有约束条件求最低运输成本python代码
时间: 2024-02-06 22:03:30 浏览: 59
求解有约束条件的最低运输成本问题可以使用线性规划进行建模和求解。下面是一个使用Python库`scipy`中的`linprog`函数求解最低运输成本问题的代码示例:
```python
import numpy as np
from scipy.optimize import linprog
# 运输成本矩阵
cost_matrix = np.array([[400, 250, 200],
[300, 450, 350],
[200, 400, 250]])
# 供应限制约束
supply_constraint = [100, 150, 200]
# 需求限制约束
demand_constraint = [120, 80, 150]
# 构建线性规划模型
c = cost_matrix.flatten() # 目标函数系数(将矩阵展平成一维数组)
A_eq = [] # 相等约束矩阵
b_eq = [] # 相等约束值
A_ub = [] # 不等约束矩阵
b_ub = [] # 不等约束值
# 构建供应约束
for supply in supply_constraint:
constraint = np.zeros_like(cost_matrix.flatten())
constraint[:cost_matrix.size] = 1
A_eq.append(constraint)
b_eq.append(supply)
# 构建需求约束
for demand in demand_constraint:
constraint = np.zeros_like(cost_matrix.flatten())
constraint[::cost_matrix.shape[1]] = 1
A_eq.append(constraint)
b_eq.append(demand)
# 求解线性规划问题
result = linprog(c, A_eq=A_eq, b_eq=b_eq, bounds=(0, None))
# 输出最优解
print("最低运输成本为:", result.fun)
print("运输方案为:")
print(result.x.reshape(cost_matrix.shape))
```
在这段代码中,我们首先定义了运输成本矩阵`cost_matrix`,供应限制约束`supply_constraint`和需求限制约束`demand_constraint`。然后,我们根据约束条件和目标函数构建了线性规划模型。通过调用`linprog`函数并传入目标函数系数、相等约束矩阵和值、不等约束矩阵和值等参数,来求解线性规划问题。最后,我们输出了最低运输成本和对应的运输方案。
请注意,这只是一个简单的示例,实际应用中可能会有更多的约束条件和变量。你可以根据具体问题进行相应的调整和扩展。另外,还可以使用其他库或工具如`cvxpy`、`PuLP`等来求解线性规划问题。
相关推荐
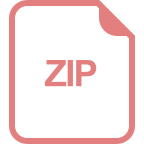
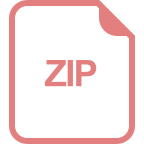














