用java写一个文件上传方法
时间: 2023-05-18 16:04:54 浏览: 77
好的,以下是一个简单的Java文件上传方法的示例代码:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URL;
import java.net.URLConnection;
public class FileUploader {
public static void uploadFile(String urlString, String filePath) throws IOException {
URL url = new URL(urlString);
URLConnection conn = url.openConnection();
conn.setDoOutput(true);
conn.setDoInput(true);
conn.setRequestProperty("Content-Type", "multipart/form-data; boundary=---------------------------7d33a816d302b6");
OutputStream out = conn.getOutputStream();
File file = new File(filePath);
String fileName = file.getName();
String boundary = "-----------------------------7d33a816d302b6";
String header = "--" + boundary + "\r\nContent-Disposition: form-data; name=\"file\"; filename=\"" + fileName + "\"\r\nContent-Type: application/octet-stream\r\n\r\n";
out.write(header.getBytes());
InputStream in = FileUploader.class.getResourceAsStream(filePath);
byte[] buffer = new byte[1024];
int len;
while ((len = in.read(buffer)) != -1) {
out.write(buffer, 0, len);
}
in.close();
String footer = "\r\n--" + boundary + "--\r\n";
out.write(footer.getBytes());
out.flush();
out.close();
InputStream responseIn = conn.getInputStream();
byte[] responseBuffer = new byte[1024];
int responseLen;
while ((responseLen = responseIn.read(responseBuffer)) != -1) {
System.out.println(new String(responseBuffer, 0, responseLen));
}
responseIn.close();
}
}
```
你可以将上面的代码保存为一个名为 FileUploader.java 的文件,然后在你的 Java 项目中使用它来上传文件。调用 uploadFile 方法时,需要传入两个参数:文件上传的 URL 和要上传的文件的本地路径。例如:
```java
FileUploader.uploadFile("http://example.com/upload", "/path/to/file.txt");
```
这将会把本地的 /path/to/file.txt 文件上传到 http://example.com/upload。
相关推荐
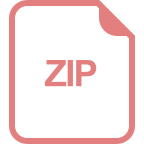
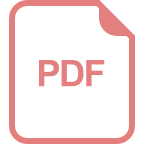
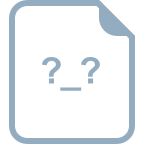














