go语言float64相加
时间: 2024-02-02 08:03:35 浏览: 23
可以直接使用"+"运算符进行相加,例如:
```go
var x float64 = 2.5
var y float64 = 3.7
sum := x + y
fmt.Println(sum) // 输出:6.2
```
请注意,float类型相加时可能会出现精度误差,建议使用math包中的函数进行精确计算。
相关问题
go语言Int转float64
可以使用 float64 函数将 int 类型转换为 float64 类型,示例代码如下:
```
var numInt int = 10
var numFloat float64 = float64(numInt)
```
在上面的代码中,将 numInt 变量从 int 类型转换为 float64 类型,并将结果存储在 numFloat 变量中。
go语言int除以100并转为float64
可以先将 int 类型除以 100 得到一个 float64 类型的结果,然后再将结果强制转换为 float64 类型。示例代码如下:
```
var numInt int = 100
var numFloat float64 = float64(numInt) / 100.0
```
在上面的代码中,将 numInt 变量除以 100 得到一个 float64 类型的结果,并将结果存储在 numFloat 变量中。需要注意的是,在除以 100 的时候,需要使用 100.0 来保证结果是 float64 类型,而不是 int 类型。
相关推荐
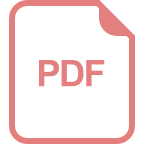
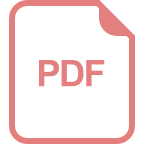
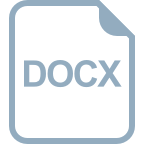












