STM32F103C8T6单片机实现 WS2812,七彩呼吸灯代码,加代码注释
时间: 2024-02-28 20:27:22 浏览: 96
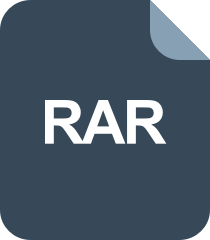
stm32f103c8t6单片机ws281x-spi+dma方式驱动WS2812灯珠的驱动程序源代码.rar

好的,你的第一个问题是关于STM32F103C8T6单片机实现WS2812七彩呼吸灯的代码。以下是代码,同时我也加上了注释方便您理解:
```c
#include "stm32f10x.h"
#include "stdint.h"
#define WS2812_PORT GPIOA
#define WS2812_PIN GPIO_Pin_7
uint8_t color[3]; // 存储颜色值
// 向 WS2812 发送一个 bit
void sendBit(uint8_t bit) {
// 发送数据 0
if (bit == 0) {
// 发送高电平
WS2812_PORT->ODR |= WS2812_PIN;
// 等待 200ns
__NOP(); __NOP(); __NOP();
// 发送低电平
WS2812_PORT->ODR &= ~WS2812_PIN;
// 等待 600ns
__NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP();
}
// 发送数据 1
else {
// 发送高电平
WS2812_PORT->ODR |= WS2812_PIN;
// 等待 600ns
__NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP(); __NOP();
// 发送低电平
WS2812_PORT->ODR &= ~WS2812_PIN;
// 等待 200ns
__NOP(); __NOP(); __NOP();
}
}
// 向 WS2812 发送一个字节
void sendData(uint8_t data) {
for (int i = 0; i < 8; i++) {
sendBit((data & 0x80) >> 7);
data <<= 1;
}
}
// 向 WS2812 发送一个颜色值,R,G,B 三个分量
void sendColor(uint8_t r, uint8_t g, uint8_t b) {
// 数据先发送 G 分量,再发送 R 分量,最后发送 B 分量
sendData(g);
sendData(r);
sendData(b);
}
// 初始化 WS2812 对应的引脚
void initWS2812(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = WS2812_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(WS2812_PORT, &GPIO_InitStructure);
// 首先关闭显示
WS2812_PORT->ODR &= ~WS2812_PIN;
}
// 七彩呼吸灯实现
void breath(void) {
uint32_t i;
float delta = 0.05;
// 红色呼吸
for (i = 0; i <= 100; i++) {
color[0] = i * delta * 255;
color[1] = 0;
color[2] = 0;
sendColor(color[0], color[1], color[2]);
// 每次等待 10ms
for (uint32_t j = 0; j < 200000; j++);
}
for (i = 100; ; i--) {
color[0] = i * delta * 255;
color[1] = 0;
color[2] = 0;
sendColor(color[0], color[1], color[2]);
for (uint32_t j = 0; j < 200000; j++);
if (i == 0) break;
}
// 绿色呼吸
for (i = 0; i <= 100; i++) {
color[0] = 0;
color[1] = i * delta * 255;
color[2] = 0;
sendColor(color[0], color[1], color[2]);
for (uint32_t j = 0; j < 200000; j++);
}
for (i = 100; ; i--) {
color[0] = 0;
color[1] = i * delta * 255;
color[2] = 0;
sendColor(color[0], color[1], color[2]);
for (uint32_t j = 0; j < 200000; j++);
if (i == 0) break;
}
// 蓝色呼吸
for (i = 0; i <= 100; i++) {
color[0] = 0;
color[1] = 0;
color[2] = i * delta * 255;
sendColor(color[0], color[1], color[2]);
for (uint32_t j = 0; j < 200000; j++);
}
for (i = 100; ; i--) {
color[0] = 0;
color[1] = 0;
color[2] = i * delta * 255;
sendColor(color[0], color[1], color[2]);
for (uint32_t j = 0; j < 200000; j++);
if (i == 0) break;
}
}
int main(void) {
initWS2812();
while (1) {
breath();
}
}
```
注释已经在代码中给出了,如果还有什么不清楚的地方,可以继续问我。
阅读全文
相关推荐
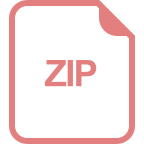
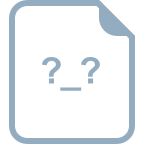
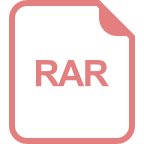
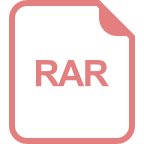
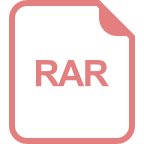
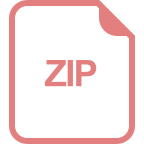
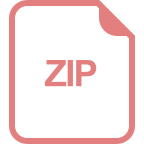
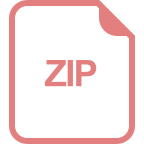
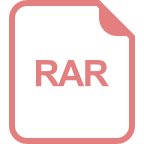
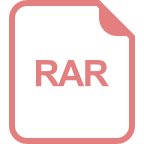
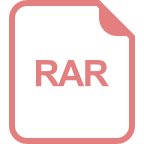
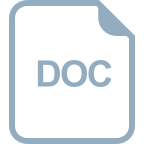