google test单元测试实例
时间: 2023-03-27 08:03:08 浏览: 139
你好,关于 Google Test 单元测试实例,我可以为您提供一些相关的信息和建议。Google Test 是一个流行的 C++ 单元测试框架,它可以帮助开发人员编写高质量的测试代码,以确保软件的正确性和稳定性。您可以在 Google Test 的官方网站上找到详细的文档和示例代码,以了解如何使用该框架进行单元测试。同时,您还可以参考一些开源项目的测试代码,如 Google Chrome 浏览器和 TensorFlow 机器学习框架,以获取更多实践经验和灵感。希望这些信息对您有所帮助。
相关问题
用从谷歌 test 框架写一段实例测试代码
在Google Test框架中,你可以编写简单的单元测试来验证代码的功能。下面是一个基础的示例,展示如何创建一个测试用例,设置断言并运行:
```cpp
#include "gtest/gtest.h" // 引入Google Test库
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
};
// 创建一个名为CalculatorTest的测试类,继承自testing::Test
class CalculatorTest : public ::testing::Test {
protected:
Calculator calculator; // 需要测试的对象
};
// 使用TEST_F宏定义一个测试用例
TEST_F(CalculatorTest, BasicAddition) {
// 设置期望值
int expectedSum = 5;
// 执行操作并获取结果
int result = calculator.add(2, 3);
// 断言实际结果是否等于预期
EXPECT_EQ(result, expectedSum);
}
int main(int argc, char **argv) {
::testing::InitGoogleTest(&argc, argv); // 初始化测试框架
return RUN_ALL_TESTS(); // 执行所有的测试
}
```
在这个例子中,我们测试了一个简单的加法计算器。测试类`CalculatorTest`包含了针对`add`方法的一个测试,检查计算2+3的结果是否等于5。
在使用GoogleTest进行单元测试时,如何自定义环境类并利用SetUp和TearDown方法进行全局初始化和清理?同时,如何在测试套件级别组织和管理测试用例?
要实现GoogleTest中的全局初始化和清理,你需要定义一个继承自`testing::Environment`的环境类,并在其中实现`SetUp()`和`TearDown()`方法。`SetUp()`方法会在所有测试用例之前执行,用于初始化操作;`TearDown()`方法则在所有测试用例执行完毕后执行,用于进行必要的清理工作。创建环境类的示例如下:
参考资源链接:[GoogleTest入门教程:环境设置与全局事件](https://wenku.csdn.net/doc/1hij93jxdz?spm=1055.2569.3001.10343)
```cpp
class MyTestEnvironment : public testing::Environment {
protected:
void SetUp() override {
// 这里可以添加全局的测试前初始化代码
}
void TearDown() override {
// 这里可以添加全局的测试后清理代码
}
};
```
然后,在`main()`函数中将这个环境类的实例添加到全局环境列表中,以便gtest可以在适当的时机调用这些方法:
```cpp
int main(int argc, char **argv) {
::testing::AddGlobalTestEnvironment(new MyTestEnvironment());
::testing::InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
}
```
对于测试套件级别管理测试用例,gtest允许你通过继承`testing::Test`类来创建自己的测试套件,并在其中定义静态方法来控制测试套件的生命周期。测试套件可以通过`TEST_SUITE`宏来定义,测试用例则通过`TEST`宏来创建。例如:
```cpp
class MyTestSuite : public testing::Test {
protected:
static void SetUpTestCase() {
// 初始化测试套件级别的操作
}
static void TearDownTestCase() {
// 清理测试套件级别的操作
}
};
TEST(MyTestSuite, TestCase1) {
// 第一个测试用例
}
TEST(MyTestSuite, TestCase2) {
// 第二个测试用例
}
// 定义测试套件
TEST_SUITE(MyTestSuite);
```
通过这种方式,gtest提供了强大的工具来精细地控制测试的生命周期,确保每个测试用例运行在一个干净的环境中。建议深入阅读《GoogleTest入门教程:环境设置与全局事件》来获取更多关于gtest使用和最佳实践的信息,特别是在处理复杂的测试场景和全局事件时。这份资料不仅涵盖了如何创建自定义的环境类和测试套件,还包括了在实际项目中应用gtest的经验和技巧。
参考资源链接:[GoogleTest入门教程:环境设置与全局事件](https://wenku.csdn.net/doc/1hij93jxdz?spm=1055.2569.3001.10343)
阅读全文
相关推荐
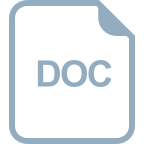
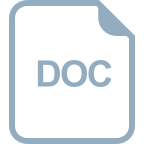
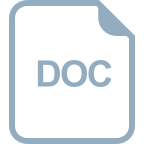
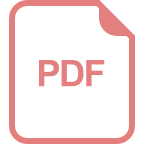
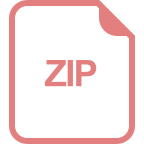
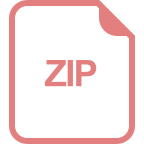
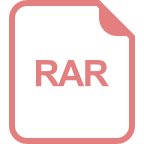
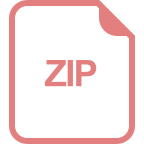
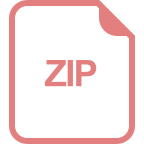
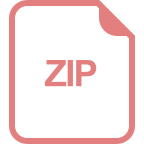
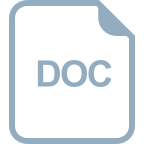
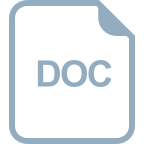
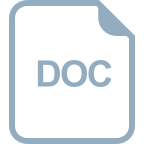
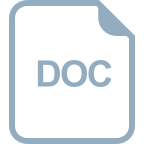
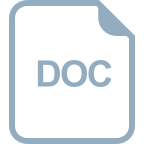
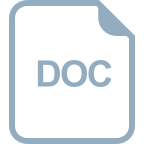
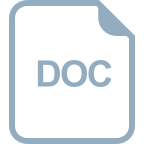