document.onmousemove = (e) => { //用鼠标的位置减去鼠标相对元素的位置,得到元素的位置 left = e.clientX - disX; top = e.clientY - disY; var docWidth = document.body.clientWidth //网页可见宽 var docHeight = document.body.clientHeight //网页可见高 if (top > docHeight - 210) { //超下边界(键盘DIV长宽分别为 295px、210px) top = docHeight - 210 } if (top < 0) { //超上边界 top = 0 } if (left > docWidth - 295) { //超右边界 left = docWidth - 295 } if (left < 0) { //超左边界 left = 0 } //绑定元素位置到positionX和positionY上面 //移动当前元素 odiv.style.left = left + 'px'; odiv.style.top = top + 'px'; };把这段代码改为不超过元素宽高
时间: 2024-02-10 22:04:41 浏览: 25
你可以尝试使用以下的代码来限制元素不超过网页的宽高范围:
```
document.onmousemove = (e) => {
let left = e.clientX - disX;
let top = e.clientY - disY;
const docWidth = document.body.clientWidth;
const docHeight = document.body.clientHeight;
const elWidth = odiv.offsetWidth; // 获取元素宽度
const elHeight = odiv.offsetHeight; // 获取元素高度
if (top > docHeight - elHeight) {
top = docHeight - elHeight;
}
if (top < 0) {
top = 0;
}
if (left > docWidth - elWidth) {
left = docWidth - elWidth;
}
if (left < 0) {
left = 0;
}
odiv.style.left = left + 'px';
odiv.style.top = top + 'px';
};
```
这样修改之后,元素就不会超出网页的宽高范围了。
相关问题
// 鼠标按下事件 imgContainer.onmousedown = function (e) { e.preventDefault(); isDragging = true; startX = e.clientX; startY = e.clientY; startScrollLeft = imgContainer.scrollLeft; startScrollTop = imgContainer.scrollTop; }; // 鼠标移动事件 imgContainer.onmousemove = function (e) { if (isDragging) { var moveX = e.clientX - startX; var moveY = e.clientY - startY; imgContainer.scrollLeft = startScrollLeft - moveX; imgContainer.scrollTop = startScrollTop - moveY; } }; // 鼠标释放事件 imgContainer.onmouseup = function (e) { isDragging = false; }; // 鼠标离开事件 imgContainer.onmouseleave = function (e) { isDragging = false; }; // 鼠标滚轮事件 img.onwheel = function (e) { e.preventDefault(); // 阻止默认滚轮行为处理页面滚动 var rect = img.getBoundingClientRect(); var mouseX = e.clientX - rect.left; // 鼠标在图片上的相对X坐标 var mouseY = e.clientY - rect.top; // 鼠标在图片上的相对Y坐标 var oldWidth = rect.width; // 原始图片宽度 var oldHeight = rect.height; // 原始图片高度 scale += e.deltaY > 0 ? -scaleFactor : scaleFactor; // 根据滚轮滚动方向确定缩放比例 scale = Math.max(scale, 0.1); // 最小缩放比例为0.1 var newWidth = oldWidth * scale; // 缩放后的宽度 var newHeight = oldHeight * scale; // 缩放后的高度 var scaleX = newWidth / oldWidth; // 宽度缩放比例 var scaleY = newHeight / oldHeight; // 高度缩放比例 var offsetX = (mouseX - rect.width / 2) * scaleX; // X轴偏移量 var offsetY = (mouseY - rect.height / 2) * scaleY; // Y轴偏移量 img.style.transform = scale(${scale}) translate(${offsetX}px, ${offsetY}px); }; // 关闭悬浮窗 popup.onclick = function () { document.body.removeChild(popup); isPopupVisible = false; // 标记悬浮窗为隐藏状态 }; imgContainer.appendChild(img); popup.appendChild(imgContainer); document.body.appendChild(popup); }这是修改后代码,任然存在图片跳动,无法正常放大问题
在代码中,图片跳动的问题可能是由于鼠标滚轮事件处理函数中的缩放和偏移计算不准确导致的。你可以尝试修改代码,将缩放和偏移计算的逻辑分开处理,以确保计算的准确性。修改后的代码如下:
```javascript
// 鼠标滚轮事件
img.onwheel = function (e) {
e.preventDefault(); // 阻止默认滚轮行为处理页面滚动
var rect = img.getBoundingClientRect();
var mouseX = e.clientX - rect.left; // 鼠标在图片上的相对X坐标
var mouseY = e.clientY - rect.top; // 鼠标在图片上的相对Y坐标
var oldWidth = rect.width; // 原始图片宽度
var oldHeight = rect.height; // 原始图片高度
scale += e.deltaY > 0 ? -scaleFactor : scaleFactor; // 根据滚轮滚动方向确定缩放比例
scale = Math.max(scale, 0.1); // 最小缩放比例为0.1
var newWidth = oldWidth * scale; // 缩放后的宽度
var newHeight = oldHeight * scale; // 缩放后的高度
var scaleX = newWidth / oldWidth; // 宽度缩放比例
var scaleY = newHeight / oldHeight; // 高度缩放比例
var offsetX = (mouseX - rect.width / 2) * scaleX; // X轴偏移量
var offsetY = (mouseY - rect.height / 2) * scaleY; // Y轴偏移量
var newOffsetX = offsetX - (newWidth - oldWidth) / 2; // 考虑缩放引起的偏移变化
var newOffsetY = offsetY - (newHeight - oldHeight) / 2; // 考虑缩放引起的偏移变化
img.style.transform = `scale(${scale}) translate(${newOffsetX}px, ${newOffsetY}px)`;
};
```
请尝试使用上述修改后的代码,看看是否能够解决图片跳动的问题。如果问题仍然存在,请提供更多相关代码或详细描述你想要实现的效果,以便我能够提供更准确的帮助。
请根据代码片段仿写实现div左下角拖拽移动用具体代码实现,import Vue from 'vue' Vue.directive('dialogZoomOut', { bind(el, binding, vnode, oldVnode) { let minWidth = 400;let minHeight = 300;let isFullScreen = false; let nowWidth = 0;let nowHight = 0;let nowMarginTop = 0;const dialogHeaderEl = el.querySelector('.el-dialog__header');const dragDom = el.querySelector('.el-dialog');dragDom.style.overflow = "auto";dialogHeaderEl.onselectstart = new Function("return false");dialogHeaderEl.style.cursor = 'move';const sty = dragDom.currentStyle || window.getComputedStyle(dragDom, null);let moveDown = (e) => {const disX = e.clientX - dialogHeaderEl.offsetLeft;const disY = e.clientY - dialogHeaderEl.offsetTop;let styL, styT;if (sty.left.includes('%')) {styL = +document.body.clientWidth * (+sty.left.replace(/%/g, '') / 100);styT = +document.body.clientHeight * (+sty.top.replace(/%/g, '') / 100);} else {styL = +sty.left.replace(/px/g, '');styT = +sty.top.replace(/px/g, '');};document.onmousemove = function (e) {const l = e.clientX - disX;const t = e.clientY - disY;dragDom.style.left = `${l + styL}px`;dragDom.style.top = `${t + styT}px`;};document.onmouseup = function (e) {document.onmousemove = null;document.onmouseup = null;};}dialogHeaderEl.onmousedown = moveDown;dialogHeaderEl.ondblclick = (e) => {if (isFullScreen == false) {nowHight = dragDom.clientHeight;nowWidth = dragDom.clientWidth;nowMarginTop = dragDom.style.marginTop;dragDom.style.left = 0;dragDom.style.top = 0;dragDom.style.height = "100VH";dragDom.style.width = "100VW";dragDom.style.marginTop = 0;isFullScreen = true;dialogHeaderEl.style.cursor = 'initial';dialogHeaderEl.onmousedown = null;} else {dragDom.style.height = "auto";dragDom.style.width = nowWidth + 'px';dragDom.style.marginTop = nowMarginTop;isFullScreen = false;dialogHeaderEl.style.cursor = 'move';dialogHeaderEl.onmousedown = moveDown;}}let resizeEl = document.createElement("div");dragDom.appendChild(resizeEl);resizeEl.style.cursor = 'se-resize';resizeEl.style.position = 'absolute';resizeEl.style.height = '10px';resizeEl.style.width = '10px';resizeEl.style.right = '0px';resizeEl.style.bottom = '0px';resizeEl.style.zIndex = '99';resizeEl.onmousedown = (e) => {let clientX = e.clientX;let disX = e.clientX - resizeEl.offsetLeft;let disY = e.clientY - resizeEl.offsetTop;document.onmousemove = function (e) {e.preventDefault(); let x = e.clientX - disX + (e.clientX - clientX);let y = e.clientY - disY;dragDom.style.width = x > minWidth ? `${x}px` : minWidth + 'px';dragDom.style.height = y > minHeight ? `${y}px` : minHeight + 'px';};document.onmouseup = function (e) {document.onmousemove = null;document.onmouseup = null;};}}})
以下是仿写的代码实现:
```html
<template>
<div class="dialog-wrapper" v-dialog-zoom-out>
<div class="el-dialog">
<div class="el-dialog__header">Dialog Title</div>
<div class="el-dialog__body">Dialog Content</div>
<div class="el-dialog__footer">
<button @click="$emit('close')">Close</button>
</div>
<div class="el-dialog__resize"></div>
</div>
</div>
</template>
<script>
export default {
name: "Dialog",
};
</script>
<style>
.dialog-wrapper {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
.el-dialog {
position: absolute;
top: 0;
left: 0;
overflow: auto;
max-height: 80vh;
background-color: #fff;
border-radius: 4px;
box-shadow: 0 1px 3px rgba(0, 0, 0, 0.3);
}
.el-dialog__header {
padding: 16px;
background-color: #f5f7fa;
border-top-left-radius: 4px;
border-top-right-radius: 4px;
cursor: move;
}
.el-dialog__body {
padding: 16px;
}
.el-dialog__footer {
display: flex;
justify-content: flex-end;
padding: 16px;
background-color: #f5f7fa;
border-bottom-left-radius: 4px;
border-bottom-right-radius: 4px;
}
.el-dialog__footer button {
padding: 8px 16px;
background-color: #fff;
border: 1px solid #c1c1c1;
border-radius: 4px;
cursor: pointer;
}
.el-dialog__resize {
position: absolute;
bottom: 0;
right: 0;
width: 10px;
height: 10px;
cursor: se-resize;
background-color: #c1c1c1;
}
</style>
```
```javascript
import Vue from "vue";
Vue.directive("dialogZoomOut", {
bind(el, binding, vnode, oldVnode) {
let minWidth = 400;
let minHeight = 300;
let isFullScreen = false;
let nowWidth = 0;
let nowHight = 0;
let nowMarginTop = 0;
const dialogHeaderEl = el.querySelector(".el-dialog__header");
const dragDom = el.querySelector(".el-dialog");
dragDom.style.overflow = "auto";
dialogHeaderEl.onselectstart = new Function("return false");
dialogHeaderEl.style.cursor = "move";
const sty = dragDom.currentStyle || window.getComputedStyle(dragDom, null);
let moveDown = (e) => {
const disX = e.clientX - dialogHeaderEl.offsetLeft;
const disY = e.clientY - dialogHeaderEl.offsetTop;
let styL, styT;
if (sty.left.includes("%")) {
styL = +document.body.clientWidth * (+sty.left.replace(/%/g, "") / 100);
styT = +document.body.clientHeight * (+sty.top.replace(/%/g, "") / 100);
} else {
styL = +sty.left.replace(/px/g, "");
styT = +sty.top.replace(/px/g, "");
}
document.onmousemove = function (e) {
const l = e.clientX - disX;
const t = e.clientY - disY;
dragDom.style.left = `${l + styL}px`;
dragDom.style.top = `${t + styT}px`;
};
document.onmouseup = function (e) {
document.onmousemove = null;
document.onmouseup = null;
};
};
dialogHeaderEl.onmousedown = moveDown;
dialogHeaderEl.ondblclick = (e) => {
if (isFullScreen == false) {
nowHight = dragDom.clientHeight;
nowWidth = dragDom.clientWidth;
nowMarginTop = dragDom.style.marginTop;
dragDom.style.left = 0;
dragDom.style.top = 0;
dragDom.style.height = "100VH";
dragDom.style.width = "100VW";
dragDom.style.marginTop = 0;
isFullScreen = true;
dialogHeaderEl.style.cursor = "initial";
dialogHeaderEl.onmousedown = null;
} else {
dragDom.style.height = "auto";
dragDom.style.width = nowWidth + "px";
dragDom.style.marginTop = nowMarginTop;
isFullScreen = false;
dialogHeaderEl.style.cursor = "move";
dialogHeaderEl.onmousedown = moveDown;
}
};
let resizeEl = document.createElement("div");
dragDom.appendChild(resizeEl);
resizeEl.style.cursor = "se-resize";
resizeEl.style.position = "absolute";
resizeEl.style.height = "10px";
resizeEl.style.width = "10px";
resizeEl.style.right = "0px";
resizeEl.style.bottom = "0px";
resizeEl.style.zIndex = "99";
resizeEl.onmousedown = (e) => {
let clientX = e.clientX;
let disX = e.clientX - resizeEl.offsetLeft;
let disY = e.clientY - resizeEl.offsetTop;
document.onmousemove = function (e) {
e.preventDefault();
let x = e.clientX - disX + (e.clientX - clientX);
let y = e.clientY - disY;
dragDom.style.width = x > minWidth ? `${x}px` : minWidth + "px";
dragDom.style.height = y > minHeight ? `${y}px` : minHeight + "px";
};
document.onmouseup = function (e) {
document.onmousemove = null;
document.onmouseup = null;
};
};
},
});
```
上述代码实现了一个类似于Element UI中的Dialog组件的拖拽和缩放功能。在使用时,只需要将 `v-dialog-zoom-out` 指令绑定到Dialog组件的容器元素上,即可实现拖拽和缩放功能。
相关推荐
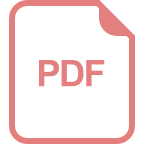
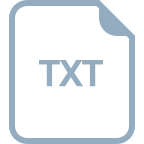













