使用AndroidStudio完成一个简易备忘录功能,主页点击加号按钮,弹出可编辑对话框,点击保存之后动态在该布局加入数据并使用sharedpreferencs保存在该页面,动态增长该布局的长度;点击减号删除该信息,以上功能都在主界面成,请写出示例代码
时间: 2024-02-11 08:10:00 浏览: 20
好的,下面是一个简单的实现备忘录功能的示例代码,供您参考:
1. 主界面布局文件 activity_main.xml:
```xml
<LinearLayout
android:id="@+id/notes_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<!-- 加号按钮 -->
<Button
android:id="@+id/add_note_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="+"
android:textSize="20sp" />
</LinearLayout>
```
2. 备忘录信息布局文件 item_note.xml:
```xml
<LinearLayout
android:id="@+id/note_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="10dp">
<TextView
android:id="@+id/note_text"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:textSize="18sp" />
<!-- 减号按钮 -->
<Button
android:id="@+id/delete_note_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="-"
android:textSize="20sp" />
</LinearLayout>
```
3. 主界面对应的 Activity 类 MainActivity.java:
```java
public class MainActivity extends AppCompatActivity {
private LinearLayout notesLayout;
private Button addNoteBtn;
private SharedPreferences sharedPreferences;
private int notesCount;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
notesLayout = findViewById(R.id.notes_layout);
addNoteBtn = findViewById(R.id.add_note_btn);
// 获取保存备忘录信息的 SharedPreferences 对象
sharedPreferences = getSharedPreferences("notes", MODE_PRIVATE);
// 获取当前已有的备忘录数量
notesCount = sharedPreferences.getInt("notes_count", 0);
// 加载已保存的备忘录信息
for (int i = 0; i < notesCount; i++) {
String note = sharedPreferences.getString("note_" + i, "");
addNoteView(note);
}
// 点击加号按钮弹出对话框
addNoteBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showAddNoteDialog();
}
});
}
// 弹出添加备忘录对话框
private void showAddNoteDialog() {
final EditText editText = new EditText(this);
new AlertDialog.Builder(this)
.setTitle("添加备忘录")
.setView(editText)
.setPositiveButton("保存", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
String note = editText.getText().toString().trim();
if (!TextUtils.isEmpty(note)) {
addNoteView(note);
saveNoteToSharedPreferences(note);
}
}
})
.setNegativeButton("取消", null)
.show();
}
// 动态添加备忘录信息到界面
private void addNoteView(String note) {
View noteView = getLayoutInflater().inflate(R.layout.item_note, null);
TextView noteText = noteView.findViewById(R.id.note_text);
Button deleteNoteBtn = noteView.findViewById(R.id.delete_note_btn);
noteText.setText(note);
// 点击减号按钮删除备忘录信息
deleteNoteBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
notesLayout.removeView(noteView);
removeNoteFromSharedPreferences(note);
}
});
notesLayout.addView(noteView);
}
// 将备忘录信息保存到 SharedPreferences 中
private void saveNoteToSharedPreferences(String note) {
SharedPreferences.Editor editor = sharedPreferences.edit();
editor.putString("note_" + notesCount, note);
editor.putInt("notes_count", ++notesCount);
editor.apply();
}
// 从 SharedPreferences 中删除备忘录信息
private void removeNoteFromSharedPreferences(String note) {
SharedPreferences.Editor editor = sharedPreferences.edit();
for (int i = 0; i < notesCount; i++) {
if (note.equals(sharedPreferences.getString("note_" + i, ""))) {
editor.remove("note_" + i);
break;
}
}
editor.putInt("notes_count", --notesCount);
editor.apply();
}
}
```
以上代码实现了一个简单的备忘录功能,包括添加、删除备忘录信息,动态增加和删除备忘录布局等。需要注意的是,此示例代码只是一个简单的实现,还可以进行优化和改进,如添加数据校验、添加滑动删除功能等。
相关推荐
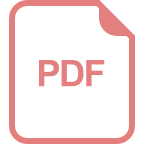
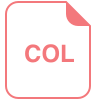
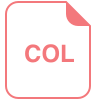
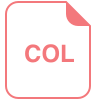
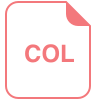
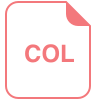









