请你扮演一个资深前端开发工程师,用vue写一个A页面跳转B页面,B页面提交多条表单信息后添加到数组中,然后返回A页面,在A页面中获取B页面提交的表单信息
时间: 2024-05-01 14:23:29 浏览: 130
好的,假设我们已经有了一个Vue的项目,我们可以通过Vue Router来实现页面的跳转。
首先,在router/index.js中定义路由:
```javascript
import Vue from 'vue'
import Router from 'vue-router'
import A from '@/components/A'
import B from '@/components/B'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'A',
component: A
},
{
path: '/b',
name: 'B',
component: B
}
]
})
```
接下来,我们在A页面中添加一个按钮,点击按钮可以跳转到B页面:
```html
<template>
<div>
<h1>A页面</h1>
<button @click="goToB">跳转到B页面</button>
</div>
</template>
<script>
export default {
methods: {
goToB () {
this.$router.push('/b')
}
}
}
</script>
```
在B页面中,我们需要添加一个表单,当用户提交表单时,将表单信息添加到一个数组中,并跳转回A页面:
```html
<template>
<div>
<h1>B页面</h1>
<form @submit.prevent="submitForm">
<label for="name">姓名</label>
<input type="text" id="name" v-model="form.name">
<br>
<label for="age">年龄</label>
<input type="number" id="age" v-model="form.age">
<br>
<button type="submit">提交</button>
</form>
</div>
</template>
<script>
export default {
data () {
return {
form: {
name: '',
age: ''
}
}
},
methods: {
submitForm () {
this.$store.commit('addFormData', this.form)
this.$router.push('/')
}
}
}
</script>
```
在这里,我们使用了Vuex来管理数据,当用户提交表单时,我们通过Vuex的commit方法来触发一个mutation,将表单信息添加到一个数组中。
在store/index.js中,我们定义一个state和一个mutation:
```javascript
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
formData: []
},
mutations: {
addFormData (state, form) {
state.formData.push(form)
}
}
})
```
最后,在A页面中,我们可以通过Vuex的state来获取B页面提交的表单信息:
```html
<template>
<div>
<h1>A页面</h1>
<button @click="goToB">跳转到B页面</button>
<div v-for="(form, index) in formData" :key="index">
<p>姓名:{{ form.name }}</p>
<p>年龄:{{ form.age }}</p>
</div>
</div>
</template>
<script>
export default {
computed: {
formData () {
return this.$store.state.formData
}
},
methods: {
goToB () {
this.$router.push('/b')
}
}
}
</script>
```
在这里,我们使用了Vuex的computed属性来获取存储在state中的表单数据。通过v-for指令,我们可以将数据展示出来。
这样,我们就完成了A页面跳转B页面,B页面提交表单信息后添加到数组中,返回A页面并获取B页面提交的表单信息的整个过程。
阅读全文
相关推荐
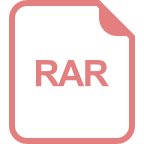
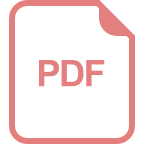
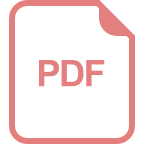
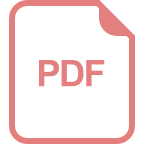
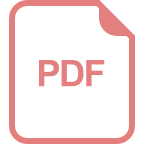
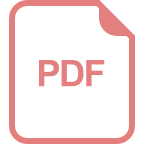
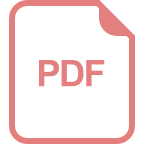
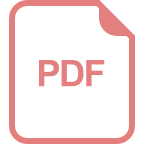
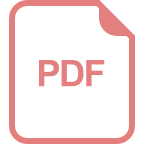
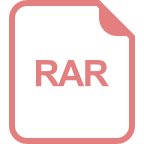
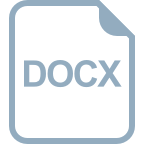