spring boot 怎么新建一个zip包
时间: 2024-02-19 13:34:08 浏览: 19
要在Spring Boot中创建Zip包,可以使用以下步骤:
1. 在pom.xml文件中添加以下依赖项:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-compress</artifactId>
<version>1.20</version>
</dependency>
```
2. 创建一个类来处理Zip文件的创建和压缩:
```
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
import org.springframework.stereotype.Service;
@Service
public class ZipService {
public void createZipFile() throws IOException {
String filename = "example.zip";
File file = new File(filename);
FileOutputStream fos = new FileOutputStream(file);
ZipOutputStream zos = new ZipOutputStream(fos);
// add files to the zip file
addToZipFile("file1.txt", zos);
addToZipFile("file2.txt", zos);
zos.close();
fos.close();
}
private void addToZipFile(String fileName, ZipOutputStream zos) throws IOException {
File file = new File(fileName);
ZipEntry zipEntry = new ZipEntry(file.getName());
zos.putNextEntry(zipEntry);
// write the file to the zip output stream
// ...
zos.closeEntry();
}
}
```
3. 在Spring Boot应用程序中使用ZipService类:
```
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ConfigurableApplicationContext;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) throws Exception {
ConfigurableApplicationContext context = SpringApplication.run(MyApplication.class, args);
ZipService zipService = context.getBean(ZipService.class);
zipService.createZipFile();
}
}
```
这将创建一个名为example.zip的Zip文件,其中包含名为file1.txt和file2.txt的两个文件。您可以根据需要更改文件名和文件内容。
相关推荐
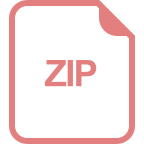
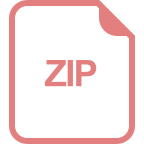
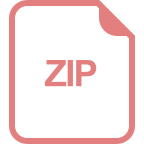














