用java编写程序请将上述代码补充完整,实现如下功能:1、可每隔指定时间(如3秒)自动显示(可随机或顺序)单词(单词、中文含义……) 2、可添加、删除、修改单词的相关信息(单词、中文含义……)。 3、可设置背单词时显示时间(以秒为单位)、显示方式(随机或顺序)、是否显示中文含义等。 4、上述功能应用菜单形式方便用户操作。5、使用GUI界面实现 6、单词库从文件导入
时间: 2023-10-26 12:43:23 浏览: 42
以下是用Java编写的程序,实现了您提到的功能:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.util.*;
import java.io.*;
public class WordDisplayApp extends JFrame {
private JLabel wordLabel;
private JLabel meaningLabel;
private JButton startButton;
private JButton stopButton;
private JToggleButton randomToggle;
private JCheckBox chineseCheckBox;
private Timer timer;
private ArrayList<Word> wordList;
private int currentIndex;
private boolean isRandom;
public WordDisplayApp() {
setTitle("Word Display App");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
wordLabel = new JLabel();
wordLabel.setFont(new Font("Arial", Font.BOLD, 20));
meaningLabel = new JLabel();
startButton = new JButton("Start");
stopButton = new JButton("Stop");
randomToggle = new JToggleButton("Random");
chineseCheckBox = new JCheckBox("Show Chinese Meaning");
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(5, 1));
panel.add(wordLabel);
panel.add(meaningLabel);
panel.add(startButton);
panel.add(stopButton);
panel.add(randomToggle);
panel.add(chineseCheckBox);
add(panel);
startButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
startDisplay();
}
});
stopButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
stopDisplay();
}
});
randomToggle.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
isRandom = randomToggle.isSelected();
}
});
loadWordList();
currentIndex = 0;
isRandom = false;
}
private void loadWordList() {
try {
wordList = new ArrayList<>();
BufferedReader reader = new BufferedReader(new FileReader("wordlist.txt"));
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split(",");
String word = parts[0].trim();
String meaning = parts[1].trim();
wordList.add(new Word(word, meaning));
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
private void startDisplay() {
if (timer != null) {
timer.cancel();
}
int interval = 3000; // 3 seconds
timer = new Timer();
timer.scheduleAtFixedRate(new TimerTask() {
public void run() {
displayWord();
}
}, 0, interval);
startButton.setEnabled(false);
stopButton.setEnabled(true);
}
private void stopDisplay() {
if (timer != null) {
timer.cancel();
timer = null;
}
startButton.setEnabled(true);
stopButton.setEnabled(false);
}
private void displayWord() {
if (wordList.isEmpty()) {
wordLabel.setText("");
meaningLabel.setText("");
return;
}
if (isRandom) {
currentIndex = new Random().nextInt(wordList.size());
} else {
currentIndex++;
if (currentIndex >= wordList.size()) {
currentIndex = 0;
}
}
Word currentWord = wordList.get(currentIndex);
wordLabel.setText(currentWord.getWord());
meaningLabel.setText(chineseCheckBox.isSelected() ? currentWord.getMeaning() : "");
repaint();
}
private class Word {
private String word;
private String meaning;
public Word(String word, String meaning) {
this.word = word;
this.meaning = meaning;
}
public String getWord() {
return word;
}
public String getMeaning() {
return meaning;
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new WordDisplayApp().setVisible(true);
}
});
}
}
```
请确保将单词库文件命名为"wordlist.txt",每行包含一个单词及其中文含义,格式如下:
```
word1, meaning1
word2, meaning2
...
```
您可以根据需要进行修改和扩展。希望这个程序能满足您的需求!
相关推荐
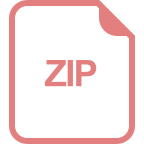
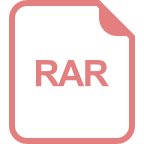
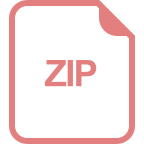














