httpclient发送post请求 参数 integer
时间: 2023-06-19 14:03:44 浏览: 280
发送 POST 请求时,可以通过设置请求体来传递参数。如果参数是整数类型,可以将其转换为字符串类型并设置到请求体中。以下是使用 Apache HttpClient 发送带有整数参数的 POST 请求的示例代码:
```
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import java.net.URI;
import java.util.ArrayList;
import java.util.List;
public class HttpClientPostExample {
public static void main(String[] args) throws Exception {
CloseableHttpClient httpClient = HttpClients.createDefault();
// 设置请求参数
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("param1", "123"));
// 构建请求体
HttpEntity entity = new StringEntity("param1=123", ContentType.APPLICATION_FORM_URLENCODED);
// 构建请求 URI
URI uri = new URIBuilder()
.setScheme("http")
.setHost("example.com")
.setPath("/api")
.build();
// 构建 POST 请求
HttpPost httpPost = new HttpPost(uri);
httpPost.setEntity(entity);
// 执行请求
CloseableHttpResponse response = httpClient.execute(httpPost);
try {
HttpEntity responseEntity = response.getEntity();
String result = EntityUtils.toString(responseEntity);
System.out.println(result);
} finally {
response.close();
}
}
}
```
在上面的示例代码中,我们将整数参数设置为字符串类型,并将其放入请求体中。在构建请求体时,我们使用了 ContentType.APPLICATION_FORM_URLENCODED 类型,该类型表示请求体中包含 URL 编码的表单参数。在执行请求后,我们将响应结果转换为字符串并打印出来。
阅读全文
相关推荐
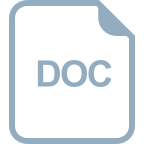
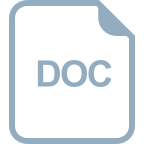
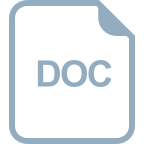
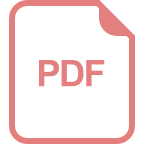
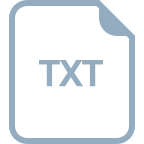
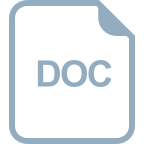
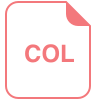
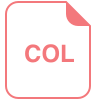
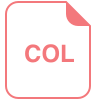
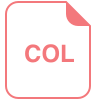
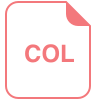







