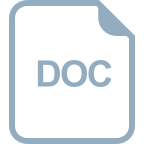
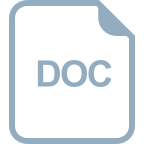
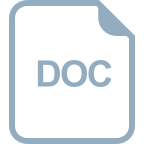
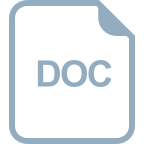
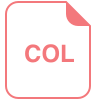
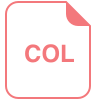
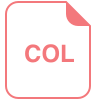
