小程序实现一个扭蛋机抽奖详细代码
时间: 2024-03-12 20:43:29 浏览: 20
好的,以下是一个简单的小程序实现扭蛋机抽奖的代码,具体实现细节可以根据自己的需要进行调整和修改:
1. HTML代码:
```
<!-- 扭蛋机界面 -->
<div class="gashapon">
<div class="gashapon-body">
<div class="gashapon-window">
<div class="gashapon-inner"></div>
</div>
<div class="gashapon-handle"></div>
</div>
<button class="gashapon-button">抽奖</button>
</div>
<!-- 中奖记录 -->
<div class="record">
<h3>中奖记录</h3>
<ul class="record-list"></ul>
</div>
```
2. CSS代码:
```
/* 扭蛋机样式 */
.gashapon {
width: 300px;
height: 400px;
position: relative;
margin: 50px auto;
}
.gashapon-body {
width: 100%;
height: 100%;
background: #eee;
border-radius: 20px;
position: relative;
overflow: hidden;
}
.gashapon-window {
width: 100%;
height: 70%;
background: #fff;
border-top-left-radius: 20px;
border-top-right-radius: 20px;
position: relative;
overflow: hidden;
box-shadow: 0 0 10px #444;
}
.gashapon-inner {
width: 100%;
height: 100%;
background: url("gashapon.png") no-repeat center;
background-size: contain;
position: absolute;
top: 0;
left: 0;
animation: gashapon-rotate 5s linear infinite;
transform-origin: center center;
}
.gashapon-handle {
width: 50px;
height: 50px;
background: #f00;
border-radius: 50%;
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
}
.gashapon-button {
display: block;
width: 100px;
height: 40px;
background: #f00;
color: #fff;
border: none;
border-radius: 20px;
position: absolute;
bottom: 0;
left: 50%;
transform: translateX(-50%);
margin-bottom: 20px;
cursor: pointer;
}
/* 中奖记录样式 */
.record {
width: 300px;
margin: 0 auto;
}
.record h3 {
text-align: center;
}
.record-list {
list-style: none;
padding: 0;
margin: 0;
}
.record-list li {
margin-bottom: 10px;
}
```
3. JavaScript代码:
```
// 定义奖品列表
var prizeList = [
{
name: "一等奖",
src: "prize1.png"
},
{
name: "二等奖",
src: "prize2.png"
},
{
name: "三等奖",
src: "prize3.png"
},
{
name: "幸运奖",
src: "prize4.png"
}
];
// 获取DOM元素
var gashapon = document.querySelector(".gashapon");
var gashaponInner = document.querySelector(".gashapon-inner");
var gashaponButton = document.querySelector(".gashapon-button");
var recordList = document.querySelector(".record-list");
// 定义变量
var isRunning = false; // 是否正在转动
var prizeIndex = -1; // 中奖奖品索引
// 抽奖函数
function lottery() {
if (isRunning) return; // 如果正在转动,则退出函数
isRunning = true; // 设置正在转动标志
// 随机生成一个奖品索引
prizeIndex = Math.floor(Math.random() * prizeList.length);
// 转动扭蛋机
gashaponInner.style.animationPlayState = "running";
// 2秒后停止转动
setTimeout(function() {
isRunning = false;
gashaponInner.style.animationPlayState = "paused";
showPrize(prizeList[prizeIndex]); // 显示中奖信息
}, 2000);
}
// 显示中奖信息
function showPrize(prize) {
// 创建中奖信息DOM元素
var li = document.createElement("li");
var img = document.createElement("img");
img.src = prize.src;
var span = document.createElement("span");
span.textContent = prize.name;
li.appendChild(img);
li.appendChild(span);
// 将中奖信息添加到记录列表中
recordList.appendChild(li);
}
// 绑定抽奖按钮的单击事件
gashaponButton.addEventListener("click", lottery);
```
以上就是一个简单的小程序实现扭蛋机抽奖的代码,可以根据自己的需要进行修改和完善。
相关推荐
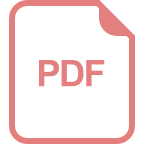
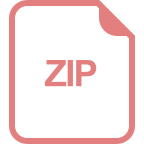












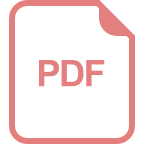