编写main函数测试template情况下线性表的基本功能的正确性
时间: 2023-05-20 14:06:48 浏览: 60
好的,可以的。以下是一个示例的main函数,用于测试template情况下线性表的基本功能的正确性:
```
#include <iostream>
#include "List.h"
using namespace std;
int main() {
List<int> myList;
// 测试插入元素
myList.insert(0, 1);
myList.insert(1, 2);
myList.insert(2, 3);
// 测试获取元素
cout << "第一个元素是:" << myList.get(0) << endl;
cout << "第二个元素是:" << myList.get(1) << endl;
cout << "第三个元素是:" << myList.get(2) << endl;
// 测试删除元素
myList.remove(1);
// 再次测试获取元素
cout << "第一个元素是:" << myList.get(0) << endl;
cout << "第二个元素是:" << myList.get(1) << endl;
return 0;
}
```
这个main函数创建了一个List对象,然后测试了插入、获取和删除元素的功能。你可以根据自己的需要修改这个main函数,测试其他的功能。
相关问题
用C++,设有两条有序链表(即 data 域元素的关键字由前往后不断增大),试设计算法,将这两 条链表合并为一条新的有序链表,原链表不变。两条链表中 data 域关键字相同的元素只选 取一个存储到新的有序链表中,不同的元素都存储到新的有序链表中。 要求: ➢ 直接编写链表的友元函数完成该功能。 ➢ 链表的 data 域可存储用户自定义类对象。 ➢ 编写测试 main()函数测试线性表的正确性
```
#include <iostream>
using namespace std;
template<class T>
class Node{
public:
T data; //数据域
Node<T> *next; //指针域
Node(const T &x, Node<T> *n = NULL){ //构造函数
data = x;
next = n;
}
Node(){next = NULL;} //构造函数
~Node(){} //析构函数
};
template<class T>
class List{
public:
List(){head = new Node<T>; head->next = NULL;} //构造函数
~List(){makeEmpty();} //析构函数
void makeEmpty(); //置空函数
Node<T> *getHead() const{return head;}
int Length() const; //求长度函数
Node<T> *Search(const T &x) const; //查找函数
Node<T> *Locate(int i) const; //定位函数
bool getData(int i, T &x) const; //取数据函数
void setData(int i, const T &x); //设置数据函数
bool Insert(int i, const T &x); //插入函数
bool Remove(int i, T &x); //删除函数
bool IsEmpty() const{return head->next == NULL;} //判断是否为空
bool IsFull() const{return false;} //判断是否为满
void Sort(); //排序函数
void Reverse(); //逆置函数
void Merge(List<T> &list); //合并函数
void Print() const; //输出函数
friend void TestList(); //测试函数
private:
Node<T> *head; //头指针
};
template<class T>
void List<T>::makeEmpty(){ //置空函数
Node<T> *p = head->next, *q;
head->next = NULL;
while(p != NULL){
q = p->next;
delete p;
p = q;
}
}
template<class T>
int List<T>::Length() const{ //求长度函数
Node<T> *p = head->next;
int len = 0;
while(p != NULL){
len++;
p = p->next;
}
return len;
}
template<class T>
Node<T> *List<T>::Search(const T &x) const{ //查找函数
Node<T> *p = head->next;
while(p != NULL && p->data != x)
p = p->next;
return p;
}
template<class T>
Node<T> *List<T>::Locate(int i) const{ //定位函数
if(i < 0) return NULL;
Node<T> *p = head;
int j = -1;
while(p != NULL && j < i){
j++;
p = p->next;
}
return p;
}
template<class T>
bool List<T>::getData(int i, T &x) const{ //取数据函数
if(i < 0) return false;
Node<T> *p = Locate(i);
if(p == NULL) return false;
else{
x = p->data;
return true;
}
}
template<class T>
void List<T>::setData(int i, const T &x){ //设置数据函数
if(i < 0) return;
Node<T> *p = Locate(i);
if(p == NULL) return;
else p->data = x;
}
template<class T>
bool List<T>::Insert(int i, const T &x){ //插入函数
Node<T> *p = Locate(i - 1);
if(p == NULL) return false;
else{
Node<T> *q = new Node<T>(x, p->next);
p->next = q;
return true;
}
}
template<class T>
bool List<T>::Remove(int i, T &x){ //删除函数
Node<T> *p = Locate(i - 1);
if(p == NULL || p->next == NULL) return false;
else{
Node<T> *q = p->next;
x = q->data;
p->next = q->next;
delete q;
return true;
}
}
template<class T>
void List<T>::Sort(){ //排序函数(冒泡排序)
Node<T> *p = head->next, *q;
T temp;
while(p != NULL){
q = p->next;
while(q != NULL){
if(p->data > q->data){
temp = p->data;
p->data = q->data;
q->data = temp;
}
q = q->next;
}
p = p->next;
}
}
template<class T>
void List<T>::Reverse(){ //逆置函数
Node<T> *p = head->next, *q;
head->next = NULL;
while(p != NULL){
q = p->next;
p->next = head->next;
head->next = p;
p = q;
}
}
template<class T>
void List<T>::Merge(List<T> &list){ //合并函数
Node<T> *a = head->next, *b = list.getHead()->next, *c = head;
while(a != NULL && b != NULL){
if(a->data < b->data){
c->next = a;
a = a->next;
c = c->next;
}
else if(a->data > b->data){
c->next = b;
b = b->next;
c = c->next;
}
else{
c->next = a;
a = a->next;
b = b->next;
c = c->next;
}
}
if(a != NULL)
c->next = a;
else if(b != NULL)
c->next = b;
}
template<class T>
void List<T>::Print() const{ //输出函数
Node<T> *p = head->next;
while(p != NULL){
cout << p->data << " ";
p = p->next;
}
cout << endl;
}
void TestList(){ //测试函数
List<int> L1, L2;
int a[5] = {1, 3, 5, 7, 9}, b[5] = {2, 4, 6, 8, 10};
for(int i = 0; i < 5; i++)
L1.Insert(i, a[i]);
for(int i = 0; i < 5; i++)
L2.Insert(i, b[i]);
cout << "L1: ";
L1.Print();
cout << "L2: ";
L2.Print();
L1.Merge(L2);
cout << "L1 after merge: ";
L1.Print();
}
int main(){
TestList();
return 0;
}
```
设有两条有序链表(即 data 域元素的关键字由前往后不断增大),试设计算法,用C++将这两 条链表合并为一条新的有序链表,原链表不变。两条链表中 data 域关键字相同的元素只选 取一个存储到新的有序链表中,不同的元素都存储到新的有序链表中。 要求: ➢ 直接编写链表的友元函数完成该功能。 ➢ 链表的 data 域可存储用户自定义类对象。 ➢ 编写测试 main()函数测试线性表的正确性
下面是一个示例代码:
#include <iostream>
using namespace std;
template<typename T>
class LinkedList {
friend LinkedList<T> merge(LinkedList<T>& list1, LinkedList<T>& list2) {
LinkedList<T> newList;
Node<T>* p1 = list1.head->next;
Node<T>* p2 = list2.head->next;
while(p1 != nullptr && p2 != nullptr) {
if(p1->data < p2->data) {
newList.insertTail(p1->data);
p1 = p1->next;
}
else if(p1->data > p2->data) {
newList.insertTail(p2->data);
p2 = p2->next;
}
else {
newList.insertTail(p1->data);
p1 = p1->next;
p2 = p2->next;
}
}
while(p1 != nullptr) {
newList.insertTail(p1->data);
p1 = p1->next;
}
while(p2 != nullptr) {
newList.insertTail(p2->data);
p2 = p2->next;
}
return newList;
}
public:
LinkedList():head(new Node<T>()) {}
~LinkedList() {
Node<T>* p = head;
while(p != nullptr) {
Node<T>* q = p->next;
delete p;
p = q;
}
}
void insertTail(T data) {
Node<T>* p = head;
while(p->next != nullptr) {
p = p->next;
}
p->next = new Node<T>(data);
}
void print() {
Node<T>* p = head->next;
while(p != nullptr) {
cout << p->data << " ";
p = p->next;
}
cout << endl;
}
private:
template<typename U>
class Node {
public:
Node():next(nullptr) {}
Node(U data):data(data), next(nullptr) {}
U data;
Node* next;
};
Node<T>* head;
};
int main() {
LinkedList<int> list1, list2;
list1.insertTail(1);
list1.insertTail(2);
list1.insertTail(4);
list1.insertTail(6);
list2.insertTail(2);
list2.insertTail(3);
list2.insertTail(5);
list2.insertTail(6);
LinkedList<int> newList = merge(list1, list2);
newList.print(); // 1 2 3 4 5 6
return 0;
}
相关推荐
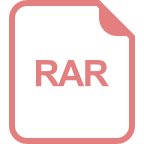
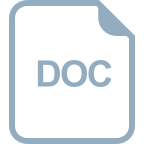
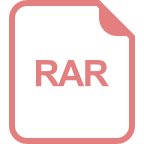


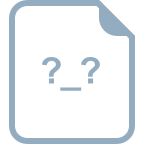
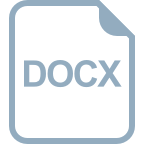
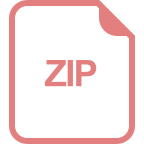
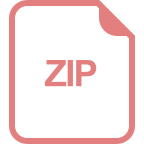
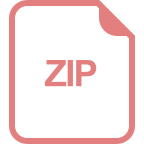
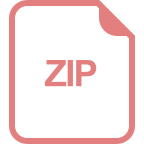