jsonobject根据属性拆分
时间: 2023-08-19 18:03:51 浏览: 98
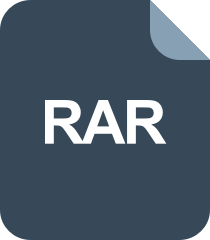
自定义JSONObject属性过滤注解
如果你想根据某个属性将一个 JSON 对象拆分成多个 JSON 对象,可以按照以下步骤操作:
1. 将 JSON 字符串转换成 JSON 对象。
2. 遍历 JSON 对象的每个属性,如果某个属性的值是数组,就对数组进行遍历。
3. 在遍历数组的过程中,根据数组中某个属性的值来创建一个新的 JSON 对象,并将原本的属性拷贝到新的 JSON 对象中。
4. 将新的 JSON 对象添加到一个新的 JSON 数组中。
5. 最后将新的 JSON 数组转换成 JSON 字符串。
以下是一个示例代码,假设我们要根据属性 "type" 拆分 JSON 对象:
```java
import org.json.*;
public class JsonObjectSplitter {
public static void main(String[] args) {
String jsonString = "{\"items\": [{\"name\": \"item1\", \"type\": \"type1\", \"value\": 1}, {\"name\": \"item2\", \"type\": \"type2\", \"value\": 2}, {\"name\": \"item3\", \"type\": \"type1\", \"value\": 3}]}";
JSONObject jsonObject = new JSONObject(jsonString);
JSONArray jsonArray = jsonObject.getJSONArray("items");
JSONObject newJsonObject = new JSONObject();
JSONArray newJsonArray = new JSONArray();
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject itemJsonObject = jsonArray.getJSONObject(i);
String type = itemJsonObject.getString("type");
if (!newJsonObject.has(type)) {
newJsonObject.put(type, new JSONArray());
}
JSONObject newItemJsonObject = new JSONObject(itemJsonObject, new String[]{"name", "value"});
newJsonObject.getJSONArray(type).put(newItemJsonObject);
}
for (String key : newJsonObject.keySet()) {
JSONObject typeJsonObject = new JSONObject();
typeJsonObject.put("type", key);
typeJsonObject.put("items", newJsonObject.getJSONArray(key));
newJsonArray.put(typeJsonObject);
}
JSONObject resultJsonObject = new JSONObject();
resultJsonObject.put("items", newJsonArray);
System.out.println(resultJsonObject.toString());
}
}
```
输出结果如下:
```json
{
"items": [
{
"type": "type1",
"items": [
{
"name": "item1",
"value": 1
},
{
"name": "item3",
"value": 3
}
]
},
{
"type": "type2",
"items": [
{
"name": "item2",
"value": 2
}
]
}
]
}
```
阅读全文
相关推荐
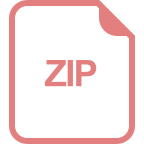
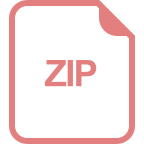













