为学生数据库管理系统编写 C++ 程序,完整可运行
时间: 2024-10-11 21:14:12 浏览: 18
为了创建一个完整的、可运行的学生数据库管理系统,你需要包含更多功能,比如用户界面(UI)、输入验证和错误处理。下面是一个更完整的例子,使用C++ CLI库提供命令行交互:
```cpp
#include <iostream>
#include <filesystem>
#include <vector>
#include <string>
#include <algorithm>
// 定义学生类
struct Student {
std::string name;
int id;
double gpa;
};
// 学生数据库类
class StudentDatabase {
private:
std::vector<Student> students;
std::string filename;
public:
// 构造函数
StudentDatabase(const std::string& db_file = "students.txt") : filename(db_file) {
load_from_file();
}
// 加载数据库
void load_from_file() {
if (!std::filesystem::exists(filename)) {
std::cout << "File not found. Creating new database..." << std::endl;
return;
}
std::ifstream file(filename);
if (!file) {
std::cerr << "Error opening file: " << filename << std::endl;
return;
}
std::string line;
while (std::getline(file, line)) {
std::istringstream iss(line);
Student s;
if (!(iss >> s.name >> s.id >> s.gpa)) break; // 读取失败时停止
students.push_back(s);
}
file.close();
}
// 保存数据库到文件
void save_to_file() {
std::ofstream file(filename);
if (!file) {
std::cerr << "Error saving to file: " << filename << std::endl;
return;
}
for (const auto& s : students) {
file << s.name << " " << s.id << " " << s.gpa << "\n";
}
file.close();
}
// 添加学生
bool add_student(const std::string& name, int id, double gpa) {
if (name.empty() || id <= 0 || gpa <= 0) {
std::cerr << "Invalid input. Please enter a valid student record.\n";
return false;
}
students.push_back({name, id, gpa});
save_to_file();
return true;
}
// 查询学生
const Student* find_student(int id) const {
for (const auto& s : students) {
if (s.id == id) return &s;
}
return nullptr;
}
// 删除学生
bool remove_student(int id) {
auto it = std::find_if(students.begin(), students.end(), [id](const auto& s) { return s.id == id; });
if (it != students.end()) {
students.erase(it);
save_to_file();
return true;
}
std::cerr << "Student with ID " << id << " not found.\n";
return false;
}
// 显示所有学生
void display_students() const {
for (const auto& s : students) {
std::cout << s.name << ", ID: " << s.id << ", GPA: " << s.gpa << std::endl;
}
}
};
int main() {
StudentDatabase db;
char command;
while (true) {
std::cout << "> ";
std::cin >> command;
switch (command) {
case 'a': // 添加学生
std::string name, gpa_str;
int id;
std::cout << "Enter student name: ";
std::getline(std::cin, name);
std::cout << "Enter ID: ";
std::cin >> id;
std::cout << "Enter GPA: ";
std::cin >> gpa_str;
double gpa = std::stod(gpa_str);
if (db.add_student(name, id, gpa)) {
std::cout << "Student added successfully!\n";
} else {
break;
}
break;
case 'q': // 查找学生
int search_id;
std::cout << "Enter ID to search: ";
std::cin >> search_id;
const Student* found_student = db.find_student(search_id);
if (found_student) {
found_student->display();
} else {
std::cout << "No student found with that ID.\n";
}
break;
// 其他类似的功能,比如删除和显示
// ...省略...
default:
std::cout << "Unknown command. Type 'a', 'q', or 'd' for actions.\n";
break;
}
}
return 0;
}
```
这个示例提供了基础的添加、查找和显示学生功能。你可以在此基础上继续完善,比如增加删除选项以及优化用户界面。
阅读全文
相关推荐
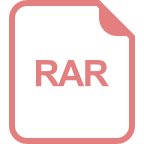
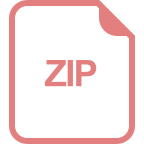
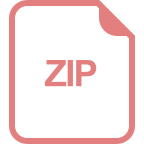
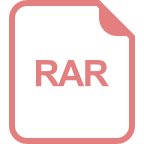
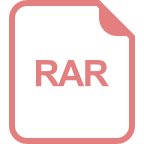
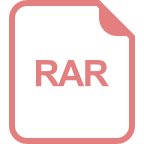
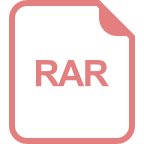
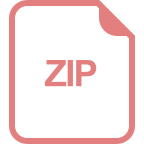
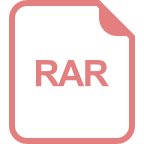
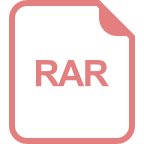
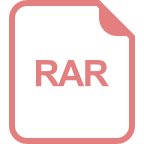
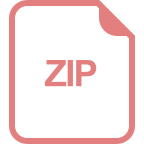
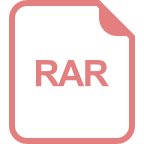
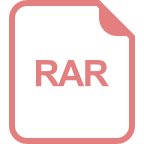
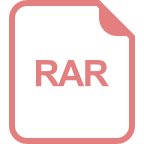
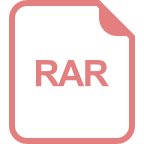
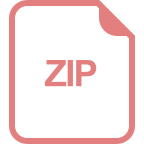
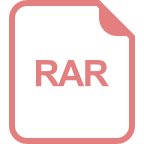
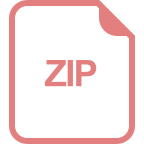