C语言编写程序:功能:N名学生的成绩已在主函数中放入一个带头节点的链表结构中,h指向链表的头节点。请编写子函数double fun(STREC *h),找出学生的最高分,由函数值返回。 说明,链表的创立建议另定义一子函数实现,并在主函数中调用实现。 注意:链表的节点类型定义如下: struct slist { double s; struct slist *next; }; typedef struct slist STREC;
时间: 2024-03-09 11:46:10 浏览: 63
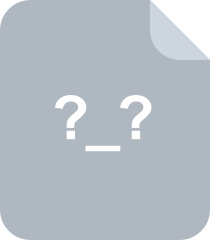
N名学生的成绩已在主函数中放入一个带头节点的链表结构中

好的,以下是符合您要求的 C 语言程序实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct slist {
double s;
struct slist *next;
};
typedef struct slist STREC;
// 链表的创建
STREC* createList(int n) {
STREC *head = (STREC*)malloc(sizeof(STREC)); // 创建头结点
head->next = NULL; // 头结点指针域置为空
STREC *tail = head; // 用 tail 记录链表的尾部
for (int i = 0; i < n; i++) {
STREC *node = (STREC*)malloc(sizeof(STREC)); // 创建新结点
printf("请输入第 %d 个学生的成绩:", i+1);
scanf("%lf", &node->s);
node->next = NULL;
tail->next = node; // 将新结点挂到尾部
tail = node; // 更新尾部指针
}
return head;
}
// 找出最高分
double fun(STREC *h) {
double maxScore = -1; // 初始化为 -1
STREC *p = h->next; // 指向第一个学生结点
while (p != NULL) {
if (p->s > maxScore) {
maxScore = p->s; // 更新最高分
}
p = p->next; // 移动指针到下一个学生结点
}
return maxScore;
}
int main() {
int n;
printf("请输入学生数量:");
scanf("%d", &n);
STREC *head = createList(n);
double maxScore = fun(head);
printf("最高分为:%.2lf\n", maxScore);
return 0;
}
```
程序的逻辑比较简单,首先通过 `createList` 函数创建带头结点的链表,并将链表的头指针传入 `fun` 函数,通过遍历链表找出最高分,最后将最高分返回并输出即可。
阅读全文
相关推荐
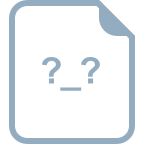
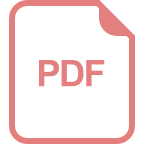
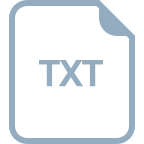
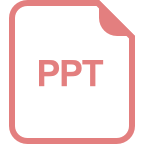
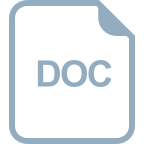
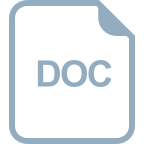
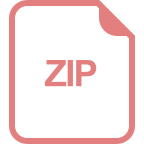
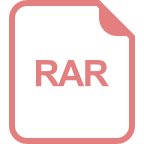
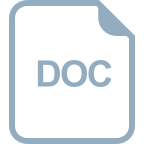
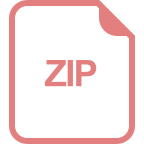
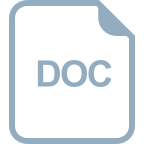
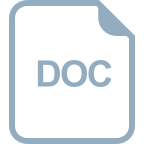
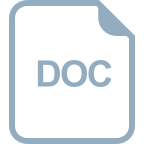
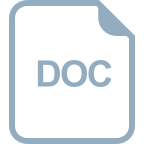
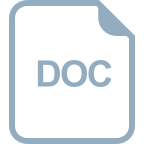