java treemap 如何使用comparable
时间: 2023-07-20 13:15:54 浏览: 56
Java TreeMap可以使用Comparable接口来对其键进行排序。要使用Comparable接口,您需要在键类中实现compareTo()方法。该方法应该返回一个整数值,表示该对象应该位于另一个对象的前面、相同或后面。以下是一个示例:
```java
import java.util.TreeMap;
public class Main {
public static void main(String[] args) {
TreeMap<Person, String> map = new TreeMap<Person, String>();
map.put(new Person("Alice", 25), "Alice's value");
map.put(new Person("Bob", 30), "Bob's value");
map.put(new Person("Charlie", 20), "Charlie's value");
for (Person key : map.keySet()) {
System.out.println(key + " => " + map.get(key));
}
}
}
class Person implements Comparable<Person> {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public int compareTo(Person other) {
if (this.age < other.age) {
return -1;
} else if (this.age > other.age) {
return 1;
} else {
return 0;
}
}
public String toString() {
return name + " (" + age + ")";
}
}
```
在上面的示例中,我们使用Person类作为TreeMap的键,并在Person类中实现了Comparable接口。我们实现了compareTo()方法,该方法比较两个Person对象的年龄,并返回一个整数值,指示哪个对象应该排在前面、相同或后面。当我们遍历TreeMap时,它将按照Person对象的年龄进行排序输出。
相关推荐
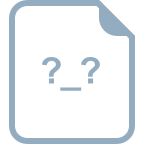














