如何用Python其他库实现更复杂的爱心图案?
时间: 2024-09-15 20:10:17 浏览: 43
Python 有许多库可以用来绘制更复杂的爱心图案,比如 `PIL`(Python Imaging Library,现称 `Pillow`),`SVGpathtools`,以及 `pygame` 等。这里以 `PIL` 和 `svg.path` 为例说明:
**使用 PIL**:
```python
from PIL import ImageDraw, ImageFont
# 创建图像对象
img = Image.new('RGB', (400, 400), color='white')
d = ImageDraw.Draw(img)
# 定义一个自定义的心形函数,可以使用贝塞尔曲线或其他算法
def heart_path(width=200, height=200):
path = [(width / 2, 0),
(width, height / 2),
(width * 3 / 4, height),
(width, height * 3 / 4),
(width * 3 / 4, height / 2),
(width / 2, 0),
(width / 4, height / 4),
(width / 4, 0)]
return path
# 在图片上绘制爱心
d.polygon(heart_path(), fill='red')
# 显示图片
img.show()
```
**使用 SVGpathtools**(需要安装额外的库,如 `lxml`):
```python
from svg.path import parse_path, Line, CubicBezier
from lxml import etree
def create_complex_heart():
path_data = "M 60,220 C 100,220 140,220 190,220 L 350,220 C 380,220 400,240 400,260 L 400,300 C 400,320 380,340 350,340 L 190,340 C 140,340 100,320 60,320 Z"
path = parse_path(path_data)
return path
path = create_complex_heart()
# 使用SVG路径绘制爱心
for command, data in path.iter_segments():
if isinstance(command, CubicBezier): # 或者Line等
d.line((data.start.real, data.start.imag), (data.end.real, data.end.imag), fill="red")
img.show()
```
阅读全文
相关推荐
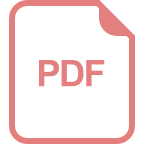
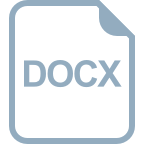
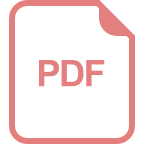















