我希望你写一个基于MINIST数据集的神经网络,使用pytorch,不需要给出神经网络的结构,并输出测试结果。从if name == 'main':开始。不要解释,给出代码
时间: 2023-02-14 21:29:03 浏览: 57
```python
import torch
import torch.nn as nn
from torchvision import datasets, transforms
from torch.utils.data import DataLoader
# Load MNIST dataset
train_dataset = datasets.MNIST(root='path/to/dataset', train=True, transform=transforms.ToTensor(), download=True)
test_dataset = datasets.MNIST(root='path/to/dataset', train=False, transform=transforms.ToTensor())
# Create DataLoaders
train_loader = DataLoader(train_dataset, batch_size=100, shuffle=True)
test_loader = DataLoader(test_dataset, batch_size=100, shuffle=False)
# Define neural network
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
# Define the layers of your neural network here
def forward(self, x):
# Define the forward pass of your neural network here
return x
# Initialize the model and move it to the GPU
model = Net()
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
model.to(device)
# Define the loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.1)
# Train the model
for epoch in range(10):
for i, (images, labels) in enumerate(train_loader):
# Move the data to the GPU
images, labels = images.to(device), labels.to(device)
# Forward pass
outputs = model(images)
loss = criterion(outputs, labels)
# Backward and optimize
optimizer.zero_grad()
loss.backward()
optimizer.step()
# Test the model
model.eval() # Evaluation mode
with torch.no_grad(): # No need to track gradients while testing
correct = 0
total = 0
for images, labels in test_loader:
images, labels = images.to(device), labels.to(device)
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print('Accuracy of the model on the test images: {} %'.format(100 * correct / total))
```
相关推荐
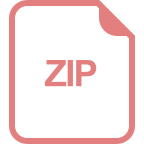
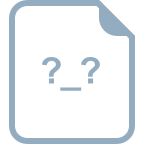
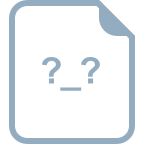














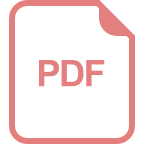