1.编写“串块链存储表示头文件.h”,其中包含:初始化空串、生成一个其值等于串常量的串、复制串、判空串、串比较、串联接、取子串、求串长、串的模式匹配、显示串等基本操作。 2.编写“串块链主程序.cpp”,调用以上函数。要求有运行界面
时间: 2024-10-14 21:07:08 浏览: 75
首先,我们来创建一个名为`StringList.h`的头文件,用于定义字符串链表的基本操作:
```cpp
#ifndef STRINGLIST_H
#define STRINGLIST_H
#include <iostream>
#include <string>
class StringListNode {
public:
std::string data;
StringListNode* next;
// 初始化为空串
StringListNode() : data(""), next(nullptr) {}
};
class StringList {
private:
StringListNode* head;
public:
// 初始化空串链表
void initEmpty();
// 生成并返回新节点,其值等于给定的串常量
StringListNode* createFromConstant(const std::string& str);
// 复制一个已有串到新节点
StringListNode* copyString(const std::string& src);
// 判断链表是否为空
bool isEmpty() const;
// 比较两个串
int compareStrings(const StringListNode* node1, const StringListNode* node2);
// 串联两个链表
StringListNode* concatenate(StringListNode* list1, StringListNode* list2);
// 取子串
StringListNode* substring(const StringListNode* start, int length);
// 求串长
int getLength(const StringListNode* node);
// 模式匹配
bool patternMatch(const StringListNode* pattern, const StringListNode* text);
// 显示串
void display(const StringListNode* node);
};
#endif // STRINGLIST_H
```
接下来,在`StringList.cpp`中实现这些操作:
```cpp
#include "StringList.h"
// 实现头文件中的函数
void StringList::initEmpty() {
head = nullptr;
}
StringListNode* StringList::createFromConstant(const std::string& str) {
StringListNode* newNode = new StringListNode();
newNode->data = str;
newNode->next = nullptr;
return newNode;
}
// ... 其他函数的实现 ...
void mainProgram() {
// 创建一个StringList实例
StringList stringList;
// 调用上述功能,并展示运行界面,例如用户输入和输出
stringList.initEmpty();
// 用户交互部分
std::cout << "请输入命令(例如 'create test', 'copy', 'compare', 'concatenate', 等): ";
std::string command;
while (std::cin >> command) {
if (command == "create") {
std::string constantStr;
std::cout << "请输入常量字符串: ";
std::getline(std::cin, constantStr);
stringList.createFromConstant(constantStr);
}
else if (command == "copy") { /* ... */ }
else if (command == "compare") { /* ... */ }
// ... 其他命令处理
// 增加循环直到用户结束或错误指令
}
// 结束时清空链表或显示结果
stringList.display(stringList.head);
}
```
这个`mainProgram`函数提供了一个基础的运行界面,用户可以输入各种命令来操作字符串链表。具体的每个命令的实现细节你需要按照需求来编写。
阅读全文
相关推荐
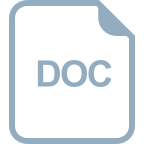
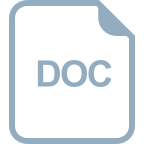
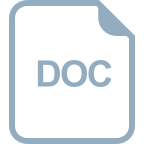
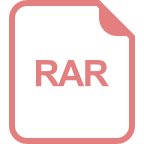
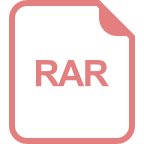
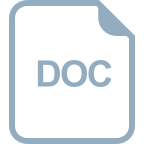
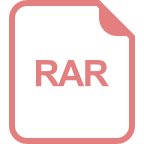
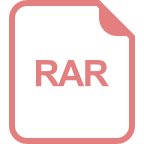
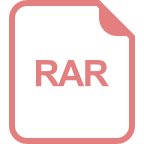
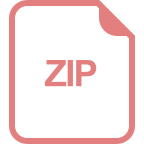
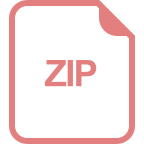
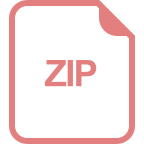
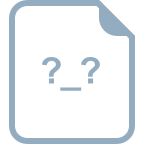
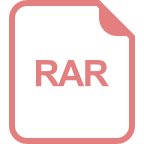
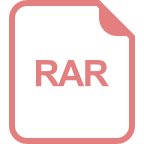
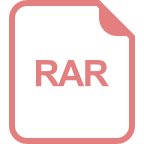
