python爬虫 后数据保存
时间: 2023-11-08 08:23:52 浏览: 39
Python爬虫后,可以将数据保存到本地文件或数据库中。常见的数据保存方式包括:
1. 文本文件:使用Python内置的open函数打开一个文件,将爬取到的数据写入到文件中。
2. CSV文件:使用Python内置的csv模块,将数据写入到CSV文件中。
3. JSON文件:使用Python内置的json模块,将数据写入到JSON文件中。
4. 数据库:使用Python中的数据库连接库(如pymysql、sqlite3等),将数据保存到数据库中。
根据具体需求和数据类型,选择合适的保存方式。
相关问题
python爬虫数据保存
你可以使用Python的爬虫库(例如requests或Scrapy)来获取网页数据,然后将数据保存到文件或数据库中。以下是一些常见的数据保存方法:
1. 保存为文本文件:使用Python的内置open函数创建一个文件对象,并将爬取到的数据写入文件中。例如:
```
import requests
url = "https://example.com"
response = requests.get(url)
data = response.text
with open("data.txt", "w", encoding="utf-8") as f:
f.write(data)
```
2. 保存为CSV文件:如果你想以表格形式保存数据,可以使用Python的csv模块。首先,将爬取到的数据组织成列表或字典格式,然后使用csv.writer将数据写入CSV文件。例如:
```
import csv
import requests
url = "https://example.com"
response = requests.get(url)
data = response.json() # 假设返回的是JSON格式数据
headers = ["name", "age", "email"]
rows = [[item["name"], item["age"], item["email"]] for item in data]
with open("data.csv", "w", newline="", encoding="utf-8") as f:
writer = csv.writer(f)
writer.writerow(headers)
writer.writerows(rows)
```
3. 保存到数据库:如果你需要在后续的操作中使用数据,可以考虑将数据保存到数据库中(如MySQL、MongoDB等)。首先,你需要安装相应的数据库驱动程序,并连接到数据库。然后,将爬取到的数据转换成数据库可以接受的格式,插入到数据库中。例如:
```
import pymysql
import requests
url = "https://example.com"
response = requests.get(url)
data = response.json() # 假设返回的是JSON格式数据
# 连接到MySQL数据库
conn = pymysql.connect(host="localhost", user="username", password="password", database="mydatabase")
cursor = conn.cursor()
# 创建表格(如果没有)
create_table_query = "CREATE TABLE IF NOT EXISTS mytable (name VARCHAR(255), age INT, email VARCHAR(255))"
cursor.execute(create_table_query)
# 插入数据
for item in data:
insert_query = "INSERT INTO mytable (name, age, email) VALUES (%s, %s, %s)"
values = (item["name"], item["age"], item["email"])
cursor.execute(insert_query, values)
# 提交事务并关闭连接
conn.commit()
cursor.close()
conn.close()
```
这些只是一些常用的方法,
python爬虫数据保存方式
Python爬虫可以将爬取到的数据保存到不同的数据存储介质中,如文本文件、CSV文件、JSON文件、数据库等。以下是几种常用的数据保存方式:
1. 文本文件:使用Python内置的open()函数,以文本方式写入爬取到的数据到文件中。
2. CSV文件:使用Python内置的csv模块,以逗号分隔的形式将数据写入文件中。
3. JSON文件:使用Python内置的json模块,将数据以JSON格式写入文件中。
4. 数据库:使用Python内置的sqlite3模块或第三方库如MySQLdb、pymongo等,将数据保存到数据库中。
在选择数据保存方式时,需要考虑数据的结构和使用场景,选择最适合的存储介质。
相关推荐
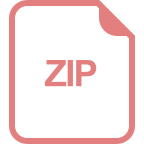
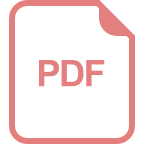
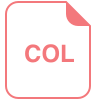
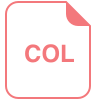
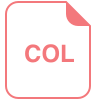
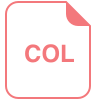
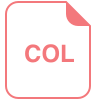







