用汇编程序编写程序,输入一个不小于50的整数n,用递归计算n!,以十进制数输出。
时间: 2024-02-12 21:06:37 浏览: 31
以下是汇编程序的代码,使用的是x86汇编语言:
```
section .data
prompt db "Enter an integer greater than or equal to 50: ", 0
result db "The factorial is: ", 0
newline db 10, 0
section .bss
n resb 4
section .text
global _start
_start:
; output prompt
mov eax, 4
mov ebx, 1
mov ecx, prompt
mov edx, 34
int 0x80
; input n
mov eax, 3
mov ebx, 0
mov ecx, n
mov edx, 4
int 0x80
; convert n to integer
mov eax, dword [n]
sub eax, 48
; check if n is greater than or equal to 50
cmp eax, 50
jl error
; compute factorial recursively
push eax
call factorial
add esp, 4
; output result
mov eax, 4
mov ebx, 1
mov ecx, result
mov edx, 19
int 0x80
; convert result to string and output
push eax
call int_to_string
add esp, 4
mov eax, 4
mov ebx, 1
mov ecx, newline
mov edx, 2
int 0x80
; exit program
mov eax, 1
xor ebx, ebx
int 0x80
factorial:
push ebp
mov ebp, esp
mov eax, [ebp+8]
; base case
cmp eax, 1
je done
; recursive case
dec eax
push eax
call factorial
add esp, 4
; multiply result by n
imul dword [ebp+8]
done:
pop ebp
ret
error:
; output error message
mov eax, 4
mov ebx, 1
mov ecx, newline
mov edx, 2
int 0x80
mov eax, 4
mov ebx, 1
mov ecx, "Error: n must be greater than or equal to 50"
mov edx, 44
int 0x80
; exit program
mov eax, 1
xor ebx, ebx
int 0x80
int_to_string:
push ebp
mov ebp, esp
mov eax, [ebp+8]
; handle negative numbers
cmp eax, 0
jge positive
neg eax
mov byte [string], '-'
inc dword [length]
positive:
; convert number to string
mov ebx, 10
div ebx
push edx
cmp eax, 0
je done2
call int_to_string
add esp, 4
done2:
; add current digit to string
pop eax
add eax, 48
mov byte [string+dword[length]], al
inc dword [length]
pop ebp
ret
section .bss
string resb 11
length resd 1
```
这段程序首先输出提示信息,然后获取输入的整数n。程序会检查n是否大于或等于50,并输出错误消息(并退出程序)如果不是。如果n大于或等于50,则程序调用一个递归函数来计算n的阶乘。最后,程序将结果转换为字符串并输出。
请注意,此程序仅适用于32位x86架构,并且需要使用Linux操作系统来运行。
相关推荐
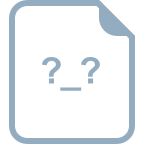
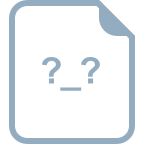

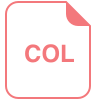


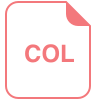









