在vue的开发项目中举几个promise使用的例子
时间: 2024-01-30 16:04:04 浏览: 156
1. 使用 Promise 封装 Ajax 请求:
```javascript
function ajax(url) {
return new Promise(function(resolve, reject) {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4) {
if (xhr.status === 200) {
resolve(xhr.responseText);
} else {
reject(xhr.statusText);
}
}
};
xhr.open('GET', url, true);
xhr.send();
});
}
ajax('/api/data')
.then(function(responseText) {
console.log(responseText);
})
.catch(function(error) {
console.error(error);
});
```
2. 使用 Promise 处理异步操作:
```javascript
function loadImage(src) {
return new Promise(function(resolve, reject) {
var image = new Image();
image.onload = function() {
resolve(image);
};
image.onerror = function() {
reject(new Error('Could not load image at ' + src));
};
image.src = src;
});
}
loadImage('image.png')
.then(function(image) {
document.body.appendChild(image);
})
.catch(function(error) {
console.error(error);
});
```
3. 使用 Promise 处理多个异步操作:
```javascript
function getData() {
return new Promise(function(resolve, reject) {
setTimeout(function() {
resolve('Data');
}, 1000);
});
}
function getMoreData(data) {
return new Promise(function(resolve, reject) {
setTimeout(function() {
resolve(data + ' More Data');
}, 1000);
});
}
function getFinalData(data) {
return new Promise(function(resolve, reject) {
setTimeout(function() {
resolve(data + ' and even more data');
}, 1000);
});
}
getData()
.then(getMoreData)
.then(getFinalData)
.then(function(finalData) {
console.log('Final data:', finalData);
})
.catch(function(error) {
console.error(error);
});
```
阅读全文
相关推荐
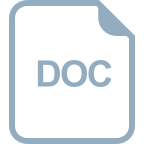
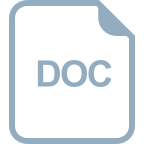
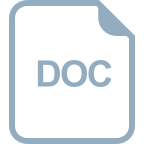
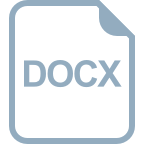
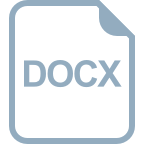
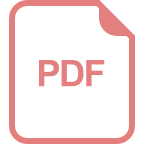
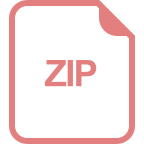
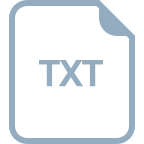
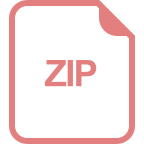
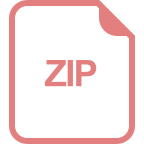
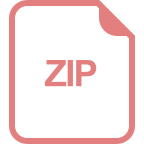
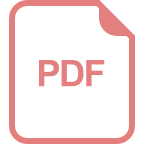
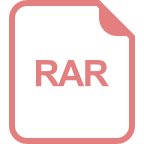
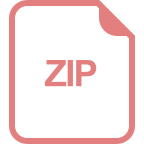
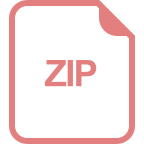
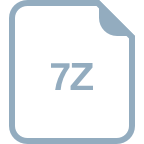