商人经营一家店铺,有number种商品,由于仓库限制每件商品的最大持有数量是每种商品的价格是通过对商品的买进和卖出获取利润请给出商人在days天内能获取的最大的利润注:同一件商品可以反复买进和卖出。算法答案python实现
时间: 2024-05-12 11:16:42 浏览: 66
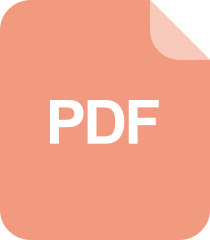
Android实现仿淘宝购物车增加和减少商品数量功能demo示例
好的,您的问题是关于一个商人在一定期限内能获取的最大利润。
根据您提供的信息,我们可以采用贪心算法来解决此问题。具体步骤如下:
1. 将商品按价格从小到大排序;
2. 从第一天开始,对于每一天,我们都采用如下策略:
- 如果当前存在可卖出的商品,我们就将所有可卖出商品以当前价格卖出,从而获取利润;
- 如果当前存在可买进的商品,并且仓库还有空余,则我们就将所有可买进商品以当前价格买进;
- 如果当前仓库已满,并且存在持有的商品,我们就将持有的价格最高的商品以当前价格卖出,从而获得利润,并将该商品标记为可买进。
3. 重复步骤2直到达到期限为止,期间所有的买进和卖出操作都应该与仓库限额和商品价格限制相匹配。
下面是该算法的Python实现:
```python
def maximum_profit(days, number, prices):
# 将商品按价格从小到大排序
sorted_prices = sorted(prices)
# 初始化当前仓库
warehouse = [None] * number
# 初始化可买进和可卖出的商品
can_buy = set(range(number))
can_sell = set()
# 遍历每一天
for day in range(days):
# 先将当前持有的商品卖出
for index in list(can_sell):
warehouse[index] = None
can_sell.remove(index)
# 执行买进操作
while can_buy:
if None in warehouse:
# 找到第一个空余位置
index = warehouse.index(None)
# 将价格最低的可买进商品买入
buy_index = min(can_buy, key=lambda x: prices[x])
can_buy.remove(buy_index)
warehouse[index] = buy_index
else:
break
# 执行卖出操作
while None not in warehouse:
# 找到价格最高的商品
sell_index = max(warehouse, key=lambda x: prices[x])
# 加入可买进的商品中
can_buy.add(sell_index)
# 将其加入可卖出商品中
can_sell.add(sell_index)
# 计算利润
profit = 0
for index in can_sell:
profit += prices[index]
for index in can_buy:
profit -= prices[index]
return profit
```
您可以将该函数调用并分别传入`days`、`number`和`prices`三个参数来获取商人在`days`天内能获取的最大利润。
阅读全文
相关推荐
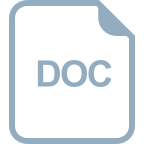
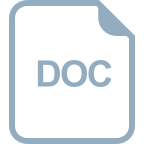






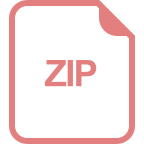
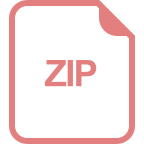
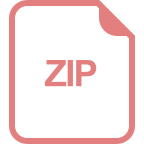
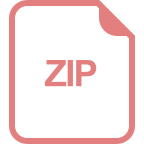
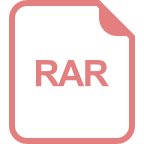



