采用C++编制曲线要素计算的命令行程序,实现: (1) JD.txt文件的读取 (2) 曲线要素计算。 (3) 曲线要素计算结果输出 (4) 给定线路任意里程计算平面坐标,并输出逐桩坐标表
时间: 2024-10-14 12:15:20 浏览: 35
要编写一个使用C++的命令行程序来实现上述功能,你需要做以下步骤:
1. **JD.txt文件读取**:
首先,你需要使用`fstream`库来处理文本文件。创建一个名为`readJDFile.cpp`的文件,然后添加以下代码来打开并读取JD.txt文件:
```cpp
#include <fstream>
#include <iostream>
std::vector<std::pair<double, double>> readJDFile(const std::string& filename) {
std::ifstream file(filename);
if (!file.is_open()) {
std::cerr << "Error opening file " << filename << std::endl;
return {};
}
std::vector<std::pair<double, double>> data;
std::string line;
while (std::getline(file, line)) {
std::istringstream iss(line);
if (!(iss >> data.back().first >> data.back().second)) {
// Handle error or skip invalid line
continue;
}
}
file.close();
return data;
}
```
2. **曲线要素计算**:
这部分取决于你的曲线要素的具体算法,例如如果涉及到笛卡尔坐标系下的曲线插值或计算弧长等。这将需要数学运算和可能的数据结构。
3. **计算结果输出**:
将计算出的结果保存在一个数据结构(如向量、列表或矩阵)中,然后打印到控制台或写入另一个文件。例如:
```cpp
void printCurveElements(const std::vector<std::pair<double, double>>& results) {
for (const auto& result : results) {
std::cout << "Element Result: (" << result.first << ", " << result.second << ")" << std::endl;
}
}
```
4. **里程到坐标计算**:
如果有一个函数可以接受里程和曲线信息来计算对应点的坐标,你可以像下面这样设计:
```cpp
struct CurveInfo {
// ... curve properties (e.g., centerline coordinates, radius)
};
std::pair<double, double> calculateCoordAtDistance(double distance, const CurveInfo& info) {
// ... implement your calculation here
}
double getCoordFromMileage(double mileage, const std::vector<CurveInfo>& curves) {
// Iterate over the curves to find the correct one and call the calculator
for (const auto& curve : curves) {
double totalLength = /* previously computed total length */;
if (mileage <= totalLength) {
double elementDist = mileage / totalLength; // normalized distance within current curve
// Calculate and return the coordinate
return calculateCoordAtDistance(elementDist, curve);
}
}
throw std::runtime_error("Invalid milestone");
}
```
最后,在主函数`main()`中整合所有操作:
```cpp
int main(int argc, char* argv[]) {
try {
std::vector<std::pair<double, double>> jdData = readJDFile("JD.txt");
// Perform calculations on jdData...
printCurveElements(/* results */);
double givenMilestone = 100.0; // Example milestone
std::pair<double, double> coord = getCoordFromMileage(givenMilestone, /* curves data */);
std::cout << "Coordinate at milestone " << givenMilestone << ": (" << coord.first << ", " << coord.second << ")" << std::endl;
// Output coordinates table (optional)
// WriteCoordTable(coordList); // Implement this function to write to a CSV file
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
return 1;
}
return 0;
}
```
记得编译这个程序时链接`cstdlib`和`cstdio`库,并提供JD.txt文件作为输入。
阅读全文
相关推荐
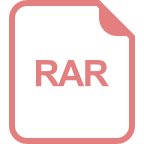
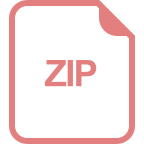
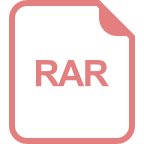
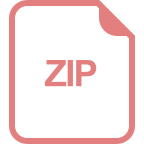
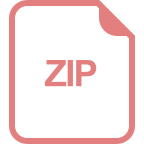
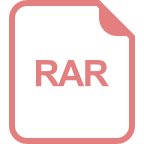
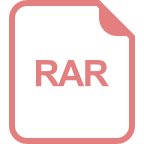
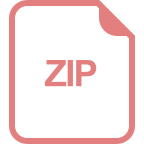
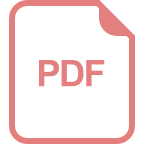
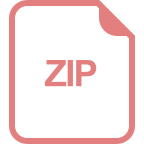
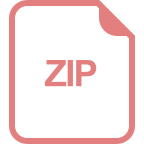
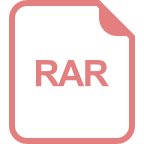
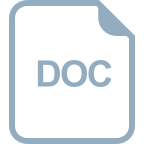
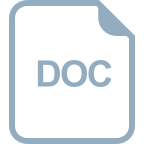
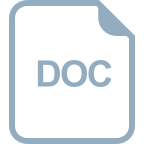
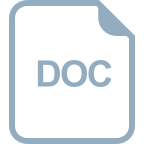
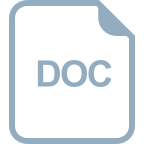
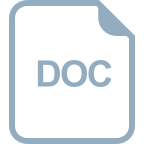