内部类 LinkedList 删改查
时间: 2024-01-12 12:47:14 浏览: 71
内部类是指在一个类的内部定义的另一个类。在 Java 中,可以在类的内部定义另一个类作为其内部类,内部类可以访问外部类的私有成员,也可以实现封装和隐藏。
LinkedList 是一个双向链表的实现,它提供了一系列的方法来操作链表。下面是一些常用的方法:
1. add():添加元素到链表的末尾。
2. addFirst():添加元素到链表的头部。
3. addLast():添加元素到链表的末尾。
4. remove():删除链表中指定的元素。
5. removeFirst():删除链表的头部元素。
6. removeLast():删除链表的末尾元素。
7. get():获取链表中指定位置的元素。
8. set():将链表中指定位置的元素替换成新的元素。
9. size():获取链表中元素的个数。
下面是一个使用内部类的 LinkedList 的例子:
```java
import java.util.LinkedList;
public class MyLinkedList {
private LinkedList<Integer> list;
public MyLinkedList() {
list = new LinkedList<>();
}
public void add(int value) {
list.add(value);
}
public void remove(int value) {
list.remove(new Integer(value));
}
public int get(int index) {
return list.get(index);
}
public void set(int index, int value) {
list.set(index, value);
}
public int size() {
return list.size();
}
// 内部类,用于实现遍历链表
private class Node {
private int index;
private Node next;
public Node(int index, Node next) {
this.index = index;
this.next = next;
}
public boolean hasNext() {
return next != null;
}
public int next() {
int value = list.get(index);
index++;
next = hasNext() ? new Node(index, next.next) : null;
return value;
}
}
// 遍历链表
public void traverse() {
Node node = new Node(0, null);
while (node.hasNext()) {
System.out.println(node.next());
}
}
}
```
在这个例子中,我们使用了一个内部类 Node 来实现遍历链表。Node 类维护了一个索引 index 和一个下一个节点的引用 next,它的 next() 方法返回当前节点的元素值并将索引加一,然后更新 next 的值。traverse() 方法创建一个 Node 对象来遍历链表,并打印每个元素的值。其他的方法则是基本的对链表的操作。
阅读全文
相关推荐

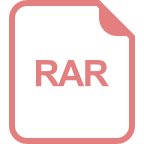


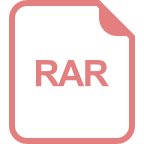
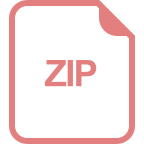
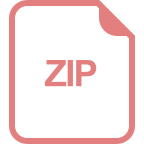
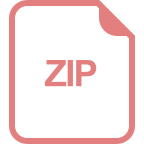
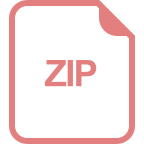
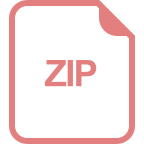
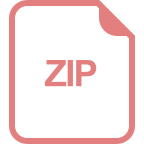
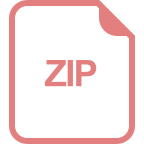