springboot 实现文件下载
时间: 2023-10-20 18:36:38 浏览: 255
在Spring Boot中实现文件下载可以通过以下步骤进行:
1. 首先,确保你的Spring Boot项目中添加了`spring-boot-starter-web`依赖。
2. 创建一个Controller类,用于处理文件下载请求。可以使用`@RestController`注解标记该类,并使用`@GetMapping`注解配置下载接口的URL路径。
3. 在下载接口方法中,使用`@PathVariable`注解来接收文件名作为参数。
4. 使用`ClassPathResource`类加载需要下载的文件。你可以通过指定文件路径或者通过相对于classpath的路径来加载文件,取决于你的项目结构。
5. 使用`HttpServletResponse`对象设置相关的响应头信息,如Content-Type、Content-Disposition等,以确保文件以附件形式下载。
6. 使用`FileCopyUtils`类的`copy()`方法将文件内容写入到response的输出流中。
以下是一个简单的示例代码:
```java
import org.springframework.core.io.ClassPathResource;
import org.springframework.util.FileCopyUtils;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.InputStream;
@RestController
public class FileDownloadController {
@GetMapping("/download/{fileName}")
public void downloadFile(@PathVariable String fileName, HttpServletResponse response) throws IOException {
ClassPathResource resource = new ClassPathResource("path/to/files/" + fileName);
if (resource.exists()) {
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment; filename=" + fileName);
InputStream inputStream = resource.getInputStream();
FileCopyUtils.copy(inputStream, response.getOutputStream());
inputStream.close();
} else {
response.setStatus(HttpServletResponse.SC_NOT_FOUND);
}
}
}
```
请注意,上述代码中的`path/to/files/`应该根据你的实际文件路径进行替换。这个路径是相对于classpath的路径,因此可以根据你的项目结构进行调整。
这样,当访问`/download/{fileName}`时,就会触发文件下载操作,并将指定的文件作为附件下载到客户端。
阅读全文
相关推荐

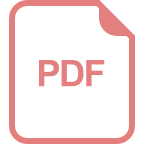
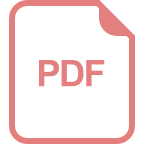
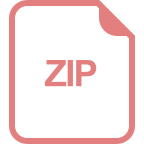
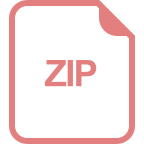

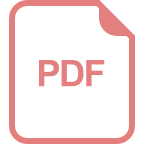
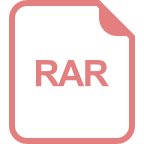
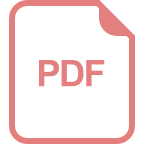
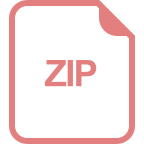
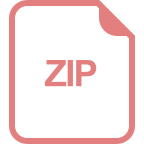
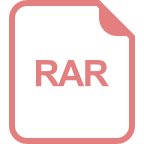
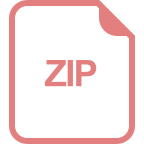