写一篇Python列表综合应用,读取Excel中数据,读取SQLite
时间: 2024-10-06 08:05:17 浏览: 34
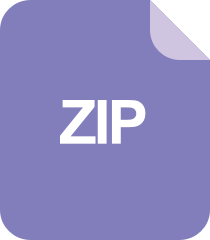
xlrd_pythonsqlite_pythonexcel_
在Python中,我们可以利用pandas库读取Excel文件的数据,并结合sqlite3库处理SQLite数据库。以下是关于这两者结合的一个示例:
首先,我们需要安装必要的库,如pandas(用于读取Excel)、openpyxl(支持XLSX格式)和sqlite3(用于SQLite操作):
```bash
pip install pandas openpyxl sqlite3
```
然后,我们可以创建一个函数,通过pandas的`read_excel`函数读取Excel文件中的数据,并将数据存储在一个DataFrame对象中:
```python
import pandas as pd
def read_excel_to_df(file_path):
try:
excel_data = pd.read_excel(file_path)
return excel_data
except FileNotFoundError:
print(f"文件 '{file_path}' 未找到")
except Exception as e:
print(f"读取Excel文件出错:{str(e)}")
# 使用函数
excel_file = 'example.xlsx'
df_excel = read_excel_to_df(excel_file)
```
接着,我们可以使用sqlite3创建数据库连接并导入数据到SQLite表中:
```python
import sqlite3
def create_connection(db_file):
conn = None
try:
conn = sqlite3.connect(db_file)
print(f"成功连接到SQLite数据库:{db_file}")
except Error as e:
print(f"无法连接到数据库:{e}")
if conn:
return conn
def import_data_to_sqlite(df, table_name, conn):
df.to_sql(table_name, con=conn, index=False, if_exists='replace')
print(f"{table_name} 数据已成功导入到SQLite数据库")
# 创建SQLite连接
conn_sqlite = create_connection('example.db')
# 如果有读取到Excel数据,将其导入SQLite
if df_excel is not None:
import_data_to_sqlite(df_excel, "data_table", conn_sqlite)
else:
print("未读取到Excel数据,因此无法导入SQLite")
# 关闭连接
conn_sqlite.close()
```
这个例子展示了如何读取Excel数据到DataFrame,然后将DataFrame内容写入SQLite数据库。
阅读全文
相关推荐
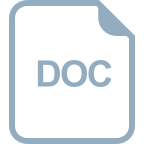
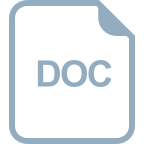
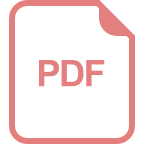
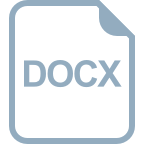
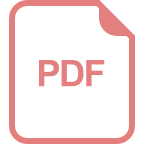
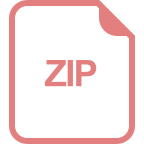
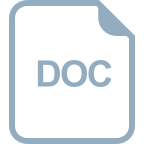










