232串口通信C语言代码实现
时间: 2024-10-25 14:08:49 浏览: 31
在C语言中,通过串口通信通常使用POSIX标准提供的`stdio.h`库的`fopen()`函数打开串口设备,或者利用特定平台如Linux下的`termios.h`头文件进行控制。以下是一个基本的232串口通信的例子,假设我们使用Linux系统,并且已经包含了必要的头文件:
```c
#include <stdio.h>
#include <fcntl.h> // for open()
#include <sys/ioctl.h> // for ioctl() and TIOCM_DTR/TIOCM_RTS
#include <unistd.h> // for close()
#define PORT "/dev/ttyS0" // 修改为你系统的串口设备路径
#define BAUDRATE 9600 // 设定波特率
// 打开串口
int open_serial_port(int baudrate) {
int fd = open(PORT, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("Error opening serial port");
return -1;
}
// 设置波特率和其他选项
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, baudrate); // 设置发送速度
cfsetospeed(&options, baudrate); // 设置接收速度
options.c_cflag &= ~CSIZE; // 清除数据位设置
options.c_cflag |= CS8; // 设置为8位数据
options.c_cflag &= ~PARENB; // 不使用校验位
options.c_cflag &= ~CSTOPB; // 不使用停止位
options.c_cflag &= ~CRTSCTS; // 禁用硬件流控
options.c_iflag &= ~(IXON | IXOFF | IXANY); // 关闭软件流控
options.c_oflag &= ~OPOST; // 取消回显功能
options.c_lflag &= ~(ICANON | ECHO | ISIG); // 关闭终端处理
tcflush(fd, TCIFLUSH); // 清空输入缓冲区
if (tcsetattr(fd, TCSANOW, &options) == -1) {
perror("Error setting serial options");
close(fd);
return -1;
}
return fd;
}
// 发送数据
void send_data(int fd, char *data, size_t len) {
write(fd, data, len);
}
// 主函数示例
int main() {
int fd = open_serial_port(BAUDRATE);
if (fd == -1) {
perror("Failed to open serial port");
return 1;
}
char message[] = "Hello from C!";
send_data(fd, message, sizeof(message));
close(fd);
return 0;
}
```
阅读全文
相关推荐
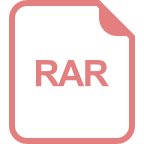
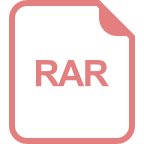
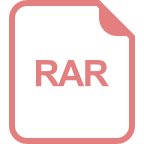
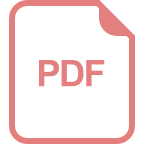
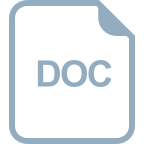
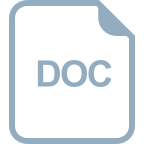
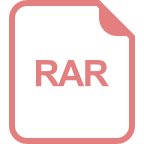
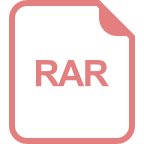
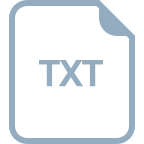
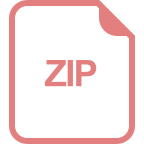
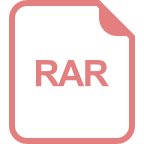
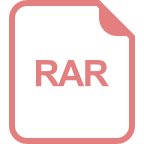
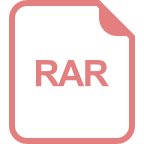
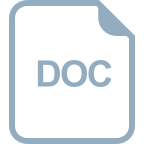
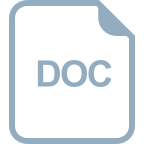