改进的免疫算法在物流中心选址问题代码
时间: 2024-03-15 17:45:32 浏览: 49
以下是改进的免疫粒子群算法在物流中心选址问题的Python代码示例。这里使用的是基于Python的PyGMO优化库。
```
import pygmo as pg
import numpy as np
# 定义问题类
class LogisticsCenterProblem:
def __init__(self, num_centers, num_customers, max_distance):
self.num_centers = num_centers
self.num_customers = num_customers
self.max_distance = max_distance
def fitness(self, x):
# 计算适应度函数值
total_distance = 0.0
for i in range(self.num_customers):
min_distance = float('inf')
for j in range(self.num_centers):
distance = np.linalg.norm(x[j] - x[self.num_centers+i])
if distance < min_distance:
min_distance = distance
total_distance += min_distance
return [total_distance]
def get_bounds(self):
# 定义决策变量的取值范围
return (np.zeros(self.num_centers*2), np.ones(self.num_centers*2)*self.max_distance)
def get_name(self):
# 定义问题名称
return "Logistics Center Location Problem"
# 定义算法类
class ImprovedImmunePSO:
def __init__(self, num_centers, num_customers, max_distance):
self.num_centers = num_centers
self.num_customers = num_customers
self.max_distance = max_distance
def optimize(self):
# 定义问题
problem = pg.problem(LogisticsCenterProblem(self.num_centers, self.num_customers, self.max_distance))
# 定义算法
algorithm = pg.algorithm(pg.immune_pso(gen=100, omega=0.5, eta1=1, eta2=1, m=20, n=20, variant=1))
# 定义种群
population = pg.population(problem, size=50)
# 进行优化
population = algorithm.evolve(population)
# 返回最优解
best_fitness = population.champion_f[0]
best_solution = population.champion_x[:self.num_centers]
return (best_fitness, best_solution)
# 测试算法
if __name__ == '__main__':
num_centers = 5
num_customers = 20
max_distance = 100.0
optimizer = ImprovedImmunePSO(num_centers, num_customers, max_distance)
best_fitness, best_solution = optimizer.optimize()
print("Best Fitness:", best_fitness)
print("Best Solution:", best_solution)
```
需要注意的是,这里的代码仅供参考,具体实现方式可能因问题的不同而有所差异。如果您有任何问题或疑问,欢迎随时向我提问。
阅读全文
相关推荐
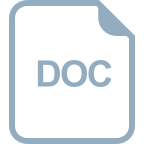
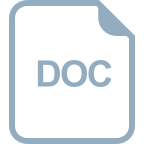

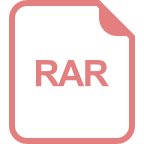
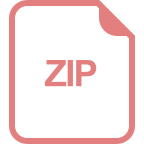
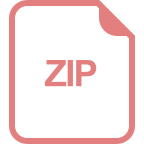
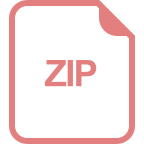
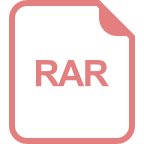
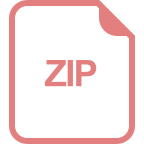
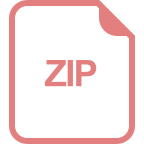
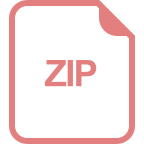
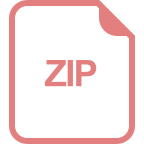
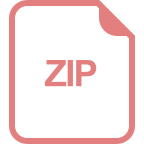
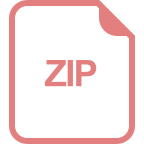
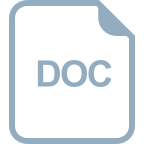