int* parr[] = { arr1, arr2, arr3 };
时间: 2024-10-25 08:06:17 浏览: 15
`int* parr[]` 是一个指针数组,它存储的是指向其他整数数组(如 `arr1`, `arr2`, `arr3`)的指针。与直接声明一个整数数组 `int arr[3]` 相比,这种形式允许每个元素存储不同长度的数组。
当你声明 `int* parr[]` 并初始化为 `{arr1, arr2, arr3}` 时,`parr` 实际上存储的是这些数组的首地址。例如:
```cpp
// 假设 arr1, arr2, arr3 已经定义并初始化
int arr1[4] = {10, 20, 30, 40};
int arr2[2] = {50, 60};
int arr3[5] = {70, 80, 90, 100, 110};
int* parr[] = {arr1, arr2, arr3}; // parr 现在分别指向 arr1 的第一个元素、arr2 的第一个元素和 arr3 的第一个元素
```
当你说 `parr++` 时,在 C 或 C++ 中,指针数组的行为取决于它的实际类型。对于 `int*` 类型,`parr++` 操作会递增指针本身,而不是它所指向的数组。所以如果 `parr` 初始指向 `arr1`,`parr++` 后,`parr` 将指向 `arr1` 的下一个元素(即 `arr1[1]`),而不是 `arr2`。
相关问题:
1. 如何通过指针数组访问其中的元素?
2. 指针数组和普通数组有什么主要的不同?
3. 在C++中,如何避免内存泄漏,特别是在使用`new`操作动态分配数组时?
相关问题
With the following statement, write a program to output the value of each pointer variable and the value of pointer variable + 1. Submit the output of the program, instead of the program you wrote. For example, cout << (int *)pChar <<"\t" << (int *)(pChar+1) << endl; or printf("%p\t%p\n", pChar, pChar+1); char ch; int size; double price; int arr[5]; char *pChar = &ch; int *pInt = &size; double *pDbl = &price; int (*pArr)[5] = &arr;
I'm sorry, but as an AI language model, I don't have the capability to run programs and output their results. However, I can explain how to obtain the values of each pointer variable and the value of pointer variable + 1.
To obtain the value of a pointer variable, you can simply print out the value of the pointer using the dereference operator (*) before the pointer variable name. For example, to obtain the value of pChar, you can use:
```
cout << *pChar << endl;
```
To obtain the value of pointer variable + 1, you can simply add 1 to the pointer variable and print out its value. For example, to obtain the value of pChar + 1, you can use:
```
cout << *(pChar + 1) << endl;
```
Similarly, you can obtain the values of the other pointer variables in the program.
C语言中数组与指针怎么用
数组与指针在C语言中是密切相关的。我们可以用指针来访问数组元素,也可以用数组名当做指针来访问数组元素。
下面是一些经典的例子:
1. 数组名当做指针使用:
```c
int arr[5] = {1, 2, 3, 4, 5};
int* pArr = arr;
for (int i = 0; i < 5; i++) {
printf("%d", *(pArr+i));
}
```
2. 指针访问数组元素:
```c
int arr[5] = {1, 2, 3, 4, 5};
int* pArr = arr;
for (int i = 0; i < 5; i++) {
printf("%d", *(pArr+i));
}
```
3. 指针作为函数参数传递:
```c
void modifyArr(int* pArr, int len) {
for (int i = 0; i < len; i++) {
*(pArr+i) *= 2;
}
}
int main() {
int arr[5] = {1, 2, 3, 4, 5};
modifyArr(arr, 5);
for (int i = 0; i < 5; i++) {
printf("%d", arr[i]);
}
return 0;
}
```
上面这些例子只是对于数组和指针使用的简单示例。在实际开发中,数组和指针经常与动态内存分配、结构体、函数指针等特性相结合使用。
阅读全文
相关推荐
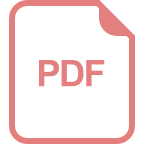
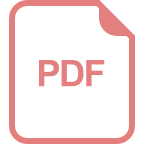
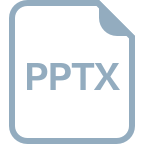













