用python的pygame第三方库写一个井子棋游戏
时间: 2023-06-10 20:07:51 浏览: 114
好的,下面是井子棋游戏的代码:
```python
import pygame
# 初始化pygame
pygame.init()
# 设置窗口大小
WINDOW_SIZE = (500, 500)
# 设置颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
# 设置字体和字号
FONT = pygame.font.SysFont('Arial', 50)
# 设置棋盘的大小和位置
BOARD_SIZE = (300, 300)
BOARD_POS = ((WINDOW_SIZE[0] - BOARD_SIZE[0]) // 2, (WINDOW_SIZE[1] - BOARD_SIZE[1]) // 2)
# 设置每个格子的大小
CELL_SIZE = 100
# 定义棋盘的类
class Board:
def __init__(self):
self.cells = [[None for _ in range(3)] for _ in range(3)]
def reset(self):
self.cells = [[None for _ in range(3)] for _ in range(3)]
def draw(self, surface):
for i in range(3):
for j in range(3):
# 绘制格子
pygame.draw.rect(surface, BLACK, (BOARD_POS[0] + j * CELL_SIZE, BOARD_POS[1] + i * CELL_SIZE, CELL_SIZE, CELL_SIZE), 2)
# 绘制棋子
if self.cells[i][j] == 'X':
pygame.draw.line(surface, BLACK, (BOARD_POS[0] + j * CELL_SIZE + 10, BOARD_POS[1] + i * CELL_SIZE + 10), (BOARD_POS[0] + (j + 1) * CELL_SIZE - 10, BOARD_POS[1] + (i + 1) * CELL_SIZE - 10), 2)
pygame.draw.line(surface, BLACK, (BOARD_POS[0] + j * CELL_SIZE + 10, BOARD_POS[1] + (i + 1) * CELL_SIZE - 10), (BOARD_POS[0] + (j + 1) * CELL_SIZE - 10, BOARD_POS[1] + i * CELL_SIZE + 10), 2)
elif self.cells[i][j] == 'O':
pygame.draw.circle(surface, BLACK, (BOARD_POS[0] + j * CELL_SIZE + CELL_SIZE // 2, BOARD_POS[1] + i * CELL_SIZE + CELL_SIZE // 2), CELL_SIZE // 2 - 10, 2)
def get_cell(self, row, col):
return self.cells[row][col]
def set_cell(self, row, col, value):
self.cells[row][col] = value
def is_full(self):
for i in range(3):
for j in range(3):
if self.cells[i][j] is None:
return False
return True
def check_win(self):
# 检查行
for i in range(3):
if self.cells[i][0] == self.cells[i][1] == self.cells[i][2] and self.cells[i][0] is not None:
return self.cells[i][0]
# 检查列
for j in range(3):
if self.cells[0][j] == self.cells[1][j] == self.cells[2][j] and self.cells[0][j] is not None:
return self.cells[0][j]
# 检查对角线
if self.cells[0][0] == self.cells[1][1] == self.cells[2][2] and self.cells[0][0] is not None:
return self.cells[0][0]
if self.cells[0][2] == self.cells[1][1] == self.cells[2][0] and self.cells[0][2] is not None:
return self.cells[0][2]
return None
# 创建窗口
screen = pygame.display.set_mode(WINDOW_SIZE)
# 设置窗口标题
pygame.display.set_caption("井子棋游戏")
# 初始化游戏
board = Board()
turn = 'X'
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
# 获取鼠标点击的位置
pos = pygame.mouse.get_pos()
# 判断是否在棋盘内
if BOARD_POS[0] <= pos[0] < BOARD_POS[0] + BOARD_SIZE[0] and BOARD_POS[1] <= pos[1] < BOARD_POS[1] + BOARD_SIZE[1]:
# 获取所在的行和列
row = (pos[1] - BOARD_POS[1]) // CELL_SIZE
col = (pos[0] - BOARD_POS[0]) // CELL_SIZE
# 判断该位置是否已经有棋子
if board.get_cell(row, col) is None:
# 设置该位置的棋子
board.set_cell(row, col, turn)
# 切换玩家
turn = 'X' if turn == 'O' else 'O'
# 绘制背景
screen.fill(WHITE)
# 绘制棋盘
board.draw(screen)
# 绘制玩家信息
text = FONT.render("玩家 " + turn + " 走", True, BLACK)
screen.blit(text, (10, 10))
# 检查游戏是否结束
winner = board.check_win()
if winner is not None:
text = FONT.render("玩家 " + winner + " 获胜", True, BLACK)
screen.blit(text, ((WINDOW_SIZE[0] - text.get_width()) // 2, (WINDOW_SIZE[1] - text.get_height()) // 2))
elif board.is_full():
text = FONT.render("游戏结束,平局", True, BLACK)
screen.blit(text, ((WINDOW_SIZE[0] - text.get_width()) // 2, (WINDOW_SIZE[1] - text.get_height()) // 2))
# 更新屏幕
pygame.display.flip()
# 退出pygame
pygame.quit()
```
运行代码后,就可以玩井子棋游戏了。玩家可以用鼠标点击棋盘上的格子来落子,游戏结束时会显示获胜者或平局。
阅读全文
相关推荐

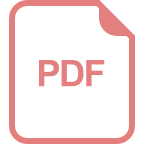

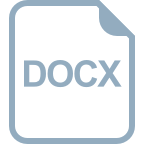
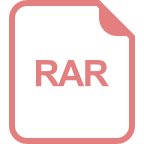
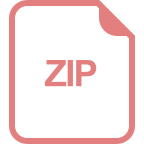
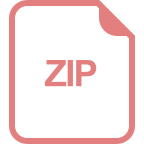
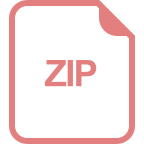
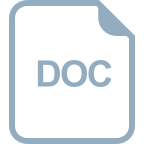
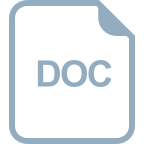
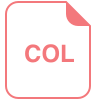
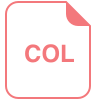
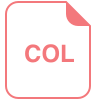

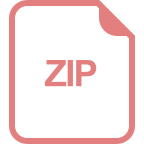
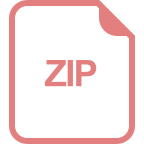