pyqt使用QTimer
时间: 2024-09-27 10:01:08 浏览: 44
PyQt中的`QTimer`是一个非常实用的工具,它允许你在应用程序中安排定时任务或周期性的事件处理。`QTimer`的基本用法是首先创建一个`QTimer`实例,然后设置间隔时间(单位通常是毫秒),接着指定一个槽函数(slot function)作为定时触发后的回调,最后启动计时器。一旦达到设定的时间间隔,`QTimer`就会自动调用该槽函数。
以下是使用`QTimer`的基本步骤:
1. 创建`QTimer`对象:
```python
timer = QTimer(self) # self是你需要关联到的对象
```
2. 设置延迟时间和信号连接:
```python
# 设定每500毫秒执行一次
timer.setInterval(500)
# 当定时器到期时,执行某个槽函数(self是我想要绑定的对象)
timer.timeout.connect(self.my_slot_function)
```
3. 启动定时器:
```python
timer.start() # 开始计时
```
4. 取消或暂停定时器:
```python
timer.stop() # 停止计时器
timer.pause() # 暂停计时器
```
相关问题
pyqt5 qtimer
PyQt5中的QTimer类是一个用于发射信号的定时器。您可以使用它来执行周期性操作,例如更新UI或执行后台任务。下面是一个简单的示例,演示如何在每个一定时间间隔内更新一个标签的文本:
```python
from PyQt5.QtCore import QTimer
from PyQt5.QtWidgets import QApplication, QLabel, QWidget, QVBoxLayout
class MyWidget(QWidget):
def __init__(self):
super().__init__()
layout = QVBoxLayout()
self.label = QLabel("Hello world!")
layout.addWidget(self.label)
self.setLayout(layout)
self.timer = QTimer()
self.timer.timeout.connect(self.update_label)
self.timer.start(1000) # 1秒钟更新一次
def update_label(self):
self.label.setText("Updated at " + QDateTime.currentDateTime().toString())
if __name__ == '__main__':
app = QApplication([])
widget = MyWidget()
widget.show()
app.exec_()
```
在上面的示例中,我们创建了一个名为MyWidget的小部件,并在其中添加了一个标签。我们还创建了一个QTimer对象,并将其timeout信号连接到一个名为update_label的方法。在update_label方法中,我们更新了标签的文本。最后,我们启动了定时器,使其每秒钟发射一次timeout信号。
Pyqt5 qtimer
PyQt5 是一个用于创建图形用户界面的 Python 绑定和工具包,它使用 Qt 库。QTimer 是 PyQt5 中的一个类,用于提供定时器功能,它可以在指定的时间间隔后发射一个 timeout 信号。这个信号可以被用来触发一个槽函数,从而执行定时任务。
QTimer 可以设置为单次(只触发一次)或周期性(循环触发)。使用时,通常会将定时器的 timeout 信号连接到一个槽函数上。在槽函数中,你可以编写需要定时执行的代码。
下面是一个简单的例子,展示如何使用 PyQt5 的 QTimer:
```python
import sys
from PyQt5.QtCore import QTimer
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QVBoxLayout, QLCDNumber
class Window(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 创建 LCD 数码显示器
self.lcd = QLCDNumber(self)
# 创建按钮
self.button = QPushButton('启动定时器', self)
self.button.clicked.connect(self.startTimer)
# 创建垂直布局并添加控件
layout = QVBoxLayout()
layout.addWidget(self.lcd)
layout.addWidget(self.button)
self.setLayout(layout)
self.setWindowTitle('QTimer 示例')
self.show()
def startTimer(self):
# 创建定时器对象,设置定时器
self.timer = QTimer(self)
# 设置定时器在 1000 毫秒后触发 timeout 信号
self.timer.setInterval(1000)
# 将定时器的 timeout 信号连接到槽函数
self.timer.timeout.connect(self.updateLCD)
# 启动定时器
self.timer.start()
def updateLCD(self):
# 更新 LCD 显示的内容
self.lcd.display(self.lcd.value() + 1)
if __name__ == '__main__':
app = QApplication(sys.argv)
mainWin = Window()
sys.exit(app.exec_())
```
在这个例子中,每当定时器的 timeout 信号被触发时,updateLCD() 槽函数就会被调用,LCD 数码显示器上的数字就会递增。
阅读全文
相关推荐
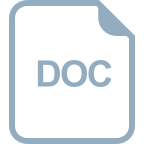
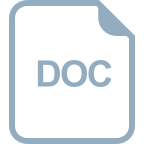
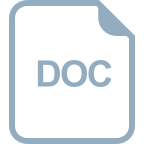






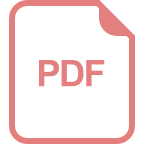






