C# Split() 多个条件
时间: 2023-07-12 21:48:07 浏览: 34
在 C# 中,可以使用 Split() 方法来将字符串分割成一个字符串数组,可以使用多个条件进行分割。具体实现方式如下:
```csharp
string str = "Hello|World;This,Is.a-Test";
string[] separators = { "|", ";", ",", "." };
string[] result = str.Split(separators, StringSplitOptions.RemoveEmptyEntries);
foreach (string s in result)
{
Console.WriteLine(s);
}
```
在上述代码中,我们定义了一个字符串变量 `str`,并定义了一个字符串数组 `separators`,其中包含多个分隔符。然后使用 Split() 方法将 `str` 字符串按照 `separators` 数组中的分隔符进行分割,最终得到一个字符串数组 `result`。最后使用 foreach 循环遍历 `result` 数组,输出分割后的每个字符串。
以上代码输出结果为:
```
Hello
World
This
Is
a
Test
```
相关问题
c# Split
在 C# 中,Split 方法用于将一个字符串按照指定的分隔符分割成多个子字符串,并将这些子字符串保存到一个数组中。语法如下:
```
public string[] Split(params char[] separator)
```
其中,separator 参数是一个可选的参数,表示指定的分隔符。如果不指定分隔符,则默认使用空格字符作为分隔符。
下面是一个示例,将一个字符串按照逗号分隔成多个子字符串:
```
string str = "C#,Java,Python";
string[] arr = str.Split(',');
foreach (string s in arr)
{
Console.WriteLine(s);
}
```
输出结果:
```
C#
Java
Python
```
在这个示例中,我们首先定义了一个字符串 str,然后使用 Split 方法将其按照逗号分隔成多个子字符串,并将这些子字符串保存到一个数组 arr 中。最后,使用 foreach 循环遍历数组 arr,并逐个输出其中的元素。
c# split
C# 中的 `Split` 方法可以用于将字符串按照指定的字符或字符串进行分割,返回一个字符串数组。具体用法如下:
```csharp
string str = "hello,world";
string[] arr = str.Split(','); // 按照逗号分割字符串
// arr 现在为 ["hello", "world"]
```
还可以指定多个分割字符或字符串,例如:
```csharp
string str = "hello world|this is a test";
string[] arr = str.Split(new char[] { ' ', '|' }); // 按照空格和竖线分割字符串
// arr 现在为 ["hello", "world", "this", "is", "a", "test"]
```
还可以指定分割的最大次数,例如:
```csharp
string str = "hello world this is a test";
string[] arr = str.Split(new char[] { ' ' }, 2); // 最多分割 2 次,即将字符串分为两个元素
// arr 现在为 ["hello", "world this is a test"]
```
相关推荐
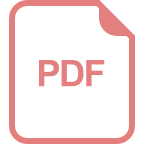
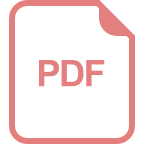












