java数据导入excel代码
时间: 2024-05-03 15:21:06 浏览: 63
可以使用Apache POI库来实现Java数据导入Excel功能。具体实现代码可以参考以下示例:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellType;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelWriter {
public static void writeData(List<String[]> data, String filePath) throws IOException {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet();
int rowCount = 0;
for (String[] rowData : data) {
Row row = sheet.createRow(rowCount++);
int columnCount = 0;
for (String value : rowData) {
Cell cell = row.createCell(columnCount++);
if (value instanceof String) {
cell.setCellValue((String) value);
} else if (value instanceof Double) {
cell.setCellValue((Double) value);
} else if (value instanceof Integer) {
cell.setCellValue((Integer) value);
}
}
}
FileOutputStream outputStream = new FileOutputStream(filePath);
workbook.write(outputStream);
outputStream.close();
workbook.close();
}
public static void main(String[] args) throws IOException {
List<String[]> data = getData();
writeData(data, "output.xlsx");
}
private static List<String[]> getData() {
// TODO: 实现获取数据的方法
}
}
```
此代码演示了如何使用Apache POI库创建一个Excel工作簿,并将Java数据写入Excel文件。如果需要导入已有的Excel文件,可以使用相似的代码读取Excel文件中的数据。
阅读全文
相关推荐
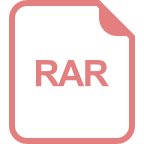
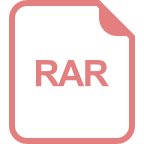
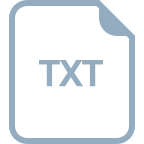
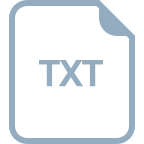
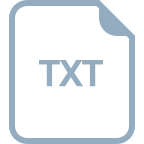
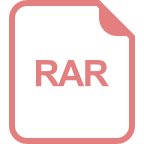
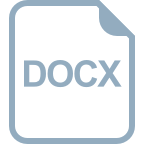
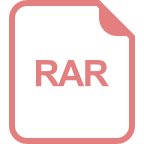
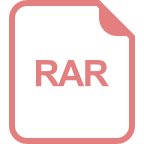
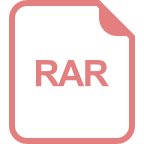
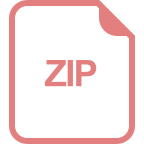
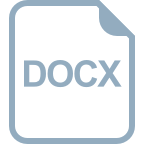
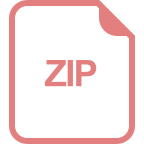
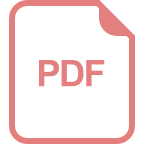
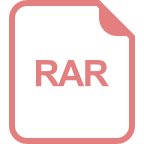
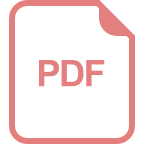