mybatis防止sql注入
时间: 2023-10-08 10:14:21 浏览: 67
MyBatis 有以下几种方法来防止 SQL 注入:
1. 使用参数化查询
MyBatis 推荐使用参数化查询来防止 SQL 注入。参数化查询使用 ? 或者 #{param} 来表示占位符,然后使用 PreparedStatement 进行预编译,将参数设置到占位符中,最后执行查询操作。这种方式可以避免用户输入的参数直接拼接到 SQL 语句中,从而防止 SQL 注入攻击。
示例:
```
<select id="getUserById" parameterType="int" resultType="User">
SELECT * FROM user WHERE id = #{id}
</select>
```
2. 使用动态 SQL
MyBatis 的动态 SQL 可以根据条件动态生成 SQL 语句,避免拼接 SQL 语句时产生 SQL 注入漏洞。MyBatis 提供了多种动态 SQL 标签,如 if、choose、when、otherwise、foreach、set、trim、where、sql 等。
示例:
```
<select id="getUserList" parameterType="Map" resultType="User">
SELECT * FROM user
<where>
<if test="name != null and name != ''">
AND name = #{name}
</if>
<if test="age != null">
AND age = #{age}
</if>
</where>
</select>
```
3. 使用 SQL 注入过滤器
MyBatis 可以通过插件来实现 SQL 注入过滤器,对于输入的参数进行过滤和转义,确保参数不会被注入到 SQL 语句中。
示例:
```
public class SqlInjectionPlugin implements Interceptor {
@Override
public Object intercept(Invocation invocation) throws Throwable {
Object[] args = invocation.getArgs();
for (int i = 0; i < args.length; i++) {
if (args[i] instanceof String) {
args[i] = sqlInjectionFilter((String) args[i]);
}
}
return invocation.proceed();
}
private String sqlInjectionFilter(String param) {
// 进行参数过滤和转义
return param;
}
@Override
public Object plugin(Object target) {
return Plugin.wrap(target, this);
}
@Override
public void setProperties(Properties properties) {
// 配置属性
}
}
```
相关推荐
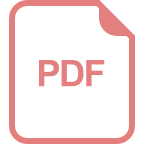
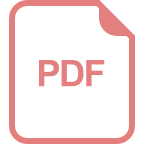
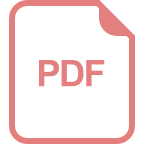














