使用tio-websocket-spring-boot-starter组件实现客户端消息的接收和发送
时间: 2023-07-30 09:04:03 浏览: 84
好的,我可以回答您的问题。
tio-websocket-spring-boot-starter是一个基于t-io框架的WebSocket组件,可以在Spring Boot应用中方便地实现WebSocket服务。要使用该组件实现客户端消息的接收和发送,您可以按照以下步骤进行:
1. 在Spring Boot应用中添加tio-websocket-spring-boot-starter依赖。
2. 在应用中定义WebSocket的处理器类,并使用@TioWebSocket注解标注该类。
3. 在处理器类中实现onTextMessage()方法,用于处理客户端发送的文本消息。
4. 在处理器类中使用Tio.sendToAll()方法发送消息给所有已连接的客户端。
5. 在客户端中使用WebSocket API连接WebSocket服务,并使用send()方法发送消息。
6. 在客户端中使用onmessage()方法接收服务端发送的消息。
以上就是使用tio-websocket-spring-boot-starter组件实现客户端消息的接收和发送的基本步骤。具体实现细节可以参考该组件的官方文档和示例代码。
相关问题
使用tio-websocket-spring-boot-starter组件实现客户端消息的接收和发送,代码示例
好的,下面是一个简单的使用tio-websocket-spring-boot-starter组件实现客户端消息的接收和发送的代码示例:
WebSocket处理器类:
```java
@TioWebSocket(path = "/websocket")
public class MyWebSocketHandler {
@OnTextMessage
public void onTextMessage(ChannelContext ctx, String message) {
// 处理客户端发送的文本消息
Tio.sendToAll(ctx.getGroupContext(), WsResponse.fromText(message, "utf-8"));
}
}
```
启动类:
```java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean
public ServerEndpointExporter serverEndpointExporter() {
return new ServerEndpointExporter();
}
}
```
客户端代码:
```javascript
var ws = new WebSocket("ws://localhost:8080/websocket");
ws.onopen = function() {
// 连接成功后发送消息
ws.send("Hello, WebSocket!");
};
ws.onmessage = function(evt) {
// 接收服务端发送的消息
var message = evt.data;
console.log("Received message: " + message);
};
ws.onclose = function() {
console.log("WebSocket closed.");
};
```
以上示例中,WebSocket处理器类使用了@TioWebSocket注解标注,并且实现了onTextMessage()方法来处理客户端发送的文本消息。在onTextMessage()方法中,使用Tio.sendToAll()方法将接收到的消息发送给所有已连接的客户端。
启动类中使用了ServerEndpointExporter类,这是Spring Boot集成WebSocket的必要配置。客户端代码中使用WebSocket API连接WebSocket服务,并在onopen()方法中发送消息,在onmessage()方法中接收服务端发送的消息。
这只是一个简单的示例,实际使用中需要根据具体需求进行修改和扩展。
使用tio-websocket-spring-boot-starter组件实现服务端消息的接收和发送
好的,这是一个关于使用 tio-websocket-spring-boot-starter 组件实现服务端消息的接收和发送的问题。
首先,你需要在你的 Spring Boot 项目中添加 tio-websocket-spring-boot-starter 组件的依赖。可以在 Maven 或 Gradle 中添加以下依赖:
```xml
<dependency>
<groupId>com.github.t-io</groupId>
<artifactId>tio-websocket-spring-boot-starter</artifactId>
<version>2.7.0.v20190920-RELEASE</version>
</dependency>
```
或
```groovy
implementation 'com.github.t-io:tio-websocket-spring-boot-starter:2.7.0.v20190920-RELEASE'
```
接下来,你需要创建一个 WebSocket 服务端类,并使用 `@TioWebSocketServer` 注解将其标记为 Tio WebSocket 服务器。例如:
```java
import org.springframework.stereotype.Component;
import org.tio.server.ServerGroupContext;
import org.tio.websocket.server.WsServerConfig;
import org.tio.websocket.server.WsServerStarter;
import org.tio.websocket.server.handler.IWsMsgHandler;
@Component
@TioWebSocketServer
public class MyWebSocketServer {
private WsServerStarter wsServerStarter;
public MyWebSocketServer(IWsMsgHandler wsMsgHandler) {
WsServerConfig wsServerConfig = new WsServerConfig(8080);
ServerGroupContext serverGroupContext = new ServerGroupContext("my-websocket-server", wsMsgHandler);
wsServerStarter = new WsServerStarter(wsServerConfig, serverGroupContext);
}
public void start() throws Exception {
wsServerStarter.start();
}
public void stop() throws Exception {
wsServerStarter.stop();
}
}
```
在上面的代码中,我们创建了一个名为 `MyWebSocketServer` 的 Spring 组件,并使用 `@TioWebSocketServer` 注解将其标记为 Tio WebSocket 服务器。`MyWebSocketServer` 类中的构造函数中注入了一个 `IWsMsgHandler` 类型的参数,这个参数将在后面用来处理 WebSocket 消息。
然后,我们创建了一个 `WsServerConfig` 对象,指定了 WebSocket 服务器的端口号。接下来,我们创建了一个 `ServerGroupContext` 对象,并将 `WsMsgHandler` 对象传递给它,用来处理 WebSocket 消息。最后,我们创建了一个 `WsServerStarter` 对象,并传递了上面创建的 `WsServerConfig` 和 `ServerGroupContext` 对象,用来启动 WebSocket 服务器。
接下来,你需要创建一个 `IWsMsgHandler` 实现类,用来处理 WebSocket 消息。例如:
```java
import org.springframework.stereotype.Component;
import org.tio.websocket.common.WsRequest;
import org.tio.websocket.common.WsResponse;
import org.tio.websocket.server.handler.IWsMsgHandler;
@Component
public class MyWsMsgHandler implements IWsMsgHandler {
@Override
public Object onText(WsRequest wsRequest, String s) throws Exception {
System.out.println("Received message: " + s);
WsResponse wsResponse = WsResponse.fromText("Hello, " + s, "utf-8");
return wsResponse;
}
@Override
public Object onBytes(WsRequest wsRequest, byte[] bytes) throws Exception {
return null;
}
@Override
public void onAfterHandshaked(WsRequest wsRequest, WsResponse wsResponse) throws Exception {
System.out.println("WebSocket handshake succeeded");
}
@Override
public void onAfterClose(WsRequest wsRequest, byte[] bytes) throws Exception {
System.out.println("WebSocket closed");
}
}
```
在上面的代码中,我们创建了一个名为 `MyWsMsgHandler` 的 Spring 组件,并实现了 `IWsMsgHandler` 接口。在 `onText` 方法中,我们将接收到的 WebSocket 文本消息进行处理,并返回一个包含响应消息的 `WsResponse` 对象。在 `onAfterHandshaked` 方法中,我们在 WebSocket 握手成功之后进行处理。在 `onAfterClose` 方法中,我们在 WebSocket 连接关闭之后进行处理。
最后,我们可以在 Spring Boot 应用程序中使用 `MyWebSocketServer` 组件来启动 WebSocket 服务器。例如:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ConfigurableApplicationContext;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) throws Exception {
ConfigurableApplicationContext context = SpringApplication.run(MyApplication.class, args);
MyWebSocketServer myWebSocketServer = context.getBean(MyWebSocketServer.class);
myWebSocketServer.start();
}
}
```
在上面的代码中,我们创建了一个 Spring Boot 应用程序,并在 `main` 方法中启动了 WebSocket 服务器。我们首先通过 `SpringApplication.run` 方法启动 Spring Boot 应用程序,并获取了一个 `ConfigurableApplicationContext` 对象。然后,我们通过 `context.getBean` 方法获取了刚才创建的 `MyWebSocketServer` 组件,并调用了 `start` 方法来启动 WebSocket 服务器。
现在,你已经成功地使用 tio-websocket-spring-boot-starter 组件实现了服务端消息的接收和发送。
相关推荐
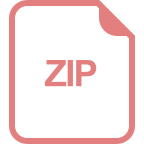
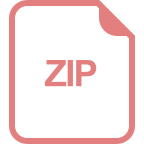
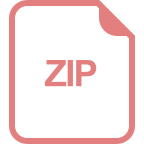












