用c语言解决荷兰国旗问题。假设有一个仅由红、白、蓝3种颜色的条块组成的序列,若给定的彩色条块序列为{蓝、白、红、白、蓝、红、白、白、红、蓝},请用快速排序算法按红、白、蓝的顺序排好,即排成荷兰国旗图案。
时间: 2023-12-28 18:24:36 浏览: 167
荷兰国旗问题可以使用三路快排算法来解决,时间复杂度为O(n)。具体步骤如下:
1.定义三个指针p0、p1、p2,分别指向序列的开头、当前位置和结尾。
2.遍历整个序列,如果当前位置指向的元素为0,则将其与p0指向的元素交换,并将p0和p1都向后移动一位;如果当前位置指向的元素为1,则将p1向后移动一位;如果当前位置指向的元素为2,则将其与p2指向的元素交换,并将p2向前移动一位,此时当前位置不变。
3.重复步骤2,直到当前位置超过p2。
4.排序完成。
以下是C语言实现代码:
```c
#include <stdio.h>
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
void sortColors(int* nums, int numsSize){
int p0 = 0, p1 = 0, p2 = numsSize - 1;
while (p1 <= p2) {
if (nums[p1] == 0) {
swap(&nums[p0], &nums[p1]);
p0++;
p1++;
} else if (nums[p1] == 1) {
p1++;
} else {
swap(&nums[p1], &nums[p2]);
p2--;
}
}
}
int main() {
int nums[] = {2, 1, 0, 1, 2, 0, 1, 1, 0, 2};
int numsSize = sizeof(nums) / sizeof(int);
sortColors(nums, numsSize);
for (int i = 0; i < numsSize; i++) {
printf("%d ", nums[i]);
}
return 0;
}
```
阅读全文
相关推荐
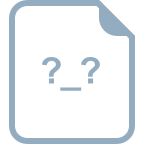
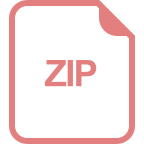
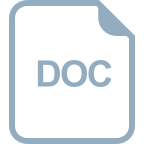
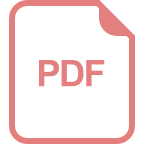
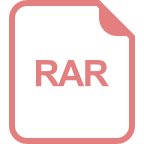
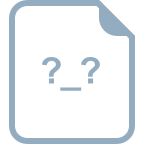
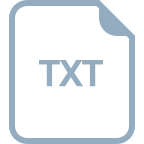
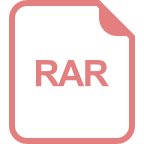
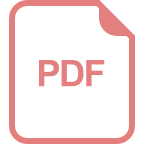
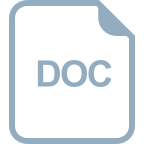
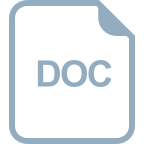
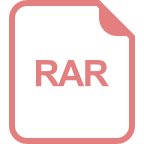
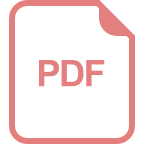
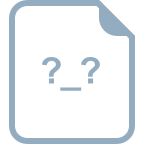