用Verilog HDL语言实现取指电路,取指电路需完成ADD32、PC寄存器、多路选择器和左移两位模块,实现该取指电路。
时间: 2024-02-12 18:08:37 浏览: 27
很抱歉,我无法为您提供完整的Verilog HDL代码,因为这需要更多的详细信息和设计规范来实现。但我可以给您一些关于如何实现取指电路的指导。
首先,ADD32模块的作用是将PC寄存器的值加上4(因为每次取指令PC都要自增4),得到下一条指令的地址。多路选择器的作用是从指令存储器中选择需要的指令,通常是通过指令地址来确定需要的指令。左移两位模块的作用是将指令存储器中的指令地址左移两位,因为指令存储器通常是按字节寻址的,而指令地址是按字寻址的。
在Verilog HDL中,您可以使用模块化设计的原则来实现取指电路。您需要首先编写ADD32模块的代码,然后编写PC寄存器模块,接着编写多路选择器模块,最后编写左移两位模块。您可以使用模块实例化的方式将这些模块组装成一个完整的取指电路。
下面是一个简单的示例代码,帮助您理解如何实现取指电路:
```
module add32(
input [31:0] a,
output reg [31:0] result
);
always @(*) begin
result = a + 32'b100;
end
endmodule
module pc_reg(
input clk,
input rst,
input [31:0] pc_in,
output reg [31:0] pc_out
);
always @(posedge clk, posedge rst) begin
if (rst == 1'b1) begin
pc_out <= 32'b0;
end else begin
pc_out <= pc_in;
end
end
endmodule
module mux(
input [31:0] a,
input [31:0] b,
input [31:0] c,
input [31:0] d,
input [1:0] sel,
output reg [31:0] result
);
always @(*) begin
case (sel)
2'b00: result = a;
2'b01: result = b;
2'b10: result = c;
2'b11: result = d;
endcase
end
endmodule
module left_shift(
input [31:0] a,
output reg [31:0] result
);
always @(*) begin
result = a << 2;
end
endmodule
module fetch(
input clk,
input rst,
input [31:0] pc,
input [31:0] inst_mem [65535:0],
output reg [31:0] inst_out
);
reg [31:0] add32_out;
reg [31:0] pc_reg_out;
reg [31:0] mux_out;
reg [31:0] left_shift_out;
add32 add32_inst(
.a(pc),
.result(add32_out)
);
pc_reg pc_reg_inst(
.clk(clk),
.rst(rst),
.pc_in(add32_out),
.pc_out(pc_reg_out)
);
mux mux_inst(
.a(inst_mem[left_shift_out[15:2]]),
.b(inst_mem[left_shift_out[15:2]+1]),
.c(inst_mem[left_shift_out[15:2]+2]),
.d(inst_mem[left_shift_out[15:2]+3]),
.sel(left_shift_out[1:0]),
.result(mux_out)
);
left_shift left_shift_inst(
.a(pc_reg_out),
.result(left_shift_out)
);
always @(posedge clk, posedge rst) begin
if (rst == 1'b1) begin
inst_out <= 32'b0;
end else begin
inst_out <= mux_out;
end
end
endmodule
```
请注意,以上代码仅供参考,实际实现可能会有所不同,具体取决于设计规范和性能要求。
相关推荐
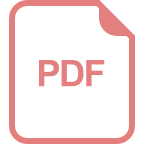














