main.cpp:6:25: error: out-of-line definition of 'Union' does not match any declaration in 'seqList<elemType>'
时间: 2024-09-25 14:04:45 浏览: 166
这个错误提示意味着你在`main.cpp`文件中的`Union`函数定义与`seqList<elemType>`类中没有匹配的声明。通常,当C++编译器遇到这种情况时,它期望找到与函数名和参数列表相匹配的函数原型(即声明)。
解决这个问题的方法有几步:
1. **检查头文件**:确保在`seqList.h`或其他适当的头文件中有一个`Union`函数的声明,类似于这样:
```cpp
class seqList<elemType> {
// ...
void Union(const elemType& item); // 声明函数
};
```
2. **复制到源文件**:如果在头文件中没有声明,可能是在某个源文件里定义过。确保不要在`main.cpp`之外的地方也定义了`Union`。
3. **匹配声明和定义**:确认函数签名(包括返回类型、参数类型和数量)在声明和实现上一致。
4. **头文件包含**:确保`main.cpp`包含了包含`seqList.h`的语句,以便编译器能找到函数声明。
如果已经检查了这些,但仍然有问题,可能是其他代码引入了冲突,需要进一步排查。如果你能提供具体的代码片段,我会更好地帮助你定位问题。
相关问题
seqlist.h:54:25: error: out-of-line definition of 'Union' does not match any declaration in 'seqList<elemType>'
The error message you're encountering suggests that there's a mismatch between the inline declaration (likely in the header file `seqlist.h`) and the actual implementation (line 54) of a function called "Union" or possibly a constructor/destructor for the `seqList` template class. Here's what it means:
1. **Constructor/Destructor Misalignment**: If `Union` refers to a destructor, like the one shown in your first reference[^1], the compiler is looking for an inline definition of `~seqList()` that matches the declaration in the header file. Ensure that the body of the destructor defined in the source (.cpp) file corresponds to the signature declared in `seqlist.h`.
```cpp
// In seqlist.cpp
template <class elemType>
seqList<elemType>::~seqList() {
delete[] data;
}
```
2. **Function Declaration vs Definition**: If `Union` is actually a different member function (e.g., a member function other than the destructor), make sure you've correctly declared it in the header (`seqlist.h`) and then implemented it in the source file.
For example, if `length()` is meant to be inline:
**In seqlist.h**
```cpp
// ...
inline int length() const; // Declare the function as inline
// ...
```
**In seqlist.cpp**
```cpp
// ...
template <class elemType>
int seqList<elemType>::length() const { return currentLength; }
// ...
```
seqlist.h:54:25: error: out-of-line definition of 'Union' does not match any declaration in 'seqList<elemType>'该怎么定义
错误消息表明,在`seqlist.h`的第54行,试图定义名为"Union"的成员函数(可能是析构函数),但这个实现与`seqList<elemType>`类中任何已声明的"Union"函数不匹配。要解决这个问题,您应该确认以下几个步骤:
1. 检查`seqList`模板类是否有一个名为`~seqList()`的析构函数声明。这通常看起来像这样:
```cpp
template <class elemType>
~seqList<elemType>() {
delete[] data;
}
```
2. 如果上述析构函数声明存在,那么在`seqlist.h`的实际实现部分(可能是在某个`.cpp`文件)中,务必确保它的签名一致,包括参数列表和返回类型。
3. 如果没有声明析构函数,确保您需要它,因为如果`seqList`拥有动态分配的数据成员(如`delete[] data;`所示),那么析构函数通常是必需的,用于释放内存资源。
4. 如果`Union`不是指的析构函数,而是其他函数(比如长度函数或其他成员),确保您正确地在类声明中声明并定义了那个函数。对于长度函数`length()`,您的例子已经展示了正确的声明:
```cpp
template <class elemType>
int length() const { return currentLength; }
```
请确保所有相关的函数声明和定义都在同一命名空间内,并且它们的类型签名(包括返回类型和参数)完全匹配。如果有疑问,检查其他相关的类定义或头文件,以找到可能的混淆点。
阅读全文
相关推荐
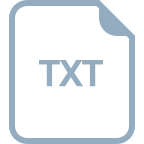
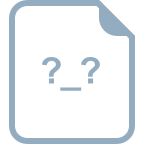
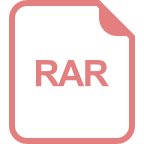














