如何使用python代码构建一个基于集成深度学习的分类框架,使用浅层神经网络代替GBDT中的决策树?
时间: 2024-05-30 09:15:29 浏览: 95
您好,关于您的问题,我可以给出以下思路:
1.首先,您需要准备训练数据集和测试数据集,并进行数据预处理和特征工程。
2.然后,您可以通过Python的各种深度学习框架(如TensorFlow、PyTorch等)来构建浅层神经网络,代替GBDT中的决策树。
3.在构建浅层神经网络时,您可以选择多种不同的网络结构,如全连接网络、卷积神经网络、循环神经网络等。
4.接下来,您可以使用交叉验证等技术来训练和调优您的神经网络,使其能够更好地适应您的数据集和分类任务。
5.最后,使用测试集来验证您的分类框架的性能和准确度。
希望这些信息能够帮助您解决问题。若还有其他问题,欢迎继续咨询。
相关问题
Python代码实现使用梯度提升树GBDT实现Boston房价预测
以下是使用Python实现使用梯度提升树GBDT实现Boston房价预测的代码:
```python
# 导入必要的库
import pandas as pd
from sklearn.datasets import load_boston
from sklearn.model_selection import train_test_split
from sklearn.ensemble import GradientBoostingRegressor
from sklearn.metrics import mean_squared_error
# 导入数据集
boston = load_boston()
# 将数据集转换为DataFrame格式
df = pd.DataFrame(boston.data, columns=boston.feature_names)
# 将目标值MEDV添加到DataFrame中
df['MEDV'] = boston.target
# 将数据集分为训练集和测试集
x_train, x_test, y_train, y_test = train_test_split(df[boston.feature_names], df['MEDV'], test_size=0.2, random_state=42)
# 构建GBDT模型
gbdt = GradientBoostingRegressor(n_estimators=100, learning_rate=0.1, max_depth=3, random_state=42)
# 模型训练
gbdt.fit(x_train, y_train)
# 模型评估
y_pred = gbdt.predict(x_test)
mse = mean_squared_error(y_test, y_pred)
print('MSE:', mse)
# 预测房价
new_x = [[0.1, 18.0, 2.0, 0, 0.5, 6.0, 70.0, 3.0, 1.0, 305.0, 19.0, 390.0, 7.0]]
new_y = gbdt.predict(new_x)
print('Predicted MEDV:', new_y)
```
以上代码中,使用load_boston()函数导入Boston房价数据集,然后将数据集转换为DataFrame格式,并将目标值MEDV添加到DataFrame中。使用train_test_split()函数将数据集分为训练集和测试集。使用GradientBoostingRegressor类构建GBDT模型,并使用fit()函数进行模型训练。使用mean_squared_error()函数对训练好的模型进行评估。最后,使用predict()函数对新的房屋特征进行预测,得到预测的房价。
Python代码使用梯度提升树GBDT实现Boston房价预测
以下是使用梯度提升树(GBDT)实现Boston房价预测的Python代码示例:
```python
# 导入所需库
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.ensemble import GradientBoostingRegressor
from sklearn.metrics import mean_squared_error
# 导入数据集
boston = datasets.load_boston()
# 定义特征和标签
X = boston.data
y = boston.target
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义模型
model = GradientBoostingRegressor(loss='ls', learning_rate=0.1, n_estimators=100, max_depth=3, random_state=42)
# 拟合模型
model.fit(X_train, y_train)
# 预测测试集
y_pred = model.predict(X_test)
# 计算均方误差(MSE)
mse = mean_squared_error(y_test, y_pred)
print("MSE: ", mse)
```
上述代码中,我们首先导入了所需的库,然后加载了波士顿房价数据集。接着我们将数据集中的特征和标签分别赋值给变量X和y,并使用train_test_split函数将数据集划分为训练集和测试集。然后我们定义了一个梯度提升树回归器,并使用fit方法拟合了训练集的数据。最后,我们使用predict方法预测了测试集的数据,并计算了均方误差(MSE),以评估模型的性能。
阅读全文
相关推荐
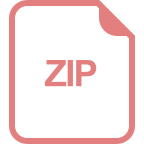
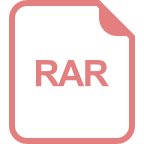
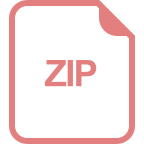













