贝叶斯优化VMD的matlab代码
时间: 2023-07-26 16:04:58 浏览: 165
以下是使用Matlab实现贝叶斯优化VMD的简单示例代码:
```matlab
% 导入信号处理工具箱
addpath('path_to_emd_library');
% 生成示例数据
t = linspace(0, 1, 1000);
x = chirp(t, 6, 1, 0.5);
% 定义VMD目标函数
fun = @(params) vmd_objective(params, x);
% 定义VMD目标函数
function obj_value = vmd_objective(params, x)
alpha = params(1);
tau = params(2);
emd = emd_mex();
imfs = emd.emd(x);
vmd_imfs = zeros(size(imfs));
for i = 1:length(imfs)
vmd_imfs(i, :) = imfs(i, :) * alpha^tau;
end
vmd_result = sum(vmd_imfs, 1);
% 计算目标函数值(示例中为信号的均方根误差)
obj_value = sqrt(sum((x - vmd_result).^2) / length(x));
end
% 定义参数空间边界
param_bounds = [0.1, 1.0; 0.1, 1.0];
% 定义贝叶斯优化选项
opts = optimoptions('bayesopt', 'MaxObjectiveEvaluations', 50);
% 运行贝叶斯优化
result = bayesopt(fun, param_bounds, 'Options', opts);
% 输出最优参数和目标函数值
best_params = result.XAtMinObjective;
best_obj = result.MinObjective;
disp("Best parameters found: ");
disp(best_params);
disp("Best objective value found: ");
disp(best_obj);
```
以上代码使用了Matlab内置函数`bayesopt`来进行贝叶斯优化。首先,导入信号处理工具箱(如emd_mex)以实现VMD函数。然后,生成示例数据。接下来,定义了一个VMD目标函数`vmd_objective`,该函数接受VMD参数和输入信号作为输入,并计算VMD结果与原始信号之间的均方根误差作为目标函数值。然后,定义了参数空间边界`param_bounds`,用于限制参数的搜索范围。接下来,使用`optimoptions`设置了贝叶斯优化的选项,例如最大目标函数评估次数。最后,通过调用`bayesopt`函数来运行贝叶斯优化,并将结果存储在`result`变量中。可以使用`result.XAtMinObjective`获取最优参数,`result.MinObjective`获取最优目标函数值。
请注意,实际应用中,VMD目标函数`vmd_objective`、参数空间边界`param_bounds`等需要根据具体问题进行定义和调整。另外,还可以通过设置其他选项来进一步调整贝叶斯优化的行为,例如采样方法、初始样本数量等。具体的实现方式可以根据实际需求进行调整。
阅读全文
相关推荐
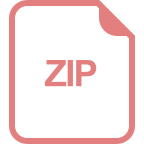
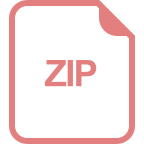

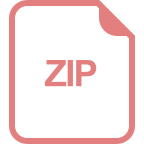
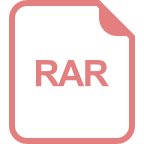
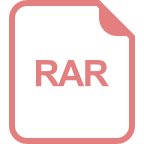
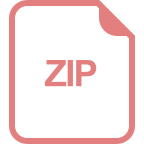
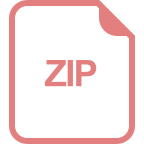
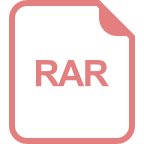
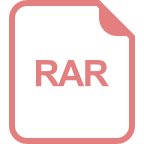
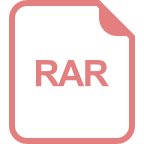
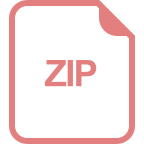
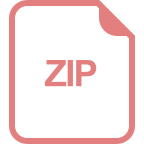
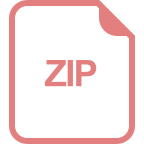
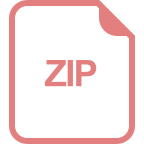
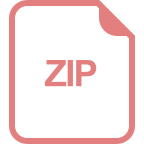
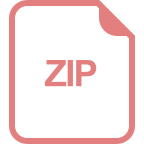