如何用python将图片中的文字提取出来(调用百度ipa)
时间: 2024-10-10 15:09:14 浏览: 19
在Python中,你可以使用百度提供的OCR(Optical Character Recognition,光学字符识别)服务,如`pytesseract`库配合百度云OCR API来提取图片中的文字。以下是基本步骤:
1. **安装所需库**:
首先,确保已经安装了`PIL`(Python Imaging Library)用于处理图像,` pytesseract `用于调用Tesseract OCR引擎,以及`requests`库用于发送HTTP请求。
```shell
pip install Pillow pytesseract requests
```
2. **注册百度API**:
访问百度云官网,注册并创建一个项目,获取到AppID和Secret Key(注意保密),这是调用API的关键凭证。
3. **使用百度OCR API**:
使用`requests`库发送POST请求给百度的OCR API,上传图片文件,并设置识别语言。
```python
import requests
from PIL import Image
def extract_text_from_image(image_path, app_id, secret_key):
# 图片转二进制数据
with open(image_path, 'rb') as f:
img_data = f.read()
headers = {
"api-key": app_id,
"app-id": app_id,
"timestamp": str(int(time.time())),
"nonce-str": generate_nonce(),
"sign": create_sign(app_id, secret_key, headers),
}
url = "https://aip.baidubce.com/rest/2.0/vision/ocr/v1/general"
response = requests.post(url, headers=headers, data=img_data)
result = response.json()
if response.status_code == 200:
for item in result["words_result"]:
return item["words"]
else:
print(f"Error: {response.content}")
def generate_nonce():
import random
return str(random.randint(32768, 65535))
def create_sign(app_id, secret_key, headers):
string_to_sign = "\n".join([f"{k}:{v}" for k, v in sorted(headers.items())])
string_to_sign += "\n" + app_id + "\n" + secret_key
hash_object = hashlib.sha256(string_to_sign.encode("utf-8"))
sign = hash_object.hexdigest()
return sign
image_path = "path/to/your/image.jpg" # 替换为你的图片路径
text = extract_text_from_image(image_path, "你的AppID", "你的SecretKey")
print(text)
```
4. **注意事项**:
- Tesseract需要预先安装,对于Windows用户可以下载预训练的语言包,比如`tesseract-ocr`。对于Linux或Mac用户,可能需要安装额外依赖。
- 提供的照片应清晰,且包含的文字尽量大且容易辨认。
阅读全文
相关推荐
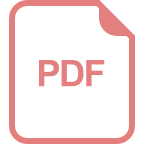
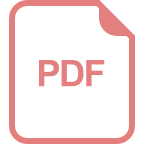
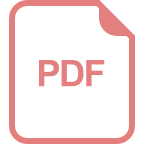
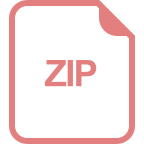
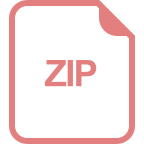
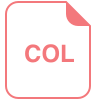
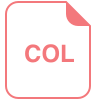
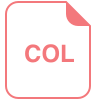
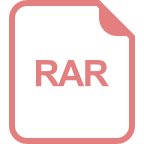
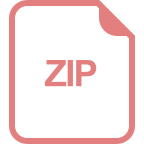
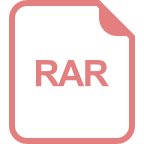