java 文件异步上传,显示进度条
时间: 2024-01-11 11:48:27 浏览: 145
对于Java文件的异步上传,您可以使用Java的多线程技术,将上传任务放在一个新线程中进行,同时在主线程中显示进度条。其中,您可以使用Java SE 7及其以上版本提供的NIO.2中的AsynchronousFileChannel类来实现文件的异步读取和写入操作。
下面是一个简单的示例代码:
```java
public class FileUploader {
public static void upload(File file, String url, ProgressListener listener) {
// 创建新线程处理上传任务
new Thread(new Runnable() {
@Override
public void run() {
FileInputStream fis = null;
try {
// 打开文件通道
AsynchronousFileChannel channel = AsynchronousFileChannel.open(file.toPath(), StandardOpenOption.READ);
ByteBuffer buffer = ByteBuffer.allocate(1024);
long position = 0;
int count = 0;
int total = 0;
// 读取文件数据并上传至服务器
while ((count = channel.read(buffer, position).get()) != -1) {
position += count;
total += count;
// 触发进度监听器回调方法
listener.onProgress(total, (int) file.length());
// 上传文件数据至服务器
HttpClient httpClient = HttpClient.newBuilder().build();
HttpRequest httpRequest = HttpRequest.newBuilder()
.uri(URI.create(url))
.POST(HttpRequest.BodyPublishers.ofByteArray(buffer))
.build();
HttpResponse<String> httpResponse = httpClient.send(httpRequest, HttpResponse.BodyHandlers.ofString());
// 重置缓冲区
buffer.clear();
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (fis != null) {
fis.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}).start();
}
public interface ProgressListener {
void onProgress(int current, int total);
}
}
```
在上述代码中,`AsynchronousFileChannel`类提供了异步读取文件的功能。调用`read()`方法可以异步读取一定数量的数据,并在读取完成后返回读取到的数据字节长度。您可以将这个长度与文件总长度进行比较,得到当前上传进度,并将进度通过回调函数传递给主线程来更新进度条。
需要注意的是,这只是一个简单的演示代码,具体的文件上传实现会根据不同的具体场景而有所不同,比如还需要处理网络中断和重传、连接数限制等问题。
阅读全文
相关推荐
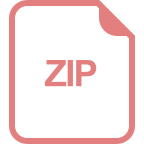
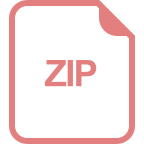
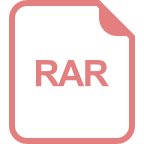
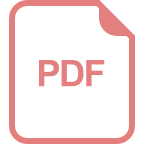
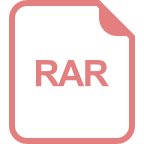
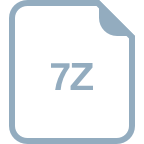
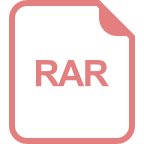
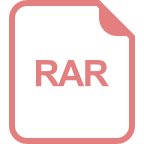
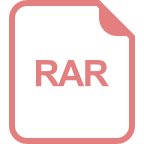
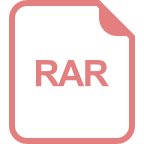
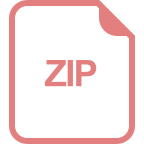
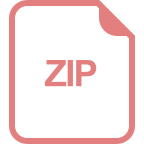
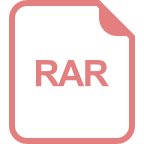
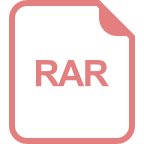
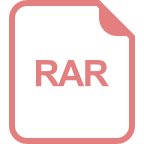
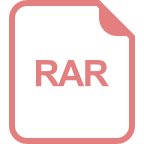