寻找迷宫的最短路径c++
时间: 2023-06-23 07:09:37 浏览: 54
寻找迷宫的最短路径可以使用广度优先搜索(BFS)算法来实现。以下是一个简单的C++代码示例:
```c++
#include <iostream>
#include <queue>
#include <cstring>
using namespace std;
const int MAXN = 100; // 最大迷宫大小
const int dx[] = {0, 0, 1, -1}; // 上下左右四个方向移动的步长
const int dy[] = {1, -1, 0, 0};
int maze[MAXN][MAXN]; // 迷宫地图
int dist[MAXN][MAXN]; // 记录每个位置到起点的最短距离
bool vis[MAXN][MAXN]; // 标记每个位置是否已访问
int n, m; // 迷宫大小
struct Node {
int x, y; // 当前位置
int step; // 走到当前位置的步数
};
bool check(int x, int y) { // 判断当前位置是否越界或障碍物
return x >= 0 && x < n && y >= 0 && y < m && maze[x][y] != 1;
}
int bfs(int sx, int sy, int ex, int ey) { // 搜索起点到终点的最短距离
queue<Node> q;
memset(dist, -1, sizeof(dist)); // 初始化距离为-1
memset(vis, false, sizeof(vis)); // 初始化未访问
Node start = {sx, sy, 0};
q.push(start);
vis[sx][sy] = true;
dist[sx][sy] = 0;
while (!q.empty()) {
Node cur = q.front();
q.pop();
if (cur.x == ex && cur.y == ey) { // 到达终点
return cur.step; // 返回最短距离
}
for (int i = 0; i < 4; i++) { // 四个方向扩展
int nx = cur.x + dx[i];
int ny = cur.y + dy[i];
if (check(nx, ny) && !vis[nx][ny]) { // 如果可以走且未访问过
Node next = {nx, ny, cur.step + 1};
q.push(next);
vis[nx][ny] = true;
dist[nx][ny] = cur.step + 1;
}
}
}
return -1; // 无法到达终点
}
int main() {
cin >> n >> m;
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
cin >> maze[i][j];
}
}
int sx, sy, ex, ey; // 起点和终点坐标
cin >> sx >> sy >> ex >> ey;
int shortest = bfs(sx, sy, ex, ey);
if (shortest == -1) {
cout << "无法到达终点" << endl;
} else {
cout << "最短距离为:" << shortest << endl;
}
return 0;
}
```
在输入迷宫地图和起点终点坐标后,调用`bfs`函数即可返回起点到终点的最短距离。如果无法到达终点,函数返回-1。
相关推荐
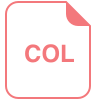
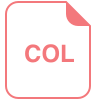












