nodejs代码生成包含100个随机数的数组
时间: 2023-11-25 09:05:02 浏览: 71
好的,以下是生成包含100个随机数的数组的 nodejs 代码示例:
```javascript
const arr = Array.from({ length: 100 }, () => Math.floor(Math.random() * 100));
console.log(arr);
```
希望可以帮到您!如果还有其他问题,请随时问我。
相关问题
mysql 单选 多选 统计_随机生成一份试卷,试卷的种类分为单选、多选、判断三种题型。nodejs6.0 mysql...
可以按照以下步骤实现:
1. 创建一个试卷模板,包含不同种类的题目数量、每个题目的分值和难度系数等信息。
2. 随机生成试卷,可以使用 Node.js 内置的 `Math.random()` 函数生成随机数,然后根据试卷模板中的信息,从数据库中随机抽取题目。
3. 统计试卷成绩,把用户的答案与正确答案进行比对,计算分值。
下面是一个示例代码:
```
const mysql = require('mysql');
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'test'
});
// 试卷模板
const paperTemplate = {
singleChoiceCount: 5,
multipleChoiceCount: 5,
judgementCount: 5,
singleChoiceScore: 2,
multipleChoiceScore: 4,
judgementScore: 2,
singleChoiceDifficulty: [1, 2, 3, 4, 5],
multipleChoiceDifficulty: [1, 2, 3, 4, 5],
judgementDifficulty: [1, 2, 3, 4, 5]
};
// 随机生成试卷
function generatePaper() {
const paper = {
singleChoice: [],
multipleChoice: [],
judgement: []
};
// 单选题
for (let i = 0; i < paperTemplate.singleChoiceCount; i++) {
const difficulty = paperTemplate.singleChoiceDifficulty[Math.floor(Math.random() * paperTemplate.singleChoiceDifficulty.length)];
connection.query(`SELECT * FROM questions WHERE type='single' AND difficulty=${difficulty} ORDER BY RAND() LIMIT 1`, (error, results, fields) => {
if (error) throw error;
paper.singleChoice.push(results[0]);
});
}
// 多选题
for (let i = 0; i < paperTemplate.multipleChoiceCount; i++) {
const difficulty = paperTemplate.multipleChoiceDifficulty[Math.floor(Math.random() * paperTemplate.multipleChoiceDifficulty.length)];
connection.query(`SELECT * FROM questions WHERE type='multiple' AND difficulty=${difficulty} ORDER BY RAND() LIMIT 1`, (error, results, fields) => {
if (error) throw error;
paper.multipleChoice.push(results[0]);
});
}
// 判断题
for (let i = 0; i < paperTemplate.judgementCount; i++) {
const difficulty = paperTemplate.judgementDifficulty[Math.floor(Math.random() * paperTemplate.judgementDifficulty.length)];
connection.query(`SELECT * FROM questions WHERE type='judgement' AND difficulty=${difficulty} ORDER BY RAND() LIMIT 1`, (error, results, fields) => {
if (error) throw error;
paper.judgement.push(results[0]);
});
}
return paper;
}
// 统计试卷成绩
function calculateScore(paper, answers) {
let score = 0;
// 单选题
for (let i = 0; i < paper.singleChoice.length; i++) {
if (answers[i] === paper.singleChoice[i].answer) {
score += paperTemplate.singleChoiceScore;
}
}
// 多选题
for (let i = 0; i < paper.multipleChoice.length; i++) {
if (answers[i + paper.singleChoice.length] === paper.multipleChoice[i].answer) {
score += paperTemplate.multipleChoiceScore;
}
}
// 判断题
for (let i = 0; i < paper.judgement.length; i++) {
if (answers[i + paper.singleChoice.length + paper.multipleChoice.length] === paper.judgement[i].answer) {
score += paperTemplate.judgementScore;
}
}
return score;
}
// 示例用法
const paper = generatePaper();
const answers = ['A', ['A', 'B', 'C'], 'T', 'F', ['A', 'B'], 'F', 'T', ['C', 'D'], 'A', 'B'];
const score = calculateScore(paper, answers);
console.log(score);
```
上述示例代码中,`generatePaper()` 函数会根据试卷模板从数据库中随机抽取题目,并返回一个包含所有题目的试卷对象。`calculateScore()` 函数接收一个试卷对象和用户的答案,返回用户的得分。示例用法中,我们手动构造了一个假的答案数组,然后计算得分并输出。实际使用时,应该由用户输入答案。
阅读全文
相关推荐
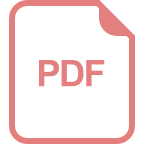
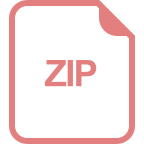
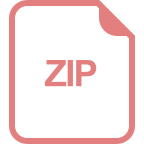
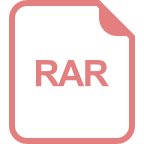
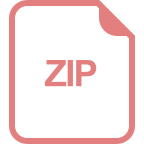
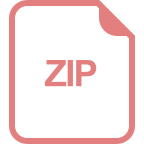
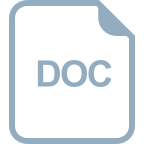
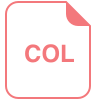
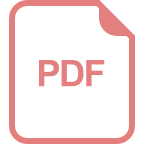
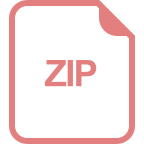
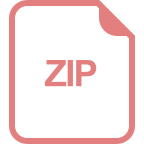
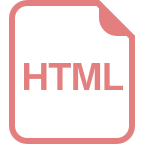