利用C语言建立一个描述学生成绩的无序链表,各结点内容如表8.3所示。计算各学生的总成绩,并输出链表中各学生结点的信息内容。最后删除链表,收回链表占用的存储空间。建立无序链表、计算总成绩、输出链表、删除链表各用一个函数实现。在主函数中调用四个函数完成上述操作。
时间: 2024-03-13 13:21:43 浏览: 64
好的,您的问题是如何利用C语言建立一个描述学生成绩的无序链表,并计算各学生的总成绩,最后删除链表并收回存储空间,对吧?
好的,我可以给您提供一份示例代码,您可以根据需要进行修改和优化。
代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 20
// 学生信息结构体
typedef struct student {
char name[MAX_NAME_LEN]; // 学生姓名
int chinese; // 语文成绩
int math; // 数学成绩
int english; // 英语成绩
int total; // 总成绩
struct student *next; // 指向下一个结点的指针
} student_t;
// 创建新结点
student_t* create_node(char *name, int chinese, int math, int english)
{
student_t *node = (student_t*)malloc(sizeof(student_t));
if (node == NULL) {
printf("Failed to allocate memory!\n");
exit(-1);
}
strncpy(node->name, name, MAX_NAME_LEN);
node->chinese = chinese;
node->math = math;
node->english = english;
node->total = chinese + math + english;
node->next = NULL;
return node;
}
// 插入新结点到链表尾部
void insert_node(student_t **head, char *name, int chinese, int math, int english)
{
student_t *node = create_node(name, chinese, math, english);
student_t *curr = *head;
if (curr == NULL) {
// 链表为空,插入作为头结点
*head = node;
} else {
// 遍历链表,找到链表尾部
while (curr->next != NULL) {
curr = curr->next;
}
curr->next = node;
}
}
// 计算链表中各结点的总成绩
void calc_total(student_t *head)
{
student_t *curr = head;
while (curr != NULL) {
curr->total = curr->chinese + curr->math + curr->english;
curr = curr->next;
}
}
// 输出链表中各学生结点的信息内容
void print_list(student_t *head)
{
student_t *curr = head;
printf("%-10s %-10s %-10s %-10s %-10s\n", "Name", "Chinese", "Math", "English", "Total");
while (curr != NULL) {
printf("%-10s %-10d %-10d %-10d %-10d\n", curr->name, curr->chinese, curr->math, curr->english, curr->total);
curr = curr->next;
}
}
// 删除链表
void delete_list(student_t **head)
{
student_t *curr = *head;
while (curr != NULL) {
student_t *tmp = curr;
curr = curr->next;
free(tmp);
}
*head = NULL;
}
int main()
{
// 建立无序链表
student_t *head = NULL;
insert_node(&head, "Tom", 80, 85, 90);
insert_node(&head, "Jerry", 90, 95, 80);
insert_node(&head, "Jack", 85, 90, 85);
// 计算总成绩
calc_total(head);
// 输出链表
print_list(head);
// 删除链表并收回内存空间
delete_list(&head);
return 0;
}
```
以上代码中,我们先定义了一个`student_t`结构体来保存学生信息,包含姓名、语文成绩、数学成绩、英语成绩和总成绩。然后定义了`create_node()`函数来创建新结点,`insert_node()`函数用于将新结点插入到链表尾部,`calc_total()`函数计算链表中各结点的总成绩,`print_list()`函数输出链表中各学生结点的信息内容,`delete_list()`函数用于删除链表并收回内存空间。在主函数中,我们先建立链表,然后计算总成绩,输出链表,最后删除链表并收回内存空间。
阅读全文
相关推荐







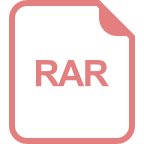



