首页Oracle日期函数可以实现哪些功能
Oracle日期函数可以实现哪些功能
时间: 2023-06-04 07:07:24 浏览: 95
Oracle日期函数可以实现日期和时间的格式化、加减、比较、转换等常见操作,比如可以通过TO_CHAR函数将日期转换成特定格式的字符串,通过ADD_MONTHS函数对日期进行加减月份的操作,通过MONTHS_BETWEEN函数计算两个日期之间相差的月份数,等等。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
大家在看
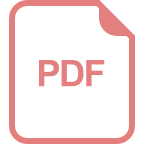
先栅极还是后栅极 业界争论高K技术
随着晶体管尺寸的不断缩小,HKMG(high-k绝缘层+金属栅极)技术几乎已经成为45nm以下级别制程的必备技术.不过在制作HKMG结构晶体管的 工艺方面,业内却存在两大各自固执己见的不同阵营,分别是以IBM为代表的Gate-first(先栅极)工艺流派和以Intel为代表的Gate-last(后栅极)工艺流派,尽管两大阵营均自称只有自己的工艺才是最适合制作HKMG晶体管的技术,但一般来说使用Gate-first工艺实现HKMG结构的难点在于如何控制 PMOS管的Vt电压(门限电压);而Gate-last工艺的难点则在于工艺较复杂,芯片的管芯密度同等条件下要比Gate-first工艺低,需要设 计方积极配合修改电路设计才可以达到与Gate-first工艺相同的管芯密度级别。
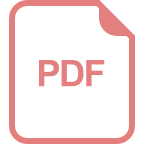
应用手册 - SoftMove.pdf
ABB机器人的SoftMove手册,本手册是中文版,中文版,中文版,重要的事情说三遍,ABB原版手册是英文的,而这个手册是中文的。
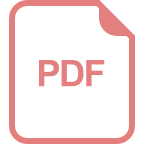
LQR与PD控制在柔性机械臂中的对比研究
LQR与PD控制在柔性机械臂中的对比研究,路恩,杨雪锋,针对单杆柔性机械臂末端位置控制的问题,本文对柔性机械臂振动主动控制中较为常见的LQR和PD方法进行了控制效果的对比研究。首先,�
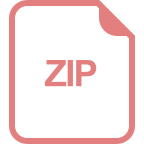
丹麦电力电价预测 预测未来24小时的电价 pytorch + lstm + 历史特征和价格 + 时间序列
pytorch + lstm + 历史特征和价格 + 时间序列
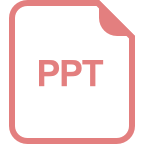
测量变频损耗L的方框图如图-所示。-微波电路实验讲义
测量变频损耗L的方框图如图1-1所示。
图1-1 实验线路
实验线路连接
本振源
信号源
功率计
定向耦合器
超高频毫伏表
滤波器 50Ω
混频器
毫安表
最新推荐
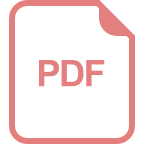
oracle数据库实现获取时间戳的无参函数
总结起来,Oracle数据库通过`SYSDATE`和`SYSTIMESTAMP`系统函数可以方便地获取当前日期和时间,通过适当的转换和计算,我们可以获取到自1970年以来的时间戳,无论是秒级还是毫秒级。这个`GETMSTIMESTAMP`函数提供了...
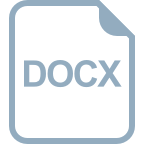
TiDB&MySql&Oracle介绍及区别
(2) 水平弹性扩展:TiDB 采用无中心的设计,可以方便地添加或减少节点,实现存储和计算资源的水平扩展。 (3) 分布式事务:TiDB 支持 ACID 事务,确保在分布式环境下数据的一致性和完整性。 (4) 真正金融级高可用...
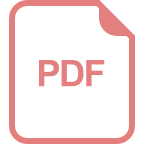
Oracle数据库按时间进行分组统计数据的方法
总之,Oracle数据库提供了丰富的工具来处理时间相关的数据分析,包括灵活的日期函数、分组函数以及递归查询机制。这些功能使得开发者能够高效地对时间序列数据进行分组统计,从而揭示出隐藏在大量数据背后的模式和...
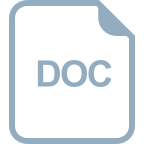
Oracle SQL 函数使用说明
Oracle SQL 函数是数据库查询和数据处理中的重要工具,提供了丰富的功能来处理各种数据类型。在Oracle 8i版本中,这些函数包括但不限于DECODE、LPAD、TO_DATE和TO_CHAR等,它们帮助用户进行条件判断、字符串操作、...
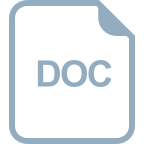
Oracle数据库经典学习教程
Oracle支持多种数据类型,如数值型(NUMBER、INTEGER)、字符型(VARCHAR2、CHAR)、日期时间型(DATE)、二进制数据(BLOB、CLOB)等,每种类型都有其特定的用途和限制。 9. **创建表和约束** 创建表是数据库设计...
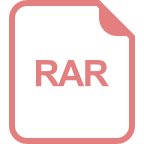
S7-PDIAG工具使用教程及技术资料下载指南
资源摘要信息:"s7upaadk_S7-PDIAG帮助"
s7upaadk_S7-PDIAG帮助是针对西门子S7系列PLC(可编程逻辑控制器)进行诊断和维护的专业工具。S7-PDIAG是西门子提供的诊断软件包,能够帮助工程师和技术人员有效地检测和解决S7 PLC系统中出现的问题。它提供了一系列的诊断功能,包括但不限于错误诊断、性能分析、系统状态监控以及远程访问等。
S7-PDIAG软件广泛应用于自动化领域中,尤其在工业控制系统中扮演着重要角色。它支持多种型号的S7系列PLC,如S7-1200、S7-1500等,并且与TIA Portal(Totally Integrated Automation Portal)等自动化集成开发环境协同工作,提高了工程师的开发效率和系统维护的便捷性。
该压缩包文件包含两个关键文件,一个是“快速接线模块.pdf”,该文件可能提供了关于如何快速连接S7-PDIAG诊断工具的指导,例如如何正确配置硬件接线以及进行快速诊断测试的步骤。另一个文件是“s7upaadk_S7-PDIAG帮助.chm”,这是一个已编译的HTML帮助文件,它包含了详细的操作说明、故障排除指南、软件更新信息以及技术支持资源等。
了解S7-PDIAG及其相关工具的使用,对于任何负责西门子自动化系统维护的专业人士都是至关重要的。使用这款工具,工程师可以迅速定位问题所在,从而减少系统停机时间,确保生产的连续性和效率。
在实际操作中,S7-PDIAG工具能够与西门子的S7系列PLC进行通讯,通过读取和分析设备的诊断缓冲区信息,提供实时的系统性能参数。用户可以通过它监控PLC的运行状态,分析程序的执行流程,甚至远程访问PLC进行维护和升级。
另外,该帮助文件可能还提供了与其他产品的技术资料下载链接,这意味着用户可以通过S7-PDIAG获得一系列扩展支持。例如,用户可能需要下载与S7-PDIAG配套的软件更新或补丁,或者是需要更多高级功能的第三方工具。这些资源的下载能够进一步提升工程师解决复杂问题的能力。
在实践中,熟练掌握S7-PDIAG的使用技巧是提升西门子PLC系统维护效率的关键。这要求工程师不仅要有扎实的理论基础,还需要通过实践不断积累经验。此外,了解与S7-PDIAG相关的软件和硬件产品的技术文档,对确保自动化系统的稳定运行同样不可或缺。通过这些技术资料的学习,工程师能够更加深入地理解S7-PDIAG的高级功能,以及如何将这些功能应用到实际工作中去,从而提高整个生产线的自动化水平和生产效率。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
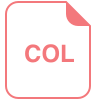
CC-LINK远程IO模块AJ65SBTB1现场应用指南:常见问题快速解决
# 摘要
CC-LINK远程IO模块作为一种工业通信技术,为自动化和控制系统提供了高效的数据交换和设备管理能力。本文首先概述了CC-LINK远程IO模块的基础知识,接着详细介绍了其安装与配置流程,包括硬件的物理连接和系统集成要求,以及软件的参数设置与优化。为应对潜在的故障问题,本文还提供了故障诊断与排除的方法,并探讨了故障解决的实践案例。在高级应用方面,文中讲述了如何进行编程与控制,以及如何实现系统扩展与集成。最后,本文强调了CC-LINK远程IO模块的维护与管理的重要性,并对未来技术发展趋势进行了展望。
# 关键字
CC-LINK远程IO模块;系统集成;故障诊断;性能优化;编程与控制;维护

python 画一个进度条
在Python中,你可以使用`tkinter`库来创建一个简单的进度条。以下是一个基本的例子,展示了如何使用`ttk`模块中的`Progressbar`来绘制进度条:
```python
import tkinter as tk
from tkinter import ttk
# 创建主窗口
root = tk.Tk()
# 设置进度条范围
max_value = 100
# 初始化进度条
progress_bar = ttk.Progressbar(root, orient='horizontal', length=200, mode='determinate', maximum=m
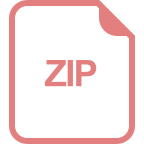
Nginx 1.19.0版本Windows服务器部署指南
资源摘要信息:"nginx-1.19.0-windows.zip"
1. Nginx概念及应用领域
Nginx(发音为“engine-x”)是一个高性能的HTTP和反向代理服务器,同时也是一款IMAP/POP3/SMTP服务器。它以开源的形式发布,在BSD许可证下运行,这使得它可以在遵守BSD协议的前提下自由地使用、修改和分发。Nginx特别适合于作为静态内容的服务器,也可以作为反向代理服务器用来负载均衡、HTTP缓存、Web和反向代理等多种功能。
2. Nginx的主要特点
Nginx的一个显著特点是它的轻量级设计,这意味着它占用的系统资源非常少,包括CPU和内存。这使得Nginx成为在物理资源有限的环境下(如虚拟主机和云服务)的理想选择。Nginx支持高并发,其内部采用的是多进程模型,以及高效的事件驱动架构,能够处理大量的并发连接,这一点在需要支持大量用户访问的网站中尤其重要。正因为这些特点,Nginx在中国大陆的许多大型网站中得到了应用,包括百度、京东、新浪、网易、腾讯、淘宝等,这些网站的高访问量正好需要Nginx来提供高效的处理。
3. Nginx的技术优势
Nginx的另一个技术优势是其配置的灵活性和简单性。Nginx的配置文件通常很小,结构清晰,易于理解,使得即使是初学者也能较快上手。它支持模块化的设计,可以根据需要加载不同的功能模块,提供了很高的可扩展性。此外,Nginx的稳定性和可靠性也得到了业界的认可,它可以在长时间运行中维持高效率和稳定性。
4. Nginx的版本信息
本次提供的资源是Nginx的1.19.0版本,该版本属于较新的稳定版。在版本迭代中,Nginx持续改进性能和功能,修复发现的问题,并添加新的特性。开发团队会根据实际的使用情况和用户反馈,定期更新和发布新版本,以保持Nginx在服务器软件领域的竞争力。
5. Nginx在Windows平台的应用
Nginx的Windows版本支持在Windows操作系统上运行。虽然Nginx最初是为类Unix系统设计的,但随着版本的更新,对Windows平台的支持也越来越完善。Windows版本的Nginx可以为Windows用户提供同样的高性能、高并发以及稳定性,使其可以构建跨平台的Web解决方案。同时,这也意味着开发者可以在开发环境中使用熟悉的Windows系统来测试和开发Nginx。
6. 压缩包文件名称解析
压缩包文件名称为"nginx-1.19.0-windows.zip",这表明了压缩包的内容是Nginx的Windows版本,且版本号为1.19.0。该文件包含了运行Nginx服务器所需的所有文件和配置,用户解压后即可进行安装和配置。文件名称简洁明了,有助于用户识别和确认版本信息,方便根据需要下载和使用。
7. Nginx在中国大陆的应用实例
Nginx在中国大陆的广泛使用,证明了其在实际部署中的卓越表现。这包括但不限于百度、京东、新浪、网易、腾讯、淘宝等大型互联网公司。这些网站的高访问量要求服务器能够处理数以百万计的并发请求,而Nginx正是凭借其出色的性能和稳定性满足了这一需求。这些大型网站的使用案例为Nginx带来了良好的口碑,同时也证明了Nginx作为一款服务器软件的领先地位。
总结以上信息,Nginx-1.19.0-windows.zip是一个适用于Windows操作系统的Nginx服务器软件压缩包,提供了高性能的Web服务和反向代理功能,并被广泛应用于中国大陆的大型互联网企业中。用户在使用该压缩包时,可以期待一个稳定、高效且易于配置的服务器环境。